Working with files in Python Programming is a common scenario, there are various packages that you can make use of in order to get the current directory, but the most widely used one is the os module.
Using os module
To get the current working directory make use of os.getcwd() method from the os module.
Example 1:import os
current_working_directory = os.getcwd()
print(current_working_directory)
Output:
/Users/code2care/Desktop
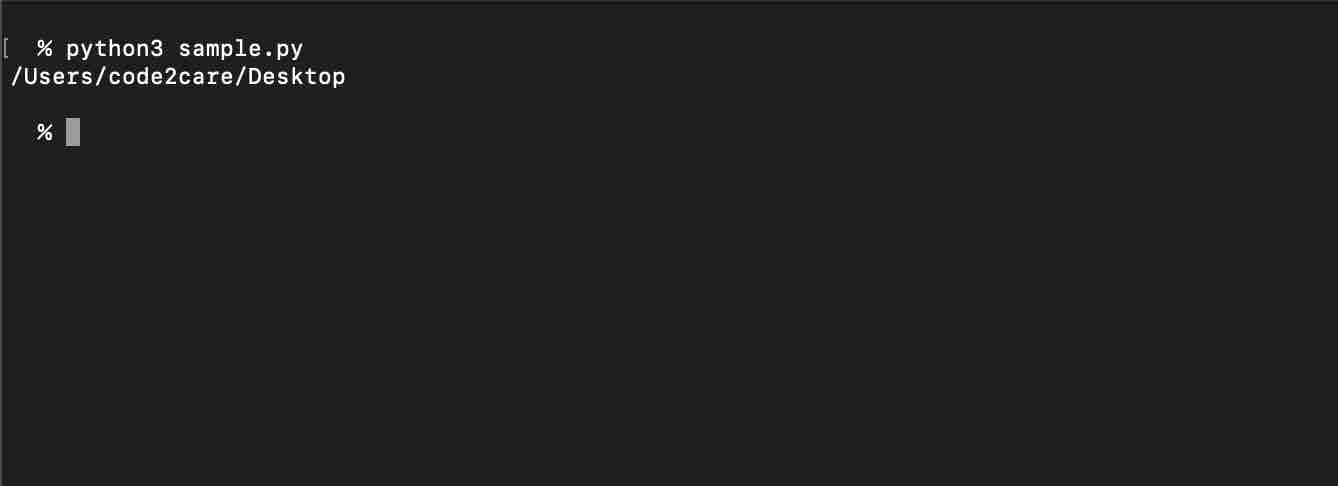
import os
present_working_directory=os.system("pwd")
print(present_working_directory)
Example 3: Using Terminal
% python3
Python 3.10.8 (main, Oct 21 2022, 22:22:30) [Clang 14.0.0 (clang-1400.0.29.202)] on darwin
Type "help", "copyright", "credits" or "license" for more information.
>>> os.getcwd()
Traceback (most recent call last):
File "<stdin>", line 1, in
NameError: name 'os' is not defined
>>> import os
>>> print(os.getcwd())
/Users/code2care/Desktop
Using pathlib module
Example 4:from pathlib import Path
path = Path.cwd();
print(path)
Output:
/Users/code2care/Documents
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- Spring Boot: Transactions Management with JDBCTemplate Example - Java
- 30+ Zoom video communications application shortcuts for macOS - MacOS
- Java JDK 21 LTS Version Release Date (General Availability) - Java-JDK-21
- Add Blank Lines Between Each Lines in Notepad++ - NotepadPlusPlus
- Share Multiple Images in WhatsApp using Android Intent - WhatsApp
- The default interactive shell is now zsh. [macOS] - MacOS
- How to Download Microsoft Excel on Mac - MacOS
- How to URLEncode a Query String in Python - Python