Let's see a few examples of how to compare Strings in a Bash Script,
Example 1: using [ ]
#!/bin/bash
name1="Mike"
name2="Mikey"
if [ "$name1" = "$name2" ]; then
echo "Both names are the same."
else
echo "Both names are not the same."
fi
Example 2: using (( ))
#!/bin/bash
name1="Mike"
name2="Mikey"
if (( "$name1" == "$name2" )); then
echo "Both names are the same."
else
echo "Both names are not the same."
fi
Example 2: using [[ ]]
#!/bin/bash
name1="Mike"
name2="Mikey"
if [[ "$name1" == "$name2" ]]; then
echo "Both names are the same."
else
echo "Both names are not the same."
fi
Comare String Methods | Explanation |
---|---|
[ "$string1" = "$string2" ] |
To check if two strings are equal. |
[ "$string1" != "$string2" ] |
To check if two strings are not equal. |
(( "$string1" == "$string2" )) |
To perform a numeric comparison for equality (0) or inequality (1). |
[[ "$string1" == "$string2" ]] |
To perform pattern matching and string comparison. |
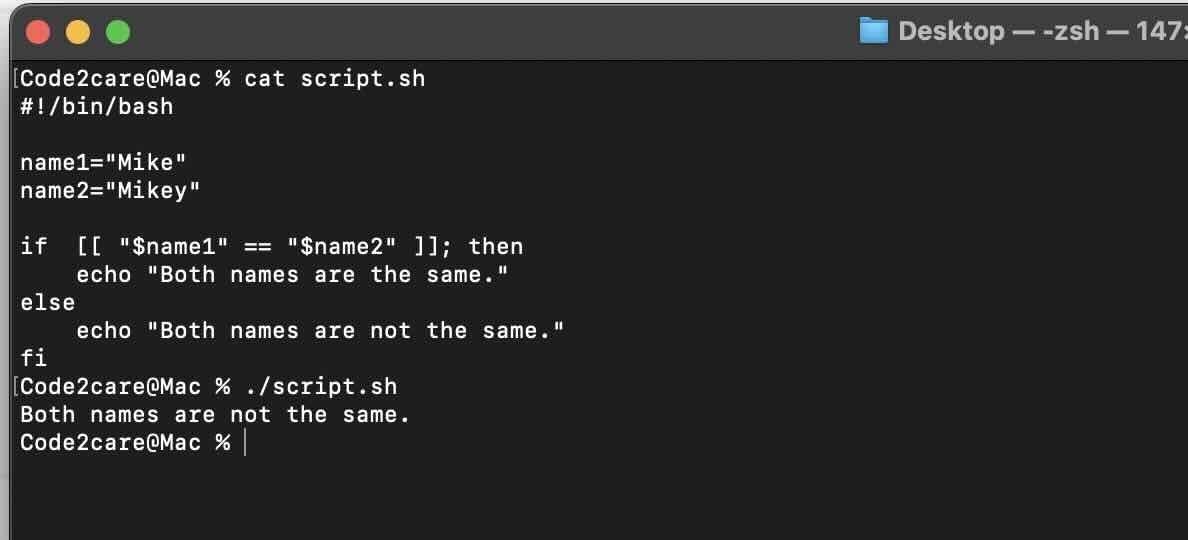
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Bash,
- How to use Autocomplete and Autosuggestion in Shell Commands
- Bash How to Save Output of a Command to a Variable
- How to know the current shell you are logged in?
- How to Echo Bash Command to a File
- Bash Command to Get Absolute Path for a File
- How to Split a String based on Delimiter in Bash Scripting
- Bash: Command Line Arguments to Bash Script Examples
- Bash Command to Download a File From URL
- How to check if a Command Exists using Bash Script
- Ways to Increment a counter variable in Bash Script
- Know Bash shell version command
- Bash command to Read, Output and Manipulate JSON File
- Bash Command to Base64 Decode a String
- Bash Command to Check Python Version
- Bash: Command to Find the Length of a String
- What is $$ in Bash Shell Script- Special Variable
- Bash - How to check if a Command Failed?
- List all Username and User ID using Bash Command
- Command to Sort File In Reverse Order [Unix/Linux/macOS]
- bash: netstat: command not found
- Bash Command To Go Back To Previous Directory
- [Fix] bash: script.sh: /bin/bash^M: bad interpreter: No such file or directory
- How to check your IP using bash for Windows?
- Bash Command To Check If File Exists
- Convert String from uppercase to lowercase in Bash
More Posts:
- [Android Studio] failed to find Build Tools revision 23.0.0 rc1 - Android-Studio
- Facebook Down Will Be Back Soon - Facebook
- How to Change the Default Search Engine on Safari - macOS Ventura/Sonoma - MacOS
- Unable to find image docker latest locally - Docker
- Open Docker Desktop from macOS Terminal - Docker
- Fix: Make makefile:31 Error 127 (GCC) - Ubuntu
- [Android Studio] Button on click example - Android-Studio
- Remove ActionBar from Activity that extends appcompat-v7 - Android