import java.util.ArrayList;
import java.util.List;
public class Example {
public static void main(String[] args) {
List<String> stringList = new ArrayList<>();
stringList.add("Java");
stringList.add("Example");
stringList.add("Text");
stringList.add(null);
stringList.forEach(str -> System.out.println(str.substring(1))); //error
}
}
In the above example as you can see I am iterating through a ArrayList of Strings that have a null value as well, so when I use forEach loop over it and iterate and do a subString on the array elements, I will get an NPE (Null Pointer Exception)
Output:Exception in thread "main" java.lang.NullPointerException: Cannot invoke "String.substring(int)" because "str" is null
at Example.lambda$main$0(Example.java:14)
at java.base/java.util.ArrayList.forEach(ArrayList.java:1511)
at Example.main(Example.java:14)
So as a developer you would need to handle the Unchecked NPE!
import java.util.ArrayList;
import java.util.List;
public class Example {
public static void main(String[] args) {
List<String> stringList = new ArrayList<>();
stringList.add("Java");
stringList.add("Example");
stringList.add("Text");
stringList.add(null);
stringList.forEach(str -> {
if(str == null) {
System.out.println("NULL Value");
} else {
System.out.println(str.substring(1));
}
});
}
}
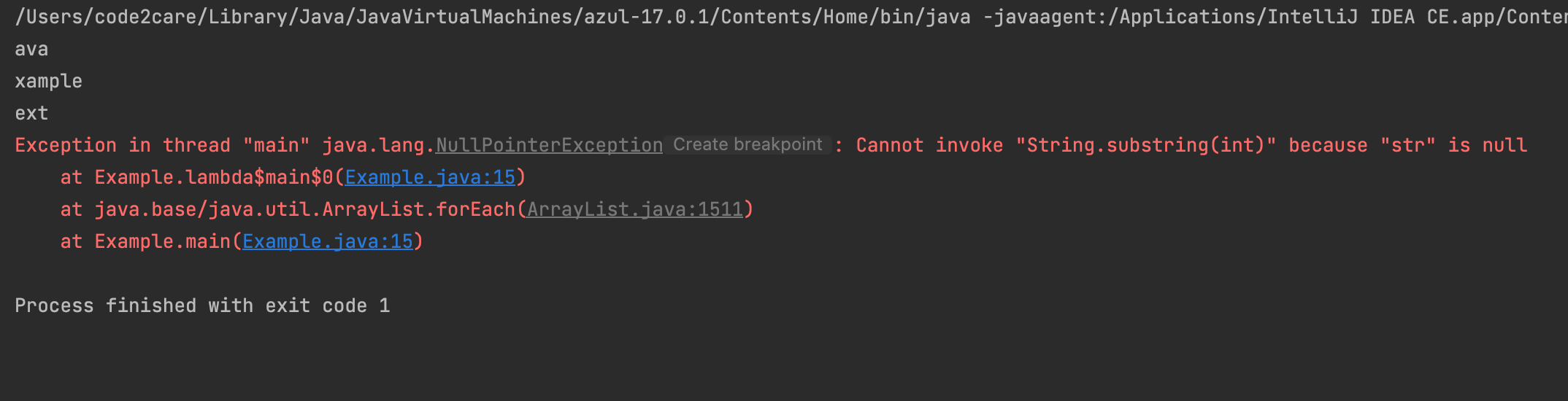
Java 8 Exception - cannot invoke because the object is null
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to Download Jira App for Mac - Jira
- Bash Command to Base64 Decode a String - Bash
- Parse XML file in Java using DOM Parser - Java
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python] - Python
- Android Studio : Change FAB icon color : FloatingActionButton - Android-Studio
- Get cURL command from Chrome Inspect Network HTTP URL - cURL
- Ways to Open New Tabs or New Windows on Windows 11 Notepad - Windows-11
- 20 - Python - Print Colors for Text in Terminal - 1000+ Python Programs - Python-Programs