If you want to make your own custom Notepad application (say as a school or college project) then the best way to get it done quickly is by using Python Programming.
In this article, we will try and create a very basic Notepad application that has the functionality as Menu options,
- Open and Edit a text file.
- Save a text file.
- Exit the Notepad
We will need to make use of the tkinter GUI module for this.
Code for Custom Notepad
import tkinter as tk
from tkinter import filedialog
def open_file():
file_path = filedialog.askopenfilename()
if file_path:
with open(file_path, 'r') as file:
text.delete(1.0, tk.END)
text.insert(tk.END, file.read())
def save_file():
file_path = filedialog.asksaveasfilename(defaultextension=".txt")
if file_path:
with open(file_path, 'w') as file:
file.write(text.get(1.0, tk.END))
root = tk.Tk()
root.title("Custom Notepad Application")
notes = tk.Text(root, wrap=tk.WORD)
notes.pack()
menu = tk.Menu(root)
root.config(menu=menu)
file_menu = tk.Menu(menu)
menu.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="Open File...", command=open_file)
file_menu.add_command(label="Save File...", command=save_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", command=root.destroy)
root.mainloop()
Demo:
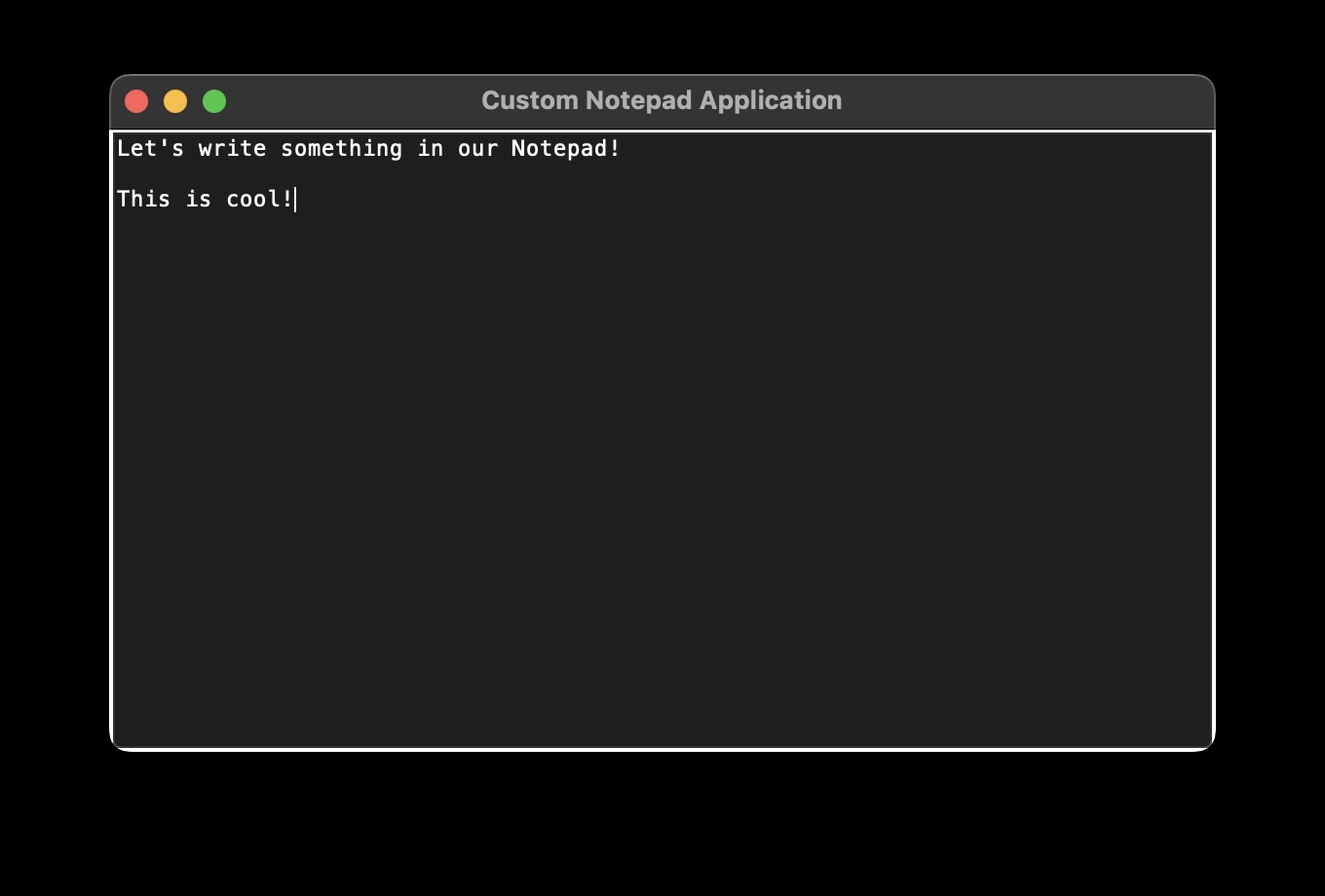
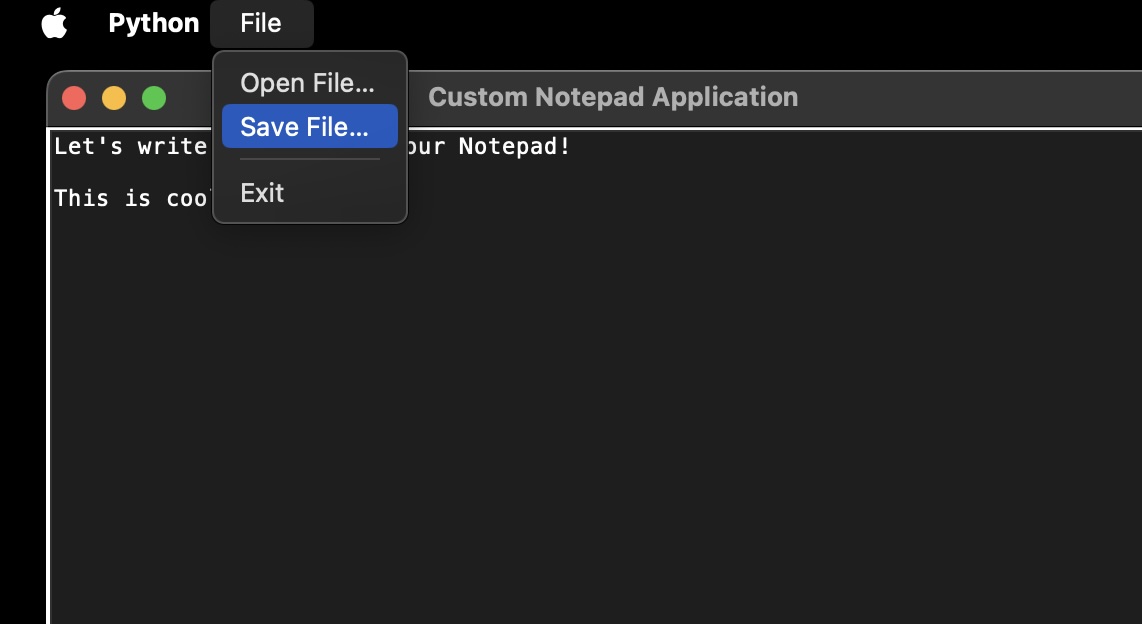
