If you have multiple delimiters in a string and you want to split them, the best way to deal with this is using the re module in Python.
Example:import re
string_data = "part1,part2|part3,part4|part5"
split_list = re.split(r'\|', string_data)
final_list = [re.split(r',', item) for item in split_list]
print(final_list)
Output:
[['part1', 'part2'], ['part3', 'part4'], ['part5']]
In the above example we have two delimiters in the string, comma and pipe. We make use of the regular expression to first split the string based on the pipe, so we get a list of sub-strings that are now comma separated. Next, we make use of the regular expression to break these lists by commas and create a list-of-list.
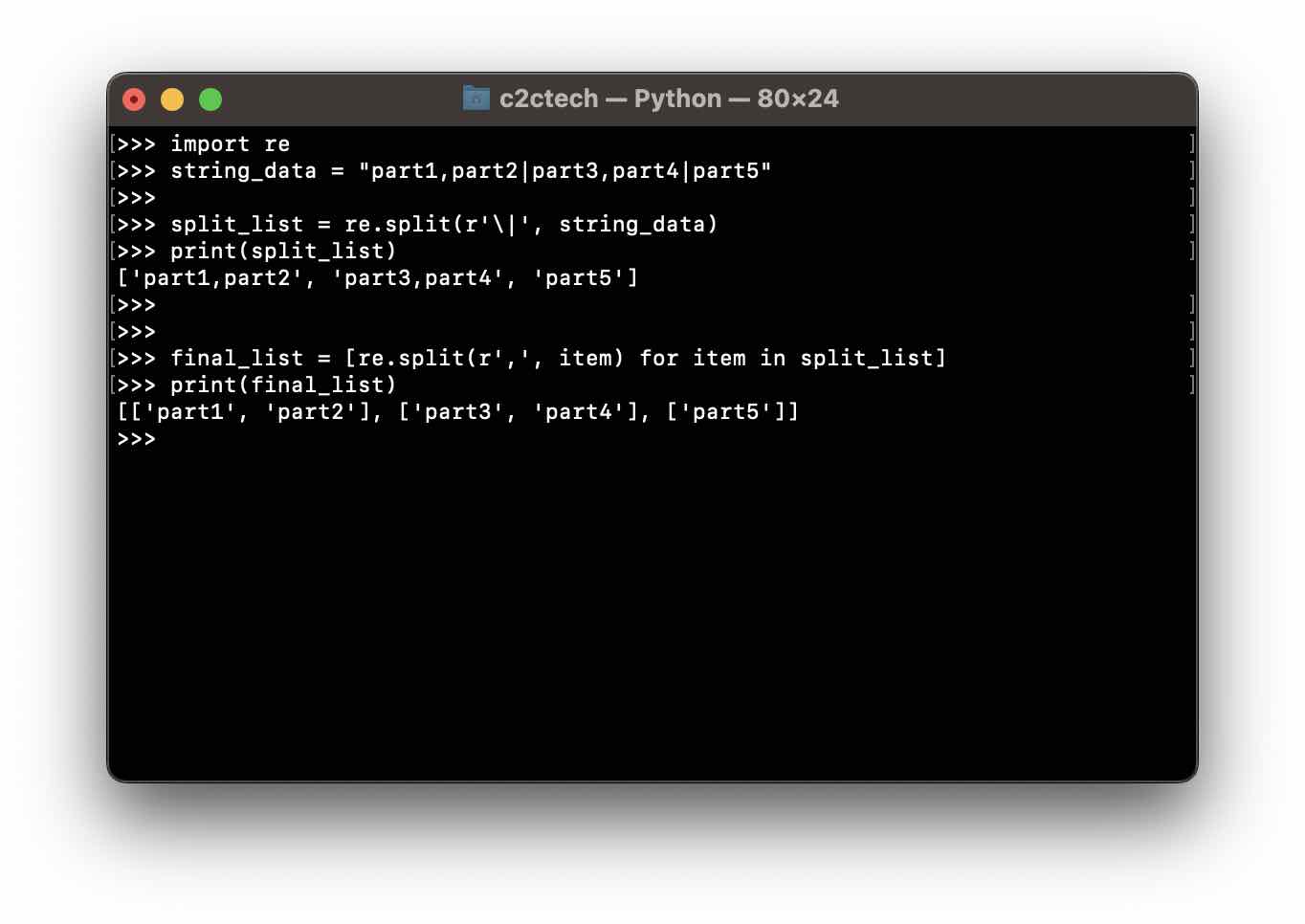
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
- Java: Check if a String contains another Sub-String with Examples - Java
- How to Create a Website (Webpage) using HTML on Windows Notepad - Windows
- Upload a File in Microsoft SharePoint using PowerShell Script - SharePoint
- How to add multiple spaces between html page text - Html
- [macOS] NetBeans IDE cannot be installed. Java (JRE) found on your computer but JDK XX or newer is required. - MacOS
- How to save a file in Nano Editor and Exit - Linux
- Facebook : Warning: Request without access token missing application ID or client token - Facebook
- Fix: ModuleNotFoundError: No module named requests - Python