Say you have created a Python file calculator.py and you want to access this file from another Python file then follow the below steps.
Step 1: Put the file inside a folder
Create a folder say calculator and add the calculator.py file in it.
def add(a, b):
return a + b
def subtract(a, b):
return a - b
Step 2: Create __init__.py
Next, create an empty __init__.py file within the same folder.
Step 3: Create another py file.
Now let's see various ways to access the calculator.py file from main.py
Example 1: Import the entire moduleimport calculator.calculator
result = calculator.calculator.add(5, 3)
print(result)
result = calculator.calculator.subtract(8, 4)
print(result)
Example 2: Import the entire module as alias
import calculator.calculator as calc
result = calc.add(5, 3)
print(result)
result = calc.subtract(8, 4)
print(result)
Example 3: Import specific functions from the module
from calculator.calculator import add, subtract
result = add(5, 3)
print(result)
result = subtract(8, 4)
print(result)
Example 4: Import all functions from the module
from calculator.calculator import *
result = add(5, 3)
print(result)
result = subtract(8, 4)
print(result)
Files Structure
calculator/
__init__.py
calculator.py
main.py
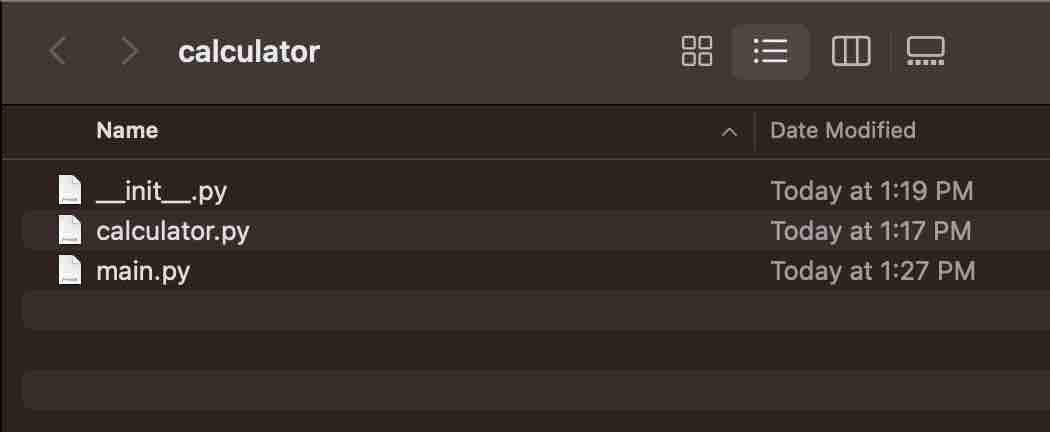
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- Force Gradle to use specific Java JDK Version - Gradle
- Twitter is down? Issues with Tweet create events affecting APIs - Twitter
- How to do screen recording on Mac - MacOS
- Hyperlink in html (anchor tag) without a underline - Html
- Android : Remove ListView Separator/divider programmatically or using xml property - Android
- Steps to Install Jenkins on M1/M2 Mac - MacOS
- Fix [oh-my-zsh] Cant update: not a git repository - Git
- How to Configure GitHub with Eclipse IDE in 2023 - Eclipse