The concept of slicing in Python makes it very efficient to work with strings, lists, or tuples to extract a section from them with the help of range and indices.
Syntax:sequence[start:end:step]
Let's try to understand each of the parameters here,
Parameter | Description | Default Value |
---|---|---|
start | Starting index of the slice (inclusive) | 0 |
end | Ending index of the slice (exclusive) | Length of sequence |
step | Step size between elements in the slice | 1 |
Slicing with Strings Example:
Now let's start with a simple example of a string as a sequence.
sequence = "Let's try to learn sequences in Python!"
Slice | Result |
---|---|
'Let's' | Start: 0, End: 6, Step: 1 |
'sequences in Python!' | Start: 19, End: None, Step: 1 |
'to learn' | Start: 8, End: 16, Step: 1 |
'Let's try to learn sequences' | Start: 0, End: 23, Step: 1 |
'Python!' | Start: 25, End: None, Step: 1 |
'Let's in!' | Start: 0, End: 14, Step: 2 |
'!' | Start: -1, End: None, Step: 1 |
'Pytse ie' | Start: 26, End: 5, Step: -1 |
Once you get an understanding of how to slice what substring you are looking for, we can convert it into code.
Code:
sequence = "Let's try to learn sequences in Python!"
print(sequence[0:6]) # Result: "Let's"
print(sequence[19:]) # Result: "sequences in Python!"
print(sequence[8:16]) # Result: "to learn"
print(sequence[0:28]) # Result: "Let's try to learn sequences"
print(sequence[32:]) # Result: "Python!"
print(sequence[0:14:8]) # Result: "Ly"
print(sequence[-1:]) # Result: "!"
print(sequence[26:5:-1]) # Result: "ecneuqes nrael ot yrt"
Slicing with Lists Example:
Slice | Result |
---|---|
"Let's" | Start: 0, End: 6, Step: 1 |
"sequences in Python!" | Start: 19, End: None, Step: 1 |
"to learn" | Start: 8, End: 16, Step: 1 |
"Let's try to learn sequences" | Start: 0, End: 28, Step: 1 |
"Python!" | Start: 32, End: None, Step: 1 |
"Ly" | Start: 0, End: 14, Step: 8 |
"!" | Start: -1, End: None, Step: 1 |
"ecneuqes nrael ot yrt" | Start: 26, End: 5, Step: -1 |
Code:
list = [0.596, 0.105, 0.782, 0.421, 0.914, 0.290, 0.682, 0.813]
import random
decimal_numbers = [random.random() for _ in range(8)]
print(decimal_numbers) # Result: [0.596, 0.105, 0.782, 0.421, 0.914, 0.290, 0.682, 0.813]
print(decimal_numbers[0:]) # Result: [0.596, 0.105, 0.782, 0.421, 0.914, 0.290, 0.682, 0.813]
print(decimal_numbers[3:7]) # Result: [0.421, 0.914, 0.290, 0.682]
print(decimal_numbers[0:7:3]) # Result: [0.596, 0.782, 0.290]
print(decimal_numbers[-1::-1]) # Result: [0.813, 0.682, 0.290, 0.914, 0.421, 0.782, 0.105, 0.596]
print(decimal_numbers[-5:-1]) # Result: [0.105, 0.290, 0.682, 0.782]
print(decimal_numbers[3::2]) # Result: [0.421, 0.290, 0.105]
print(decimal_numbers[2:0:-1]) # Result: [0.682, 0.290, 0.421, 0.782]
print(decimal_numbers[4::-1]) # Result: [0.914, 0.421, 0.782, 0.105, 0.596]
Slicing with tuple Example:
cities = ('New York', 'Paris', 'London', 'Tokyo')
Slice | Result |
---|---|
