To add a progress bar in the console for a Python program we need to make use of the tqdm module - the most rich and famous python progress bar library.
Make sure to get the tqdm module downloaded using the pip installer.
pip install tqdm
Details:
pip show tqdm
Name: tqdm
Version: 4.65.0
Summary: Fast, Extensible Progress Meter
Home-page: https://tqdm.github.io
Author:
Author-email:
License: MPLv2.0, MIT Licences
Location: /opt/homebrew/lib/python3.11/site-packages
Requires:
Required-by: spacy
Example 1: using for loop and time.sleep function
from tqdm import tqdm
import time
for i in tqdm(range(5)):
time.sleep(1)
Demo of the progress bar in jupyter notebook (Google Colab):
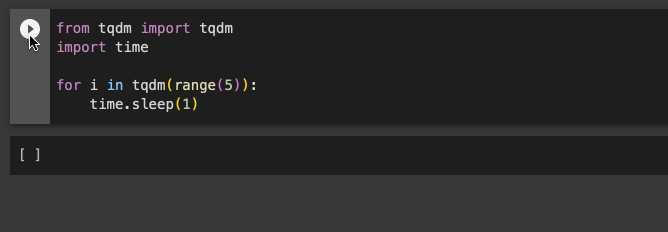
Example 2: Progress bar while loop
from tqdm import tqdm
import time
total_iterations = 5
progress_bar = tqdm(total=total_iterations, desc="Progress", bar_format="{l_bar}{bar}{r_bar}")
iteration = 0
while iteration < total_iterations:
time.sleep(1)
progress_bar.update(1)
iteration += 1
progress_bar.close()
Output:
Progress: 0%| | 0/5 [00:00<?, ?it/s]
Progress: 20%|โโ | 1/5 [00:01<00:04, 1.00s/it]
Progress: 40%|โโโโ | 2/5 [00:02<00:03, 1.00s/it]
Progress: 60%|โโโโโโ | 3/5 [00:03<00:02, 1.00s/it]
Progress: 80%|โโโโโโโโ | 4/5 [00:04<00:01, 1.01s/it]
Progress: 100%|โโโโโโโโโโ| 5/5 [00:05<00:00, 1.01s/it]
There is a lot you can do with the progress bar like setting the bar height, width, multiprocessing, changing color, text, and animation, for that you can follow the documentation on GitHub.
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- Python - Convert float to String - Python
- Java: Convert String to InputStream - Java
- Best free Decompiler for Java - Java
- Setting up RSS feeds notifications within Outlook - HowTos
- Add Buttons at the bottom of Android Layout xml file - Android
- How to configure Maven for Java 8/11/17 - Java
- Error:The SDK Build Tools revision (XX.X.X) is too low for project. Minimum required is XX.X.X - Android
- adb: The Android Debug Bridge and Commands - Android