In Bash scripting, we can split a string into substrings based on a delimiter using the built-in IFS - Internal Field Separator variable and the read command.
Let's take a look at an example.
Example 1:
#!/bin/bash
data_string="1,2,3,4,5,6,7,8,9,10"
delimiter=","
IFS="$delimiter"
read -ra str_array <<< "$data_string"
for element in "${str_array[@]}"; do
echo "$element"
done
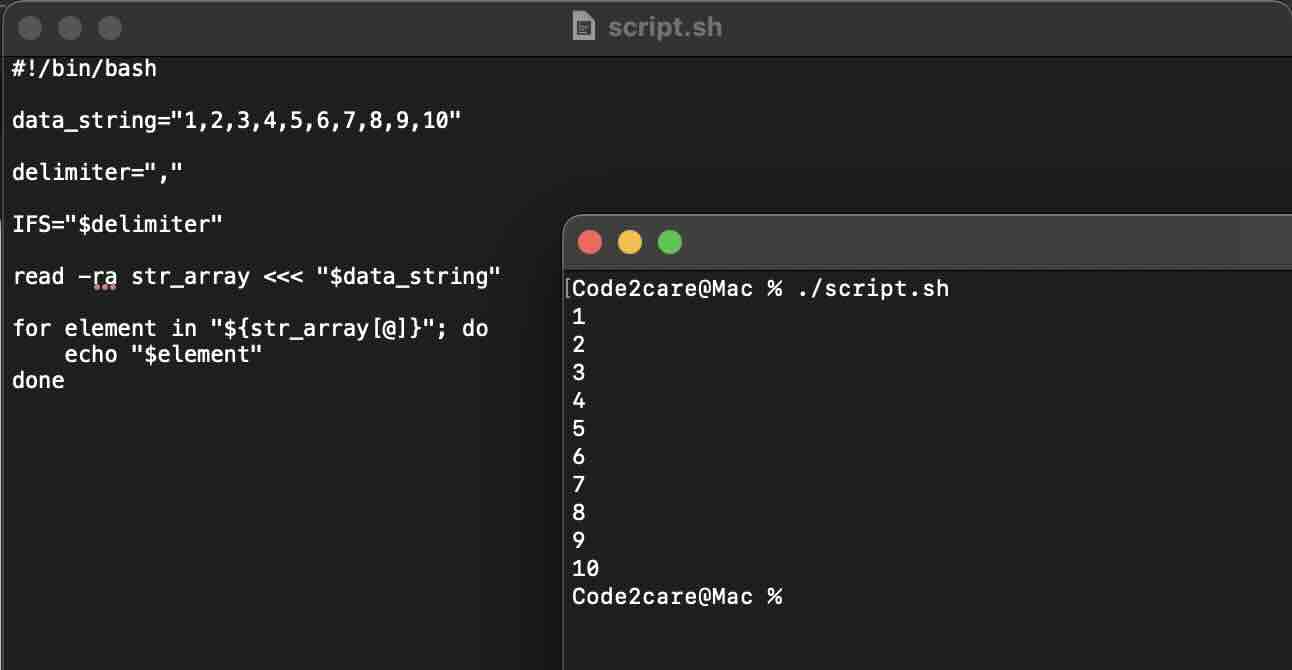
Example 2: Delimiter as Pipe
#!/bin/bash
data_string="USA|UK|China|Japan|India|France"
IFS="|"
read -ra str_array <<< "$data_string"
for element in "${str_array[@]}"; do
echo "$element"
done
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Bash,
- How to use Autocomplete and Autosuggestion in Shell Commands
- Bash How to Save Output of a Command to a Variable
- How to know the current shell you are logged in?
- How to Echo Bash Command to a File
- Bash Command to Get Absolute Path for a File
- How to Split a String based on Delimiter in Bash Scripting
- Bash: Command Line Arguments to Bash Script Examples
- Bash Command to Download a File From URL
- How to check if a Command Exists using Bash Script
- Ways to Increment a counter variable in Bash Script
- Know Bash shell version command
- Bash command to Read, Output and Manipulate JSON File
- Bash Command to Base64 Decode a String
- Bash Command to Check Python Version
- Bash: Command to Find the Length of a String
- What is $$ in Bash Shell Script- Special Variable
- Bash - How to check if a Command Failed?
- List all Username and User ID using Bash Command
- Command to Sort File In Reverse Order [Unix/Linux/macOS]
- bash: netstat: command not found
- Bash Command To Go Back To Previous Directory
- [Fix] bash: script.sh: /bin/bash^M: bad interpreter: No such file or directory
- How to check your IP using bash for Windows?
- Bash Command To Check If File Exists
- Convert String from uppercase to lowercase in Bash
More Posts:
- How to Generate SHA-512 digest in Notepad++ - NotepadPlusPlus
- Java SE JDBC with Prepared Statement Parameterized Select Example - Java
- Convert LocalDateTime to java.util.Calendar Object in Java - Java
- How to Save and Exit a File in Nano [macOS/Linux/Ubuntu] Terminal - Linux
- Add Line Break (New Line) in Jupyter Notebook Markup Cell - Python
- Write to a File using Java Stream API - Java
- Split a String into Sub-string and Parse in Python - Python
- [fix] fatal: this operation must be run in a work tree in git - Git