Switch statements in PowerShell are really useful to design a script with a menu, and to perform actions based on the selection.
Let's take a look at a few examples.
Example1 :
<#
PowerShell Switch Statement Example
Determine Day of the Week
#>
# User Input
$number = Read-Host "Enter a number from 1 to 7"
switch ($number) {
1 {
Write-Host "Day 1: Sunday"
}
2 {
Write-Host "Day 2: Monday"
}
3 {
Write-Host "Day 3: Tuesday"
}
4 {
Write-Host "Day 4: Wednesday"
}
5 {
Write-Host "Day 5: Thursday"
}
6 {
Write-Host "Day 6: Friday"
}
7 {
Write-Host "Day 7: Saturday"
}
default {
Write-Host "Invalid number. Please enter a number from 1 to 7."
}
}
Example 2:
# Menu Options
Write-Host "AI Operations Menu:"
Write-Host "1. Stable Diffusion"
Write-Host "2. AI Analysis"
Write-Host "3. Exit"
$choice = Read-Host "Enter Menu Option 1 - 3"
$choice = [int]$choice
switch ($choice) {
1 {
Write-Host "You selected: Stable Diffusion"
}
2 {
Write-Host "You selected: AI Analysis"
}
3 {
Write-Host "Exiting.."
}
default {
Write-Host "Invalid choice. Please enter a valid option (1, 2, or 3)."
}
}
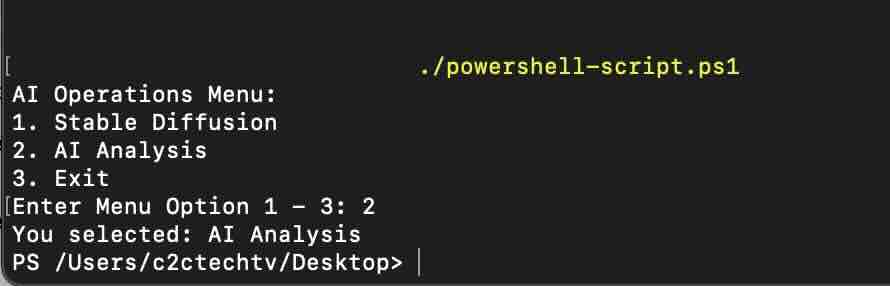
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Powershell,
- How to upgrade PowerShell on Mac
- How to install AWS CLI 2 on Windows 11 using PowerShell
- How to switch to Powershell on Mac Terminal
- How to connect to Microsoft Exchange Online using PowerShell
- How to Check PowerShell Version? [Windows/Mac/Linux]
- How to Comment out Code in PowerShell Script
- How to Identify installed PowerShell version
- How to install PowerShell on macOS
- Open PowerShell Terminal in Visual Studio Code (VSCode)
- PowerShell ps1 script is not digitally signed, you cannot run this script on the current system
- Update Powershell Using Command Line
- PowerShell 1..10 foreach Example
- PowerShell Fix: Get-Help cannot find the Help files for this cmdlet on this computer
- List of PowerShell Cmdlet Commands for Mac
- Help or Man equivalent in PowerShell
- PowerShell SubString with Examples
- PowerShell: How to Get Folder Size
- PowerShell Traditional For Loop Example
- PowerShell Switch Statement with Examples
- PowerShell Concatenate String Examples
- PowerShell For Each Loop Examples
- PowerShell: Grep Command Alternative - Select-String
- How to delete a file using PowerShell [Windows/macOS]
- Fix: nano is not recognized as an internal or external command - Windows PowerShell
- PowerShell on Mac: The term get-service is not recognized as a name of a cmdlet, function, script file, or executable program
More Posts:
- Enable Dark Mode in Google Search - Google
- How to show line numbers in Nano on Mac - MacOS
- How to resolve Failed to create interpreter PyCharm Error - Python
- PowerShell: Steps to Connect to connect to Exchange Online - Powershell
- Microsoft Teams Error - You cannot add another work account to Teams at the moment - Teams
- Python matplotlib segmentation fault: 11 macOS Big Sur - Python
- What is the doctype for HTML5? - Html
- [Eclipse] Syntax error, annotations are only available if source level is 1.5 or greater - Eclipse