There are two modules os and pathlib you can make use of to delete a file using Python code, let us see some examples,
Example 1: Using the os module
import os
file = "/Users/code2care/PycharmProjects/pythonProject/sample.txt"
if os.path.exists(file):
os.remove(file)
print("File: " + file + " deleted ...")
else:
print("File not found: " + file + " ...")
Example 2: Using the pathlib module
import pathlib
fileStr = "/Users/code2care/PycharmProjects/pythonProject/sample.txt"
file = pathlib.Path(fileStr)
if file.exists():
file.unlink()
print("File: " + fileStr + " deleted ...")
else:
print("File not found: " + fileStr + " ...")
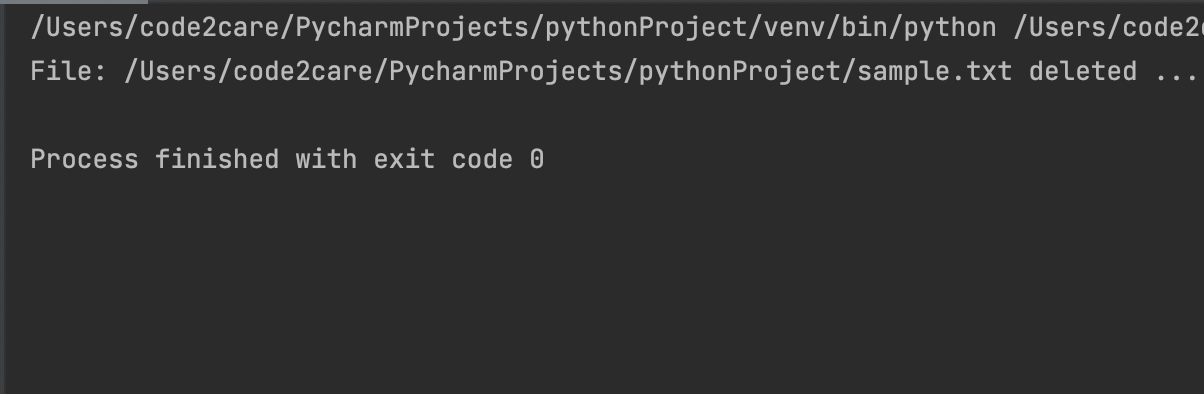
Output - Delete file using Python code
Note if you do not handle the code to look if the file exists or not you will get a FileNotFoundError,
Error:Traceback (most recent call last):
File "/Users/c2c/PycharmProjects/pythonProject/main.py", line 5, in <module>
file.unlink()
File "/Library/Developer/CommandLineTools/Library/Frameworks/Python3.framework/Versions/3.9/lib/python3.9/pathlib.py", line 1344, in unlink
self._accessor.unlink(self)
FileNotFoundError: [Errno 2] No such file or directory: '/Users/c2c/PycharmProjects/pythonProject/sample.txt'
References:
- https://docs.python.org/3/library/os.html
- https://docs.python.org/3/library/pathlib.html
- https://docs.python.org/3/library/exceptions.html
- https://docs.python.org/3/library/os.path.html?highlight=path#module-os.path
- https://docs.python.org/3/library/os.html?highlight=unlink#os.unlink
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- Android Constant and Resource Type Mismatches Lint - Android
- How to Know Which Python Version Installed on Jupyter Notebook - Python
- How to Schedule Mails in macOS Ventura - MacOS
- How to Uninstall Microsoft Teams on Mac - Teams
- PowerShell ps1 script is not digitally signed, you cannot run this script on the current system - Powershell
- Java Generics Methods Examples - Java
- How to install Python 2.7.xx on macOS 12.3 Monterey or higher - Python
- How to make TextView Text Transparent [Android] - Android