If you have a use case where you want to prefix files within a directory with number sequences such as 001, 002, 003 .... 999. Well, for such a case you can simply write a bash script that can do this job quickly.
Below is a very simple script in bash that will do this job for you, just keep the file in the folder where you want to run it. For safety, it will ask you to enter the list of file extensions that you want to rename with a number prefix, you can add any number of file extensions separated by space. example "csv xml xls xlsx json txt"
#!/bin/bash
# Script to add a numeric prefix to files within a directory
#
# Author: Code2care.org
# Date Created: 4/5/2023
#
# Note: User needs to provide the file extensions eg. txt, CSV, xlsx
# to consider only those types.
#
# DISCLAIMER: Use this script at your own risk.
# We are not responsible for any damage it may cause.
#
echo "Enter the file extensions you want to rename (separated by spaces):"
read -ra extensions
file_count=1
for ext in "${extensions[@]}"; do
for file in *."$ext"; do
extension="${file##*.}"
new_name="$(printf "%03d" $file_count)-$file"
mv "$file" "$new_name"
((file_count++))
done
done
Example:
# ./prefix-file-script.sh
Enter the file extensions you want to rename (separated by spaces):
csv txt
# ls -l
total 8
-rw-r--r-- 1 c2ctech staff 0 May 4 23:09 001-file-c.csv
-rw-r--r-- 1 c2ctech staff 0 May 4 23:09 002-file-d.csv
-rw-r--r-- 1 c2ctech staff 0 May 4 23:09 003-file-a.txt
-rw-r--r-- 1 c2ctech staff 0 May 4 23:09 004-file-b.txt
-rwxr-xr-x@ 1 c2ctech staff 669 May 4 23:02 prefix-file-script.sh
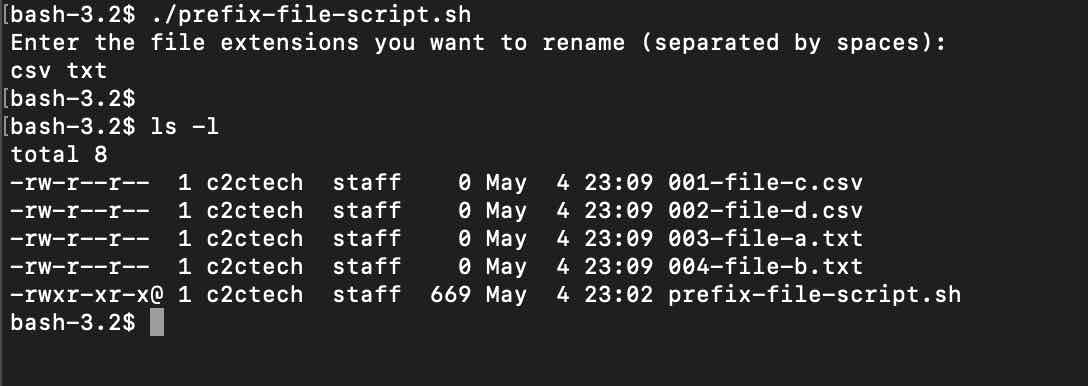
You may customize the script if you have files over 999 and if you want to have a custom string prefixed as well or use the underscore operator instead of a hyphen.
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- How to use Autocomplete and Autosuggestion in Shell Commands
- Bash How to Save Output of a Command to a Variable
- How to know the current shell you are logged in?
- How to Echo Bash Command to a File
- Bash Command to Get Absolute Path for a File
- How to Split a String based on Delimiter in Bash Scripting
- Bash: Command Line Arguments to Bash Script Examples
- Bash Command to Download a File From URL
- How to check if a Command Exists using Bash Script
- Ways to Increment a counter variable in Bash Script
- Know Bash shell version command
- Bash command to Read, Output and Manipulate JSON File
- Bash Command to Base64 Decode a String
- Bash Command to Check Python Version
- Bash: Command to Find the Length of a String
- What is $$ in Bash Shell Script- Special Variable
- Bash - How to check if a Command Failed?
- List all Username and User ID using Bash Command
- Command to Sort File In Reverse Order [Unix/Linux/macOS]
- bash: netstat: command not found
- Bash Command To Go Back To Previous Directory
- [Fix] bash: script.sh: /bin/bash^M: bad interpreter: No such file or directory
- How to check your IP using bash for Windows?
- Bash Command To Check If File Exists
- Convert String from uppercase to lowercase in Bash
- How to know file encoding in Microsoft Windows Notepad? - Microsoft
- Submit html form on dropdown menu value selection or change using javascript - JavaScript
- [Android] This view is not constrained vertically: at runtime it will jump to the top unless you add a vertical constraint - Android
- How to adjust MacBook Desktop icons size - MacOS
- How to check if a variable is set in Bash Script or Not - Bash
- How to enable Do Not Disturb (DND) mode in Microsoft Teams - Teams
- Install Bash Completion on macOS - Bash
- Program 10: Modulo of Two Numbers - 1000+ Python Programs - Python-Programs