Calculating SHA hash value of a file is really helpful for its data integrity.
You will notice that the software setups or files shared on the website that you download have their hash values displayed on the download pages. This hash can be used to compare the hash of the downloaded or transferred file to know if the downloaded file can be trusted, if they match, you can ensure that the file has not been tampered with during transmission or storage.
In this example, we take a look at how to calculate the SHA hash value for two files in Java.
import java.io.FileInputStream;
import java.io.IOException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Arrays;
/**
* Java example to Calculate SHA hash
* value of two files to see if they
* have the same content or not
*
* Author: Code2care.org
* Version: v1
* Date: 07/10/2023
*
*/
public class FileSHAComparisonExample {
private static final String SHA_ALGORITHM = "SHA-256";
private static final int BUFFER_SIZE = 8192;
public static void main(String[] args) {
String file1Path = "/Users/dev/IdeaProjects/basics/src/data_2023.csv";
String file2Path = "/Users/dev/IdeaProjects/basics/src/data_2024.csv";
try {
byte[] file1Hash = calculateSHA256(file1Path);
byte[] file2Hash = calculateSHA256(file2Path);
boolean areFilesEqual = Arrays.equals(file1Hash, file2Hash);
if (areFilesEqual) {
System.out.println("The files have the same content.");
} else {
System.out.println("The files have different content.");
}
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public static byte[] calculateSHA256(String filePath) throws IOException, NoSuchAlgorithmException {
MessageDigest messageDigest = MessageDigest.getInstance(SHA_ALGORITHM);
try (FileInputStream inputStream = new FileInputStream(filePath)) {
byte[] buffer = new byte[BUFFER_SIZE];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
messageDigest.update(buffer, 0, bytesRead);
}
}
return messageDigest.digest();
}
}
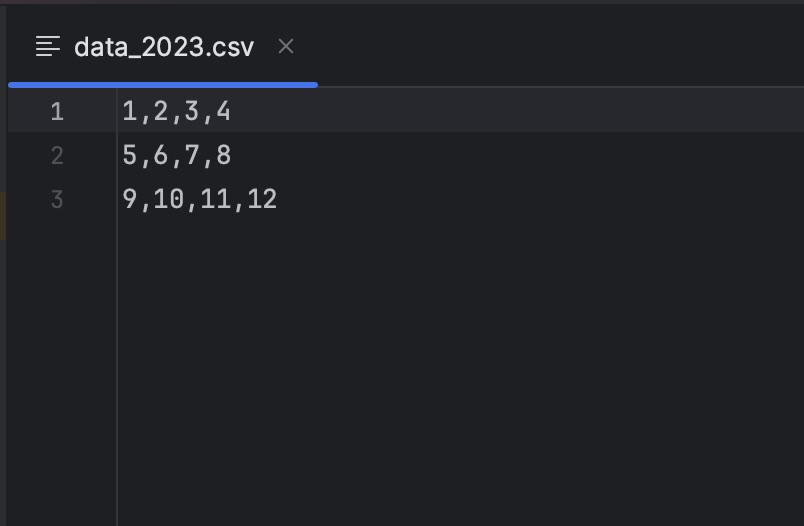
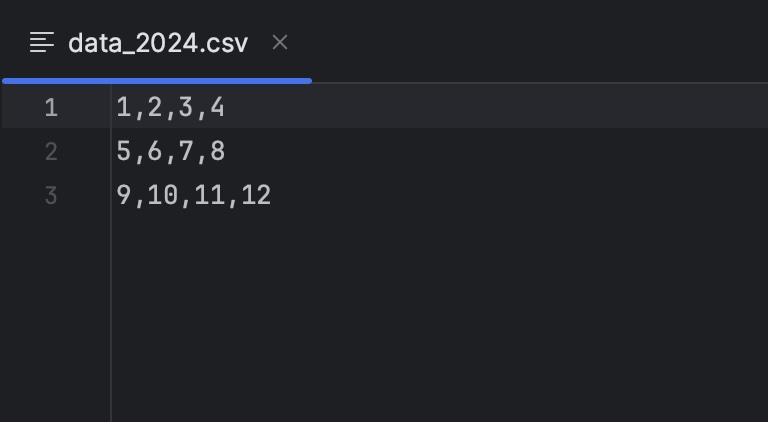
Output:
Now let's change the contents of one of the files and run the code again.
Output:
SHA Algorithm | Description | Hash Size |
---|---|---|
SHA-1 | Secure Hash Algorithm 1 | 160 bits |
SHA-224 | SHA-2 with 224-bit hash | 224 bits |
SHA-256 | SHA-2 with 256-bit hash | 256 bits |
SHA-384 | SHA-2 with 384-bit hash | 384 bits |
SHA-512 | SHA-2 with 512-bit hash | 512 bits |
SHA-512/224 | SHA-2 with 224-bit hash (512) | 224 bits |
SHA-512/256 | SHA-2 with 256-bit hash (512) | 256 bits |
SHA-3-224 | SHA-3 with 224-bit hash | 224 bits |
SHA-3-256 | SHA-3 with 256-bit hash | 256 bits |
SHA-3-384 | SHA-3 with 384-bit hash | 384 bits |
SHA-3-512 | SHA-3 with 512-bit hash | 512 bits |
Some examples of SHA hashes for the data_2023.csv file.
SHA-1 Hash: ffee510fa54db38070bf2fa9f07761e7666c1ebc
SHA-384 Hash: 07b73d610d6632556e195dc1687c6a3278ee14f588ca199598ed998065af1fc09f36acf20d05eb213b603c850497aaf2
SHA-224 Hash: ac322d5fb2c85630000952b0b428d95f4ea1d5469c461cca8e9205e1
SHA-256 Hash: 6171f17906ba66cb81255085fb2ef6203435549d41aeaf9c11640d410888ea23
SHA-512 Hash: 0fcb2d37e0877162cf870bf70143005cda762a5ee0e0f969a19406b029cf8926e53037a735ba76b9d8c3402f9002024995e0f5c3b4d301cb3bd8f22496552555
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- [fix] zsh: command not found: telnet on Mac - MacOS
- How to Share Microsoft SharePoint Site with Users or Groups - SharePoint
- [Interview Question] Can Constructors be Overloaded in Java? - Java
- Save webpage as pdf in Google Chrome for Mac OS X - Mac-OS-X
- IntelliJ: Error: Could not find or load main class, java.lang.ClassNotFoundException - Java
- Splitting String in Java with Examples - Java
- Installing Android Studio Dolphin on Mac with Apple (M1/M2) Chip - Android-Studio
- How to Know the Build Version Details of Microsoft Office 365 Applications - Microsoft