In Python pandas, we can perform an outer join between two DataFrames using the pandas.merge() function and by setting the parameter how as outer.
Let's take a look by an example.
Example: Pandas Outer Join
import pandas as pd
data_city_NYC = {
'Temp_NYC': [25, 30, 27],
'Humidity_NYC': [50, 45, 55]
}
data_city_Chicago = {
'Temp_Chicago': [28, 32, 29],
'Humidity_Chicago': [60, 58, 62]
}
dates_NYC = pd.date_range(start='2023-07-01', periods=3, freq='D')
dates_Chicago = pd.date_range(start='2023-07-04', periods=3, freq='D')
df_city_NYC = pd.DataFrame(data_city_NYC, index=dates_NYC)
df_city_Chicago = pd.DataFrame(data_city_Chicago, index=dates_Chicago)
print("DataFrame: NYC City")
print(df_city_NYC)
print("\nDataFrame: Chicago City")
print(df_city_Chicago)
# Outer join on the index (date)
outer_merged_cities_df = pd.merge(df_city_NYC, df_city_Chicago, left_index=True, right_index=True, how='outer')
print("\nOuter Joined DataFrame Data:")
print(outer_merged_cities_df)
Output:
DataFrame: NYC City
Temp_NYC Humidity_NYC
2023-07-01 25 50
2023-07-02 30 45
2023-07-03 27 55
DataFrame: Chicago City
Temp_Chicago Humidity_Chicago
2023-07-04 28 60
2023-07-05 32 58
2023-07-06 29 62
Outer Joined DataFrame Data:
Temp_NYC Humidity_NYC Temp_Chicago Humidity_Chicago
2023-07-01 25.0 50.0 NaN NaN
2023-07-02 30.0 45.0 NaN NaN
2023-07-03 27.0 55.0 NaN NaN
2023-07-04 NaN NaN 28.0 60.0
2023-07-05 NaN NaN 32.0 58.0
2023-07-06 NaN NaN 29.0 62.0
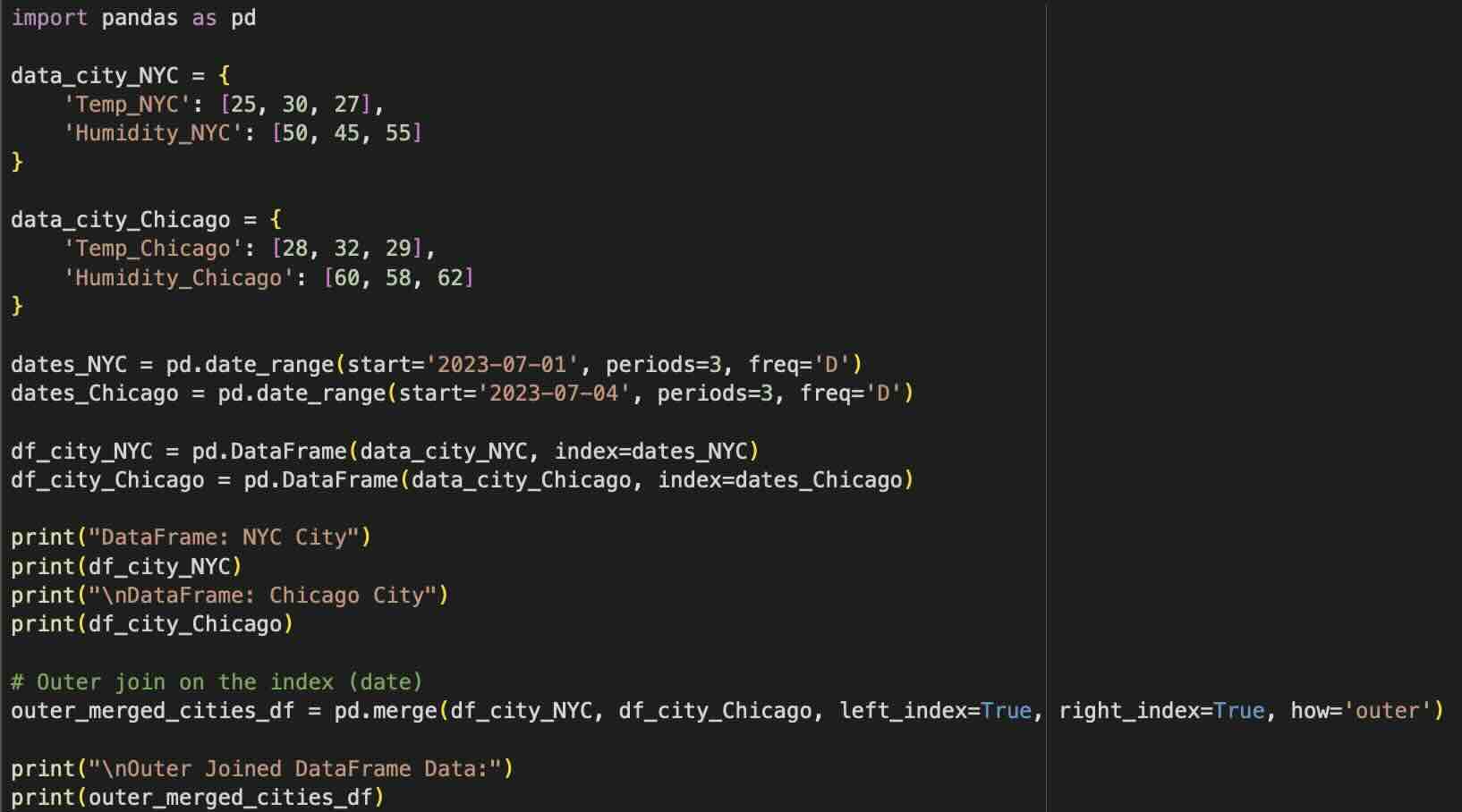
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- macOS Big Sur java.lang.UnsatisfiedLinkError CoreFoundation - Android Studio - Android-Studio
- Best Free Gif screen capture app now available for M1 Chip Mac - LICECap - MacOS
- [fix] iCloud - Verification Failed: An unknown error occurred Apple ID - iOS
- Java + Spring JDBC Template + Gradle Example - Java
- Fix: ModuleNotFoundError: No module named boto3 [Python] - Python
- Fix: This app is no longer shared with you error iPhone or iPad iOS - HowTos
- How to turn off Stage Manager - macOS Ventura - MacOS
- Read Java JDBC Connection Details from Properties File - Java