If you are using Java 7 java.nio classes to read a file, you may come across the NoSuchFileException if the file is not found. NoSuchFileException is a checked exception that was added to Java 7 and it extends FileSystemException that in turn extends IOException.
Example:
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.NoSuchFileException;
import java.nio.file.Paths;
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
String fileName = "/Users/proj/src/main/sample.txt";
try (Stream<String> linesStream = Files.lines(Paths.get(fileName))) {
linesStream.forEach(System.out::println);
} catch (NoSuchFileException ex) {
System.out.println("File not found:" + fileName);
ex.printStackTrace();
} catch (IOException ioe) {
ioe.printStackTrace();
}
}
}
Exception stack trace:
java.nio.file.NoSuchFileException: /Users/proj/src/main/sample.txt
at sun.nio.fs.UnixException.translateToIOException(UnixException.java:86)
at sun.nio.fs.UnixException.rethrowAsIOException(UnixException.java:102)
at sun.nio.fs.UnixException.rethrowAsIOException(UnixException.java:107)
at sun.nio.fs.UnixFileSystemProvider.newByteChannel(UnixFileSystemProvider.java:214)
at java.nio.file.Files.newByteChannel(Files.java:361)
at java.nio.file.Files.newByteChannel(Files.java:407)
at java.nio.file.spi.FileSystemProvider.newInputStream(FileSystemProvider.java:384)
at java.nio.file.Files.newInputStream(Files.java:152)
at java.nio.file.Files.newBufferedReader(Files.java:2784)
at java.nio.file.Files.lines(Files.java:3744)
at java.nio.file.Files.lines(Files.java:3785)
at JavaReadTextFile.main(JavaReadTextFile.java:13)
Fix/Solutions:
- Make sure you have provided the absolute path of the file.
- Make sure the file exists.
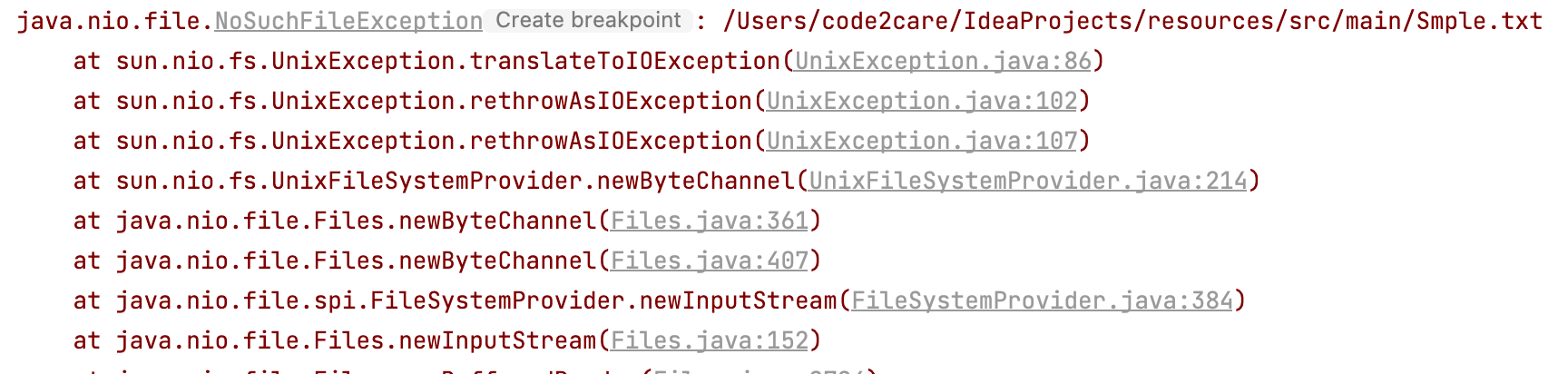
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Disable Control Scroll Zoom-in and Zoom-out in Notepad++ - NotepadPlusPlus
- Background task activation is spurious error - Windows 10, Office 2016, Office 365 - HowTos
- Fix: ModuleNotFoundError: No module named azure-core - Azure
- What is HTTP 500 Internal Server Error Code on web browsers - HowTos
- How to install curl on Alpine Linux - Linux
- Add X days from today in Command Line - HowTos
- Fix: >>> pip install - SyntaxError: invalid syntax - PIP
- Java TLSv1.3 protocol code example using SSLSocket - Java