If you have a list in Python and you want to search to see if a particular element is present in the list, you can make use of the one of the following three ways,
1. Using the "in" and "not in" operator
Example:list_of_cities = ["NYC", "Tokyo", "Chicago", "Austin", "London"]
if "London" in list_of_cities:
print(f"London is the list {list_of_cities}")
if "Mumbai" not in list_of_cities:
print(f"Mumbai is not the list {list_of_cities}")
Output:
London is the list ['NYC', 'Tokyo', 'Chicago', 'Austin', 'London']
Mumbai is not the list ['NYC', 'Tokyo', 'Chicago', 'Austin', 'London']
2. Using the index() method
Example:my_list = ["Apple", "Mango", "Banana", "Grapes"]
try:
index = my_list.index("Mango")
print("Mango is at index", index)
except ValueError:
print("Mango is not in the list")
Mango is at index 1
Its better to handle ValueError as if the element you are looking for is not found you get this error.
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
<ipython-input-42-3b37ee759fe2> in <cell line: 3>()
1 my_list = ["Apple", "Mango", "Banana", "Grapes"]
2
----> 3 index = my_list.index("Orange")
4 print("Mango is at index", index)
ValueError: 'Orange' is not in list
3. Using the count() method
Example:my_list = [24,21,12,45,24,12,45,23]
if my_list.count(10) > 0:
print("10 is found in my_list")
else:
print("10 is not found in my_list")
10 is not found in my_list
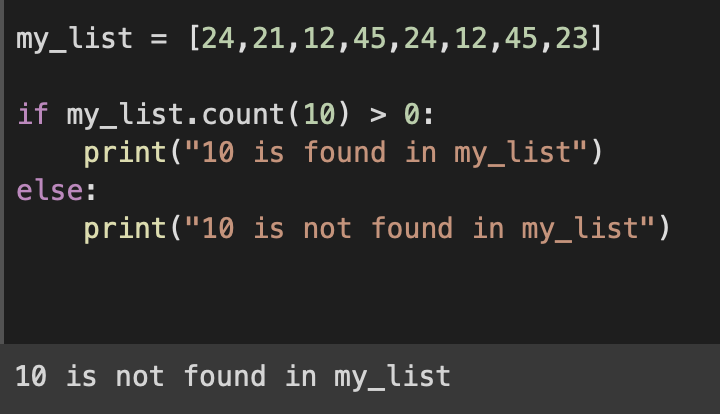
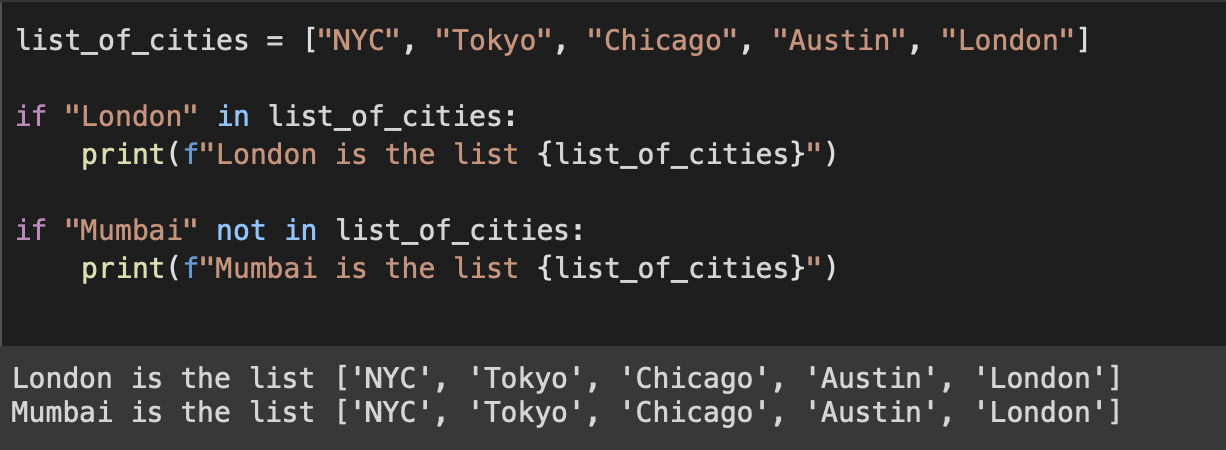
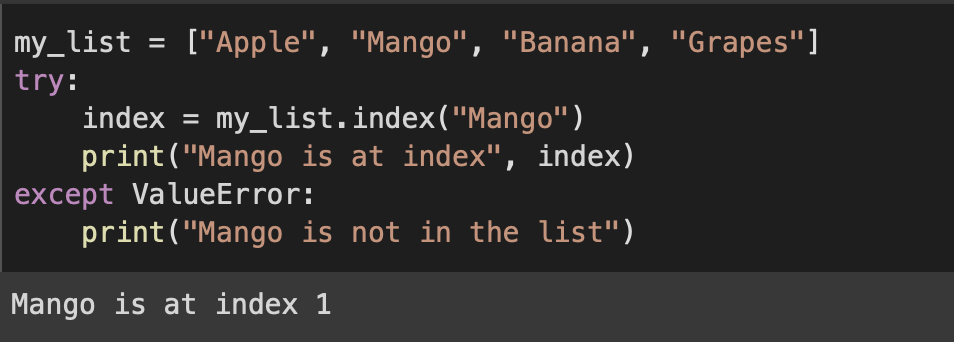
HashTags: #searchAListPython #pythonFindInList #listSearchPython #pythonFindElementInList #pythonFindItemInList
References:
- https://docs.python.org/3/library/array.html?highlight=index#array.array.index- https://docs.python.org/3/library/array.html?highlight=index#array.array.count
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
- Where does Notepad++ save temp files? - NotepadPlusPlus
- Drag drop files here option missing for SharePoint document library - SharePoint
- How to install Python Specific version (3.8, 3.9 or 3.10) using Brew - Python
- PHP Warning: Cannot modify header information - headers already sent - PHP
- Java: Convert a Text file into Array of Bytes Example - Java
- Whats new in Python 3.10 Pre-release - Python
- 5 Reasons Why Jupyter Notebook is Not Opening and Solutions - Python
- How to Pretty Print cURL JSON Output in Terminal - cURL