In the above example, we had taken a look at how to rename column(s) of a DataFrame in pandas, and now in this example, we see how to rename the index names.
Again, we will make use of the DataFrame.rename function.
Example:
import pandas as pd
code2care_data = {
'Year': [2020, 2021, 2022, 2023],
'Country': ['United States', 'India', 'United Kingdom', 'Germany'],
'City': ['New York', 'Mumbai', 'London', 'Berlin'],
'Mobile': [312125, 2234010, 150125, 413822],
'Desktop': [353871, 427896, 374675, 258976],
'Search Engine': ['Google', 'Bing', 'Google', 'Yahoo']
}
df = pd.DataFrame(code2care_data)
print("\nDataFrame before renaming Column Names:")
print(df)
# Renaming the indexes
new_index_names = {
0: 'Row 1',
1: 'Row 2',
2: 'Row 3',
3: 'Row 4'
}
df = df.rename(index=new_index_names)
print("\nDataFrame after renaming Index:")
print(df)
Output:
DataFrame before renaming Index Names:
Year Country City Mobile Desktop Search Engine
0 2020 United States New York 312125 353871 Google
1 2021 India Mumbai 2234010 427896 Bing
2 2022 United Kingdom London 150125 374675 Google
3 2023 Germany Berlin 413822 258976 Yahoo
DataFrame after renaming Indexes:
Year Country City Mobile Desktop Search Engine
Row 1 2020 United States New York 312125 353871 Google
Row 2 2021 India Mumbai 2234010 427896 Bing
Row 3 2022 United Kingdom London 150125 374675 Google
Row 4 2023 Germany Berlin 413822 258976 Yahoo
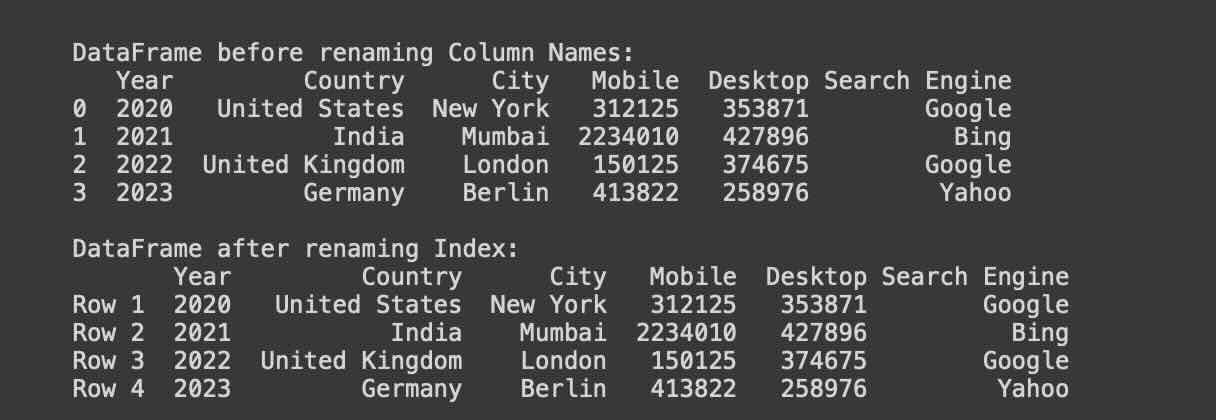
Read More: https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.rename.html
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- How to Enable or Disable Dark Mode on macOS Ventura 13 - MacOS
- Notepad++: How to add Quotes to CSV File - NotepadPlusPlus
- Binary, Decimal, Octal, and Hexadecimal Numbers Tables - 2022
- Create Hidden File or Directory using Shell Command - Linux
- Git: Delete Branch Locally and Remotely at Origin - Git
- How to declare Arrays in Java with Examples - Java
- How to check the version of NodeJS installed - HowTos
- Fix: ReferenceError: require is not defined in ES module scope [Node] - JavaScript