There are a few ways in which we can comment out a block of code in Python, let's take a look at them with examples.
Example 1: Triple Quotes - Multi-line Comments (''')
'''
def add_numbers(a, b):
no1 = a
no2 = b
sum = no1 + no2
return sum
'''
def diff_numbers(a, b):
no1 = a
no2 = b
diff = no2 - no1
return diff
result = diff_numbers(5, 10)
print("Result:", result)
Example 2: Single line Comments (using #)
# def add_numbers(a, b):
# no1 = a
# no2 = b
# sum = no1 + no2
# return sum
def diff_numbers(a, b):
no1 = a
no2 = b
diff = no2 - no1
return diff
result = diff_numbers(1, 2)
print("Result:", result)
Comment/Uncomment Code using IDE Keyboard Shortcuts:
# def add_numbers(a, b):
# no1 = a
# no2 = b
# sum = no1 + no2
# return sum
def diff_numbers(a, b):
no1 = a
no2 = b
diff = no2 - no1
return diff
result = diff_numbers(1, 2)
print("Result:", result)
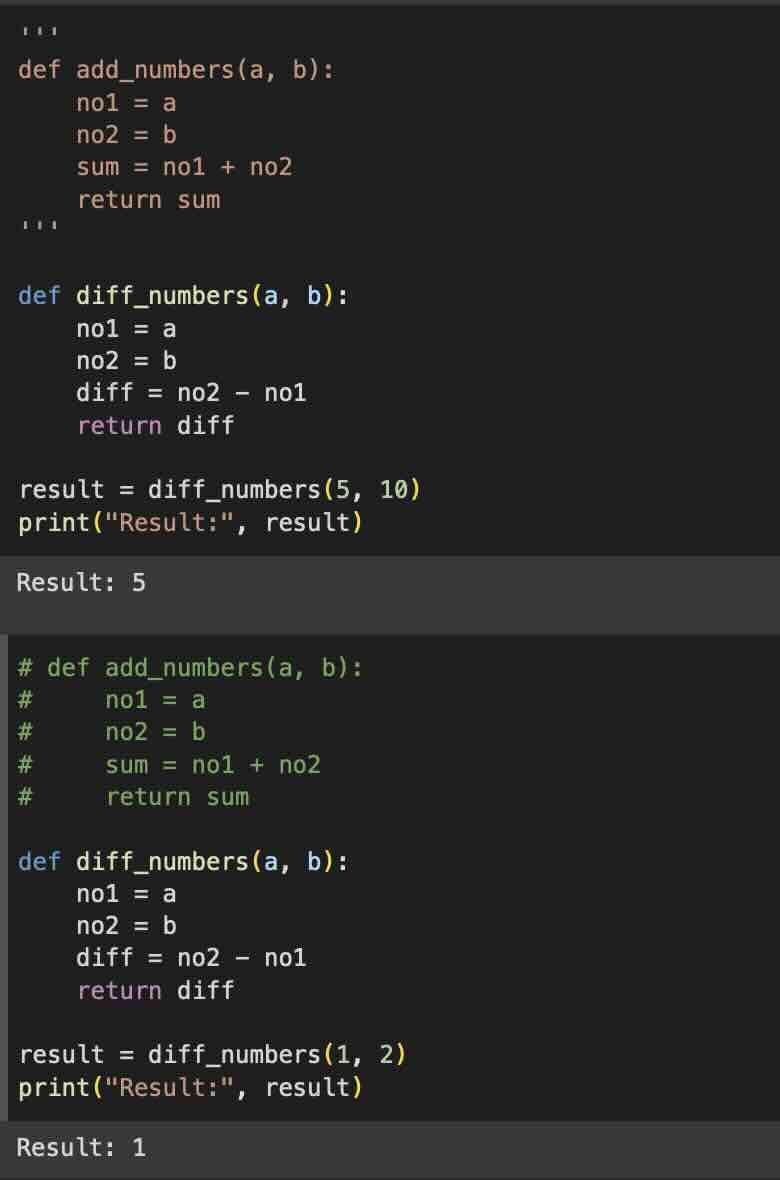
IDE | Comment Code (Mac) | Comment Code (Windows) | Uncomment Code (Mac) | Uncomment Code (Windows) |
---|---|---|---|---|
Visual Studio Code | ⌘ + / | Ctrl + / | ⌘ + / | Ctrl + / |
PyCharm | ⌘ + / | Ctrl + / | ⌘ + / | Ctrl + / |
Spyder | ⌘ + 1 | Ctrl + 1 | ⌘ + 1 | Ctrl + 1 |
Sublime Text | ⌘ + / | Ctrl + / | ⌘ + / | Ctrl + / |
Atom | ⌘ + / | Ctrl + / | ⌘ + / | Ctrl + / |
Jupyter Notebook | ⌘ + / | Ctrl + / | ⌘ + / | Ctrl + / |
IDLE | ⌘ + 3 | Ctrl + 3 | ⌘ + 4 | Ctrl + 4 |
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- How to Change Encoding of File from ANSI to UTF-8 in Windows Notepad - Windows
- How to zoom-in or zoom-out in Windows Notepad - NotepadPlusPlus
- How to install Python 3.9 using brew on Mac - Python
- Fix: error: non-static type variable T cannot be referenced from a static context - Java
- How to get Spotify macOS App installed on Mac device - MacOS
- List of Programming Languages Supported by Notepad++ - NotepadPlusPlus
- How to Open and Use Microsoft Edge Console - Microsoft
- Java 8: Stream map with Code Examples - Java