The one thing that makes Python different then most other programming and scripting languages is its strictness towards code indentation. If the code is not well indented, your code will not execute and you will see a IndentationError.
The IndentationError exception class is the base class of syntax errors related to incorrect indentation. It is a subclass of SyntaxError.
To understand it better, one should take a look at the PEP 8 Indentation section.
As per PEP 8 instructions - "We should use 4 spaces per indentation level."
Now let's try to figure out IndentationError: unexpected indent with a simple Python code example.
Example:print("hello there")
print("how are you?")
Exception:
File "<ipython-input-16-2298b55469ab>", line 2
print("how are you?")
^
IndentationError: unexpected indent
Why unexpected indent exception?
In Python, we should add an indentation (4 spaces per indentation level per PEP 8) to indicate the beginning or ending of code blocks, such as,
For Loops
Example:
numbers = [84, 87, 76, 92, 14, 87, 98]
for number in numbers:
print(number) # 4 space indentation!
While Loops
Example:
numbers = [84, 87, 76, 92, 14, 87, 98]
index = 0
while index < len(numbers):
print(numbers[index]) # 4 space indentation! for the block!
index += 1
If-Else Conditional Statements
Example:
numbers = [84, 87, 76, 92, 14, 87, 98]
for number in numbers:
if number % 2 == 0:
print(f"{number} is even")
else:
print(f"{number} is odd")
- Function definition
Example:
def print_even_odd(numbers):
for number in numbers:
if number % 2 == 0:
print(f"{number} is even")
else:
print(f"{number} is odd")
numbers = [84, 87, 76, 92, 14, 87, 98]
print_even_odd(numbers)
Try-Except Blocks
Example:
def print_even_odd(numbers):
try:
for number in numbers:
if number % 2 == 0:
print(f"{number} is even")
else:
print(f"{number} is odd")
except TypeError:
print("Error: The input should be a list of numbers")
numbers = [84, 87, 76, 92, 14, 87, 98]
print_even_odd(numbers)
As you would have understood, the two lines we have is neither among the above cases, thus the second line cannot have a intention, thus causing IndentationError: unexpected indent
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts: - How to word wrap in HTML - Html
- Change Max and Min Value of Android Seekbar Programmatically - Android
- How to reset eclipse layout - Android
- Syntax error, parameterized types are only available if source level is 1.5 or greater [Java] - Eclipse
- Relative Imports examples in Python 3.x - Python
- JBoss stuck loading JBAS015899: AS 7.1.1.Final Brontes starting - Java
- How to install ddtrace - Datadog tracing library for Python - Python
- Get the Size of HTTP Response using cURL Command (Content Length) - cURL
For Loops
Example:numbers = [84, 87, 76, 92, 14, 87, 98] for number in numbers: print(number) # 4 space indentation!
While Loops
Example:numbers = [84, 87, 76, 92, 14, 87, 98] index = 0 while index < len(numbers): print(numbers[index]) # 4 space indentation! for the block! index += 1
If-Else Conditional Statements
Example:numbers = [84, 87, 76, 92, 14, 87, 98] for number in numbers: if number % 2 == 0: print(f"{number} is even") else: print(f"{number} is odd")
- Function definition
Example:
def print_even_odd(numbers): for number in numbers: if number % 2 == 0: print(f"{number} is even") else: print(f"{number} is odd") numbers = [84, 87, 76, 92, 14, 87, 98] print_even_odd(numbers)
Try-Except Blocks
Example:def print_even_odd(numbers): try: for number in numbers: if number % 2 == 0: print(f"{number} is even") else: print(f"{number} is odd") except TypeError: print("Error: The input should be a list of numbers") numbers = [84, 87, 76, 92, 14, 87, 98] print_even_odd(numbers)
In Python, we should add an indentation (4 spaces per indentation level per PEP 8) to indicate the beginning or ending of code blocks, such as,
As you would have understood, the two lines we have is neither among the above cases, thus the second line cannot have a intention, thus causing IndentationError: unexpected indent
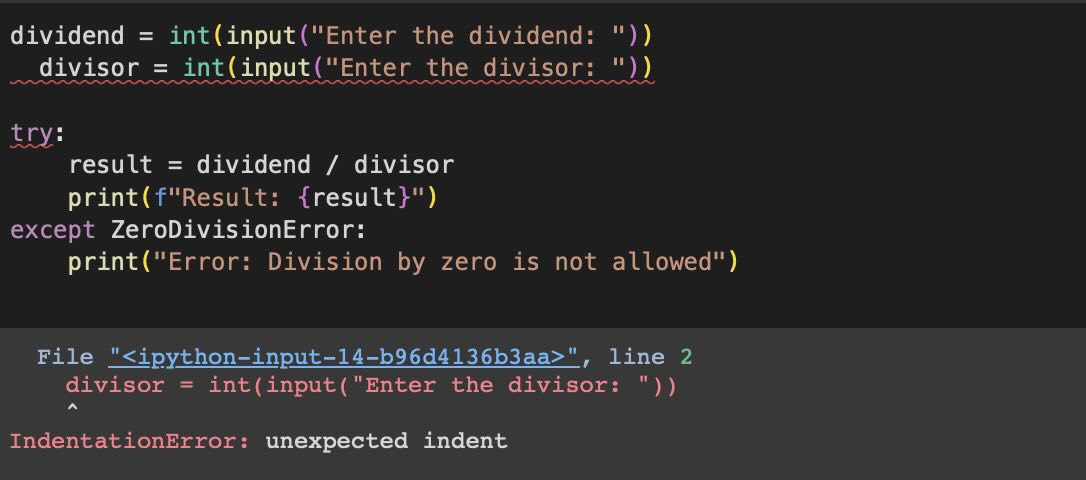
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
- How to word wrap in HTML - Html
- Change Max and Min Value of Android Seekbar Programmatically - Android
- How to reset eclipse layout - Android
- Syntax error, parameterized types are only available if source level is 1.5 or greater [Java] - Eclipse
- Relative Imports examples in Python 3.x - Python
- JBoss stuck loading JBAS015899: AS 7.1.1.Final Brontes starting - Java
- How to install ddtrace - Datadog tracing library for Python - Python
- Get the Size of HTTP Response using cURL Command (Content Length) - cURL