If you want to rename the column names of a DataFrame, you can make use of DataFrame.rename function from the pandas module.
Let's take a look at some code examples:
Example 1:
>>> import pandas as pd
>>> df = pd.DataFrame({"Column1": ["2023-01",32.2], "Column2": ["2023-02", 31.1]})
>>> df.rename(columns={"Column1": "Year-Month", "Column2": "Temperature"})
Year-Month Temperature
0 2023-01 2023-02
1 32.2 31.1
Example 2:
import pandas as pd
code2care_data = {
'Year': [2020, 2021, 2022, 2023],
'Country': ['United States', 'India', 'United Kingdom', 'Germany'],
'City': ['New York', 'Mumbai', 'London', 'Berlin'],
'Mobile': [312125, 2234010, 150125, 413822],
'Desktop': [353871, 427896, 374675, 258976],
'Search Engine': ['Google', 'Bing', 'Google', 'Yahoo']
}
df = pd.DataFrame(code2care_data)
print("\nDataFrame before renaming Column Names:")
print(df)
# Renaming the columns
new_column_names = {
'Country': 'Visitor Country',
'City': 'Visitor City',
'Mobile': 'Mobile Visits',
'Desktop': 'Desktop Visits',
'Search Engine': 'Search Engine Source'
}
df = df.rename(columns=new_column_names)
print("\nDataFrame after renaming Column Names:")
print(df)
Output:
DataFrame before renaming Column Names:
Year Country City Mobile Desktop Search Engine
0 2020 United States New York 312125 353871 Google
1 2021 India Mumbai 2234010 427896 Bing
2 2022 United Kingdom London 150125 374675 Google
3 2023 Germany Berlin 413822 258976 Yahoo
DataFrame after renaming Column Names:
Year Visitor Country Visitor City Mobile Visits Desktop Visits \
0 2020 United States New York 312125 353871
1 2021 India Mumbai 2234010 427896
2 2022 United Kingdom London 150125 374675
3 2023 Germany Berlin 413822 258976
Search Engine Source
0 Google
1 Bing
2 Google
3 Yahoo
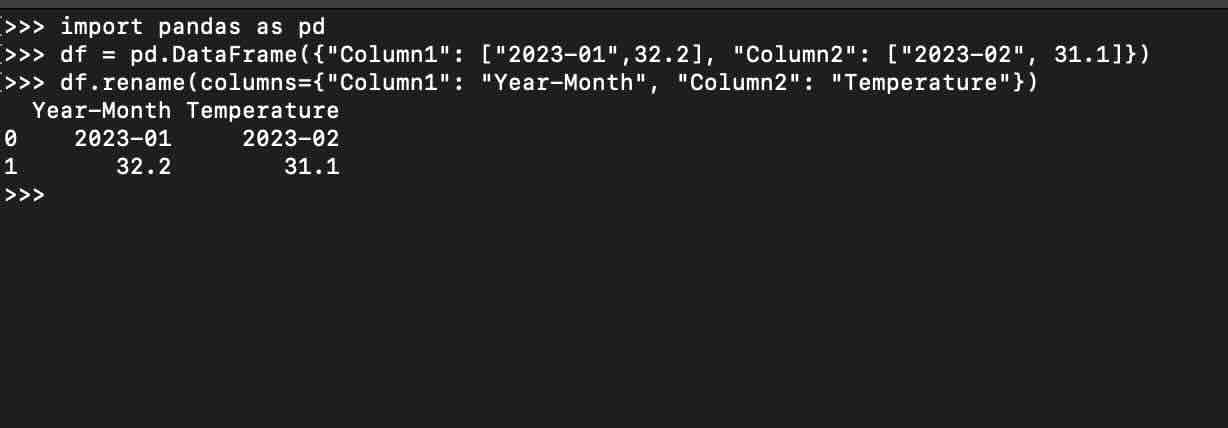
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- How to add ruler in Sublime Text tab window - Sublime-Text
- Binary, Decimal, Octal, and Hexadecimal Numbers Tables - 2022
- Stop a Running Command on macOS Terminal - MacOS
- How to Convert CSV file to SQL Script using Notepad++ - NotepadPlusPlus
- Pandas: Reading .xlsx or .xls Excel Files - Python
- [Fix] java.net.MalformedURLException: unknown protocol - Java
- Clone a particular remote brach using git clone command - Git
- Convert JSON to Java Collection Map using Jackson - Java