If you have a for loop in your Python code, and you want to exit it on a specific condition, then you can make use of the break statement.
This is just like other programming languages like C, C++, C# or Java.
Example:check_prime_numbers = [1, 2, 5, 6, 8, 9, 11, 12, 17]
for num in check_prime_numbers:
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
print(f"{num} is not a prime number")
break
else:
print(f"{num} is a prime number")
The above prime number example is a perfect one to understand how to make use of the break statement. We have a list of numbers and we want to know if they are prime or not. As soon as in the nested for loop we understand that the number is not prime we break the loop.
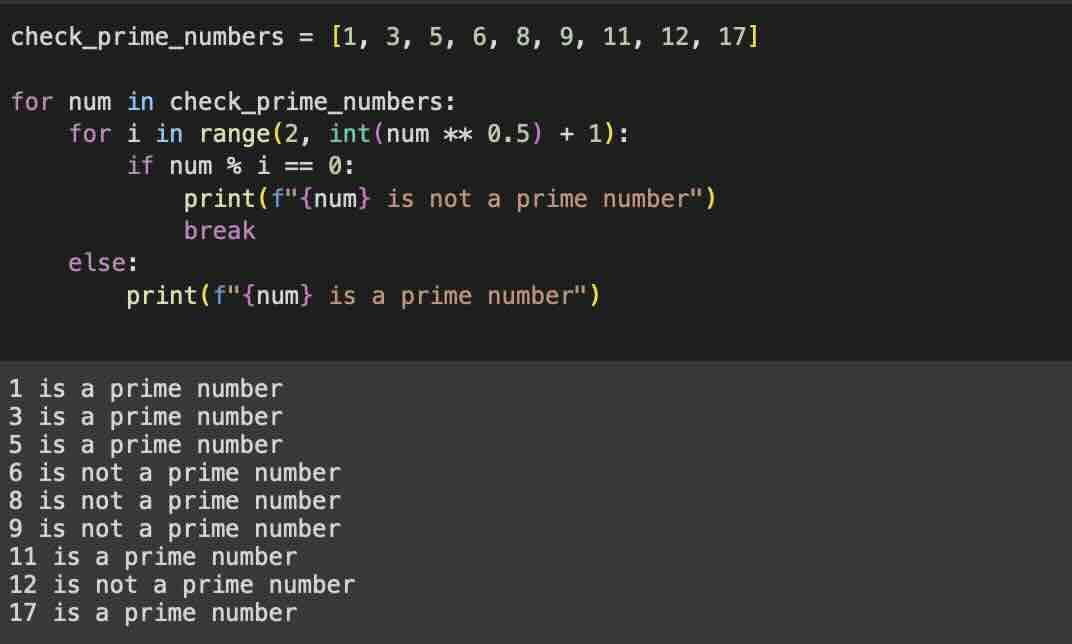
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
- Save Screenshots on Mac in JPG instead of PNG Format - MacOS
- Use 5G Network on Android Emulator - Android
- How to find path of file on Mac Terminal - MacOS
- Android Studio: Cannot perform refactoring operation - Android-Studio
- Use Google Chrome Canvas to create drawings - Chrome
- Disable EditText Cursor Android - Android
- How to Open PowerShell on Mac? - Powershell
- Robinhood unexpected server error - Android