('New York',) | Start: 0, End: 1, Step: 1 |
('Paris', 'London') | Start: 1, End: None, Step: 1 |
('Tokyo',) | Start: 2, End: 3, Step: 1 |
('New York', 'Paris', 'London', 'Tokyo') | Start: 0, End: None, Step: 1 |
('Tokyo', 'Paris') | Start: 2, End: None, Step: -1 |
() | Start: None, End: None, Step: None |
('London', 'Paris', 'New York') | Start: -1, End: None, Step: -1 |
('Paris', 'London') | Start: 1, End: 3, Step: 1 |
Code:
cities = ('New York', 'Paris', 'London', 'Tokyo')
print(cities[0:1]) # Result: ('New York',)
print(cities[1:]) # Result: ('Paris', 'London', 'Tokyo')
print(cities[2:3]) # Result: ('London',)
print(cities[0:]) # Result: ('New York', 'Paris', 'London', 'Tokyo')
print(cities[3:1:-1]) # Result: ('Tokyo', 'Paris')
print(cities[:]) # Result: ('New York', 'Paris', 'London', 'Tokyo')
print(cities[-1:]) # Result: ('Tokyo',)
print(cities[-2:-4:-1]) # Result: ('London', 'Paris')
Some more examples:
Slice | Result | Description |
---|---|---|
sequence[:] | Entire sequence | Returns a new sequence that is a copy of the entire sequence. |
sequence[::] | Entire sequence (equivalent to sequence[:]) | Returns a new sequence that is a copy of the entire sequence. |
sequence[::2] | Every second item | Returns a new sequence containing every second item. |
sequence[1::2] | Every second item starting at index 1 | Returns a new sequence containing every second item starting at index 1. |
sequence[::-1] | Reversed sequence | Returns a new sequence with items in reverse order. |
sequence[:5] | First five items | Returns a new sequence containing the first five items. |
sequence[-5:] | Last five items | Returns a new sequence containing the last five items. |
sequence[2:7] | Items from index 2 to 6 | Returns a new sequence containing items from index 2 to 6. |
sequence[1:-1] | Everything except the first and last items | Returns a new sequence excluding the first and last items. |
sequence[::3] | Every third item | Returns a new sequence containing every third item. |
sequence[:-2] | Everything except the last two items | Returns a new sequence excluding the last two items. |
sequence[-3:-1] | Second-last and third-last items | Returns a new sequence containing the second-last and third-last items. |
sequence[1:9:2] | Every second item from index 1 to 8 | Returns a new sequence containing every second item from index 1 to 8. |
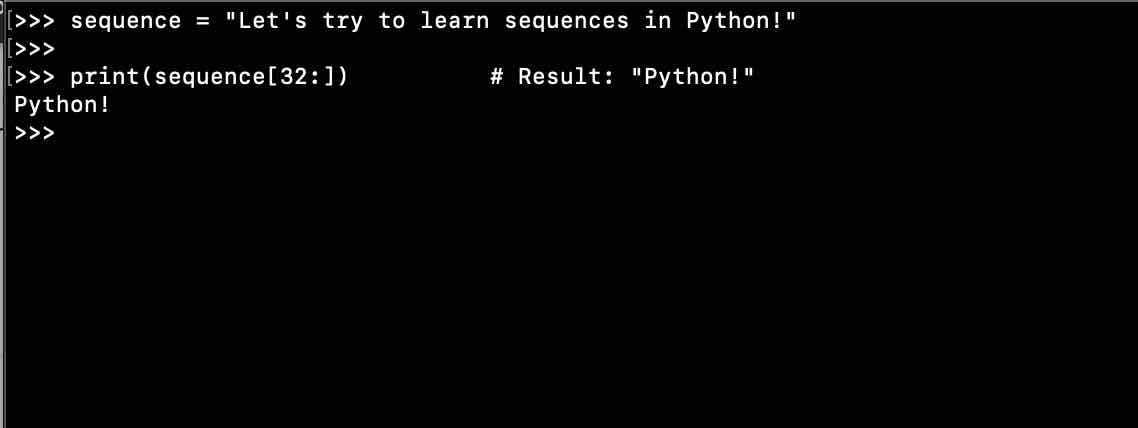
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- How to kill tomcat server process using Mac Terminal Command - Tomcat
- How to run .bat file on Mac - MacOS
- Fix: chroot: failed to run command /bin/bash: No such file or directory - Bash
- Fix: ModuleNotFoundError: No module named pandas - Python
- Fix: line 1: import: command not found Python - Python
- Quickly install Apache Server on Ubuntu Linux - Ubuntu
- Online JSON Validator Tool - Tools
- Enable spell check in Sublime Text (macOS) - MacOS