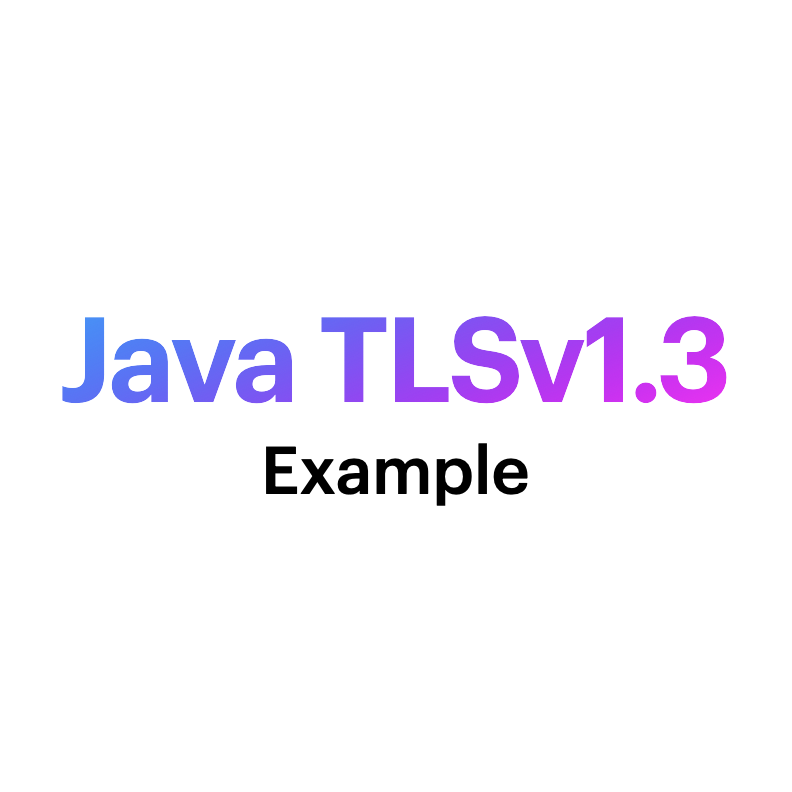
βοΈOracle Java added support to TLS v1.3 in 8u261-b12 update!
Java has support to TLSv1.3 protocol for Java JDK 1.8 and well supported by JDK 11 and later, this example demonstrates how you can connect to server (http address) using SSLSocket using TLSv1.3 protocol and TLS_AES_128_GCM_SHA256 cipher.
import javax.net.ssl.SSLSocket;
import javax.net.ssl.SSLSocketFactory;
import java.io.*;
/**
*
* Example of TLSv1.3 connection
* This code should work with
* Java JDK 8 11, 15
*
* Note: Make sure you are using
* the the JDK version that
* Supports TLSv1.3
*
*/
public class TSLv1_3Example {
private static final String host="code2care.org";
private static final int port=443;
private static final String TLSV1_3 = "TLSv1.3";
private static final String TLS_AES_128_GCM_SHA256 = "TLS_AES_128_GCM_SHA256";
private static SSLSocket sslSocket;
public static void main(String... args) throws Exception {
getSslSocket();
sendRequest();
readResponse();
}
public static void getSslSocket() throws IOException {
SSLSocketFactory sslSocketFactory = (SSLSocketFactory) SSLSocketFactory.getDefault();
sslSocket = (SSLSocket) sslSocketFactory.createSocket(host, port);
//Setting the TSLv1.3 protocol
sslSocket.setEnabledProtocols(new String[]{TLSV1_3});
//Setting the Cipher: TLS_AES_128_GCM_SHA256
sslSocket.setEnabledCipherSuites(new String[]{TLS_AES_128_GCM_SHA256});
//Handshake
sslSocket.startHandshake();
}
/**
* Read the sslSocket response
*
*/
public static void readResponse() {
try(BufferedReader in = new BufferedReader(
new InputStreamReader(
sslSocket.getInputStream()))) {
String responseData;
while ((responseData = in.readLine()) != null)
System.out.println(responseData);
} catch (IOException e) {
System.out.println("Error occurred while reading Socket Response...");
e.printStackTrace();
}
}
/**
*
* Send Request to sslSocket
*
* @throws IOException
*/
public static void sendRequest() throws IOException {
OutputStreamWriter outputStreamWriter = new OutputStreamWriter(sslSocket.getOutputStream());
PrintWriter printWriter = new PrintWriter(new BufferedWriter(outputStreamWriter));
printWriter.println("GET /test-url HTTP/1.1\r\n");
printWriter.flush();
}
}
Output:
HTTP/1.1 200 OK
Server: code2care.org
Date: Tue, 13 Apr 2021 05:43:25 GMT
Content-Type: text/html
Content-Length: 267
Connection: close
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Indent XML Formatting In Notepad++ - NotepadPlusPlus
- [fix] Spring Boot Data JPA - No identifier specified for entity - Java
- Fix: Minecraft EXCEPTION_ACCESS_VIOLATION Fatal Error Java Runtime - Java
- Android [SDK Manager] The system cannot find the path specified - Android-Studio
- How to refresh Safari on Mac (macOS) using keyboard shortcut - MacOS
- [fix] java: incompatible types: double cannot be converted to java.lang.Integer Generics - Java
- MySQL #6 - Error on delete of './my-database/db.opt' (Errcode: 13 - Permission denied) - MySQL
- Change the default login shell on macOS Ventura 13.0 - MacOS