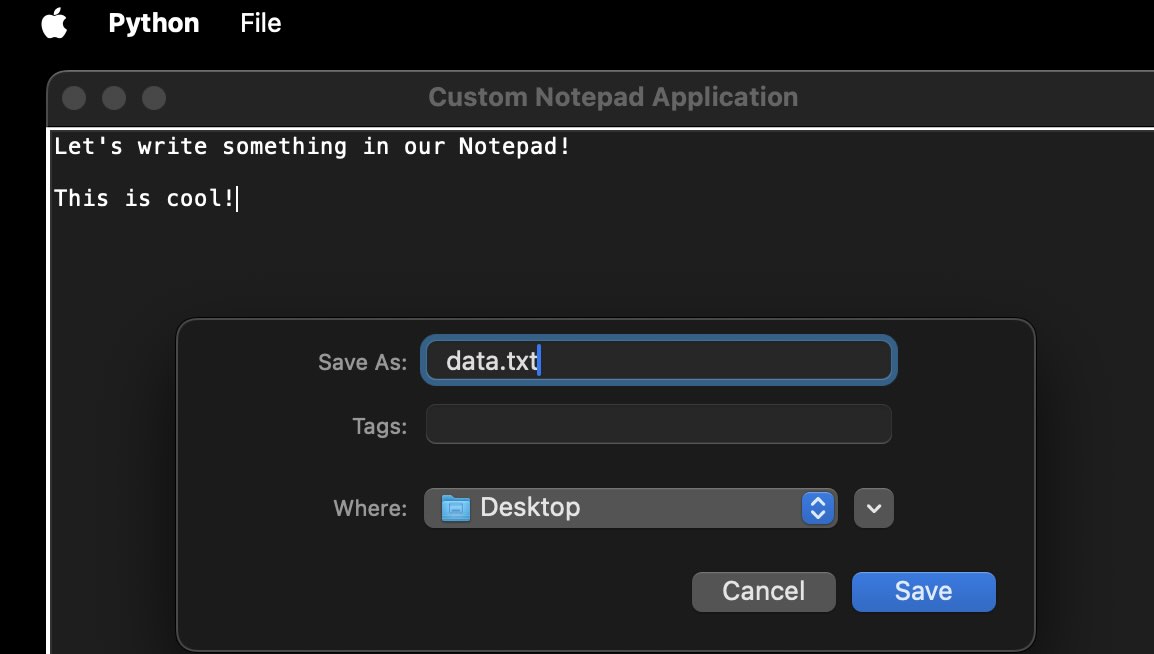
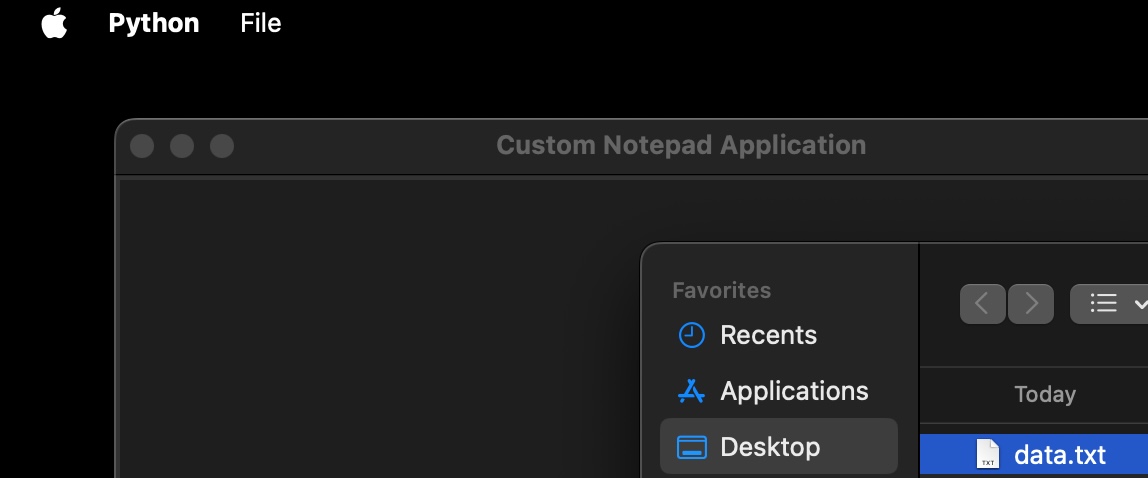
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- How to restart WiFi using Crosh Terminal (ChromeOS Chromebook) - Chrome
- How to Turn Off Assistive Access on iOS 17 - iOS
- Git Remove Untracked Files using Command - Git
- Cannot open or preview pdf with view only and restricted download access in Microsoft Teams - Teams
- Fix: NoSuchBeanDefinitionException: No bean named x available (application-config.xml) - Java
- How to Uninstall Brew on Mac - MacOS
- Wrap Text in Python using - textwrap module - Python
- 45: Take two strings and concatenate them. [1000+ Python Programs] - Python-Programs