EOFError (EOF = End of File) exception is a concrete exception in Python that is raised when the input() function encounters an end-of-file condition (EOF) without reading any data.
Let us take a look at an example.
Example:user_input = input("Enter your Name: ")
print(user_input)
python3 example.py
Enter your Name: Traceback (most recent call last):
File "/Users/c2ctechtv/Desktop/example.py", line 1, in <module>
user_input = input("Enter your Name: ")
^^^^^^^^^^^^^^^^^^^^^^^^^^
EOFError
% python3 example.py
Enter your Name: Traceback (most recent call last):
File "/Users/c2ctechtv/Desktop/example.py", line 2, in <module>
user_input = input("Enter your Name: ")
^^^^^^^^^^^^^^^^^^^^^^^^^^
EOFError
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/c2ctechtv/Desktop/example.py", line 5, in <module>
print("Please provide a valid input: "+ e)
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~^~~
TypeError: can only concatenate str (not "EOFError") to str
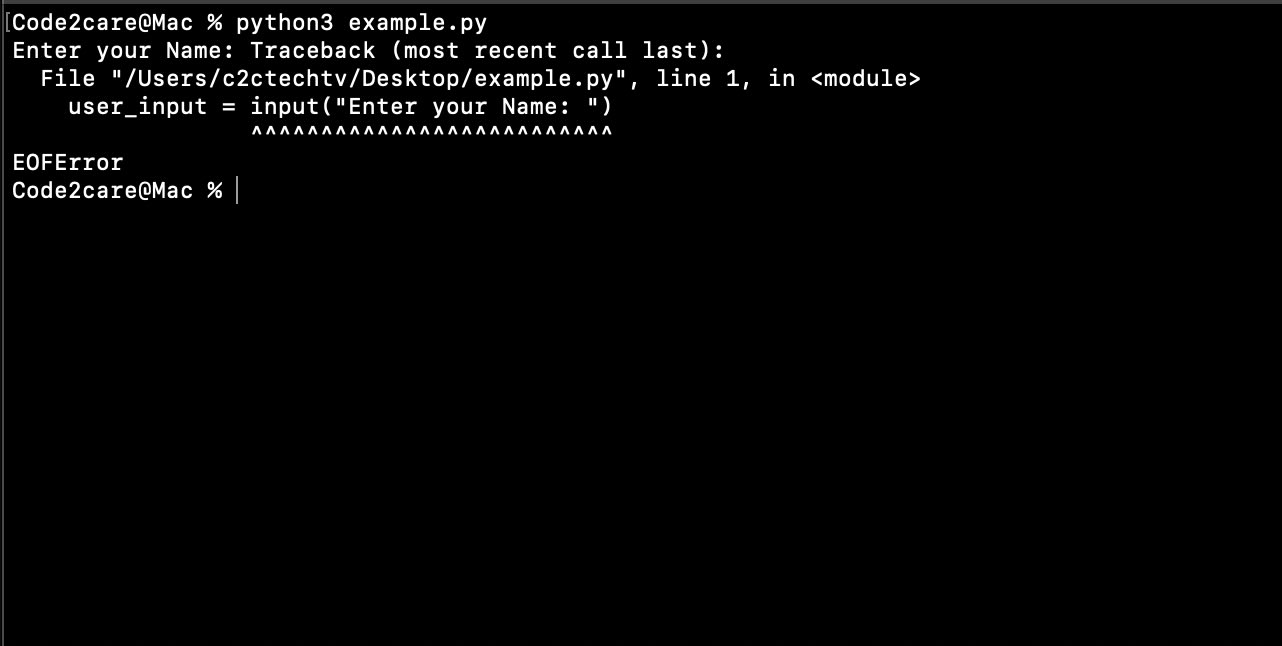
Fix EOFError:
We can handle the EOFError by surrounding the code with try/except block.
try:
user_input = input("Enter your Name: ")
print(user_input)
except EOFError as e:
print("An EOFError occurred:", e)
print("Please provide a valid name.")
except Exception as e:
print("An error occurred:", e)
python3 example.py
Enter your Name: An EOFError occurred: EOF when reading a line
Please provide a valid name.
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- How to connect to Microsoft Exchange Online using PowerShell - Powershell
- AppleScript Example with TextEdit - MacOS
- How to know if you are on iOS 17 on your iPhone - iOS
- Create SharePoint Site Collection with new Content database in existing web application - SharePoint
- Check Android Studio App is M1/M2 Chip based post installation - Android-Studio
- Disable Apps from Opening on Mac Startup (Login items) - MacOS
- How to Open Notepad as Administrator on Windows 11 - Windows
- Fix: React Native Error : UncheckedIOException: Could not move temporary workspace - Gradle