To remove quotes from within a String in Python, we can make use of the functions such as strip(), re and replace().
Example 1: Remove Leading and Trailing Single Quotes using strip()
str_with_single_quotes = "'Hello there! How are you?'"
str_without_single_quotes = str_with_single_quotes.strip("'")
print(str_without_single_quotes)
Output:
Hello there! How are you?
Example 2: Remove Leading and Trailing Double Quotes using regex - re module
import re
str_with_single_quotes = "He said \"I am not coming!\""
str_without_single_quotes = re.sub(r"\"", "", str_with_single_quotes)
print(str_without_single_quotes)
Output:
He said I am not coming!
Example 3: Using replace() function to repalce both single and double quotes
import re
str_with_single_quotes = "\"This isn't mine\""
str_without_single_quotes = str_with_single_quotes.replace("'", "").replace("\"", "")
print(str_without_single_quotes)
Output:
This isnt mine!
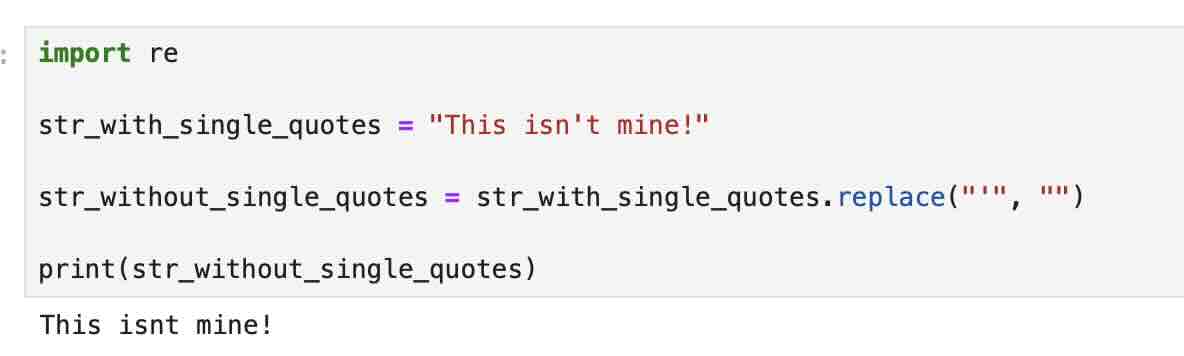
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- Your Android SDK is missing, out of date or corrupted SDK Problem - Android-Studio
- Add Line Number before each line in Notepad++ using Column Editor - NotepadPlusPlus
- Fix - bash: man: command not found - Linux
- Install Visual Studio VS Code on Mac using Brew - MacOS
- How to check about details of Notepad++ text editor - NotepadPlusPlus
- MySQL Error :1007 SQLSTATE: HY000 (ER_DB_CREATE_EXISTS) Message: Can't create database '%s'; database exists - MySQL
- Unbound classpath container: JRE System Library [JavaSE-1.7] - Java
- Add comma or semicolon at end of each line Notepad++ - NotepadPlusPlus