We need to make use of the datetime module methods such as combine, fromisoformat to convert a date to datetime in Python Programming.
Example 1: Using Python 2.x
import datetime
date = datetime.date(2023, 7, 23)
datetime_obj = datetime.datetime.combine(date, datetime.time())
print(datetime_obj)
Output:
Example 2: Using Python 3.x (using fromisoformat())
import datetime
date = datetime.date(2023, 7, 24)
date_str = str(date)
datetime_obj = datetime.datetime.fromisoformat(date_str)
print(datetime_obj)
Output:
Example 3: Using Python 3.x (using combine())
import datetime
date = datetime.date(2023, 7, 25)
datetime_obj = datetime.datetime.combine(date, datetime.time())
print(datetime_obj)
Output:
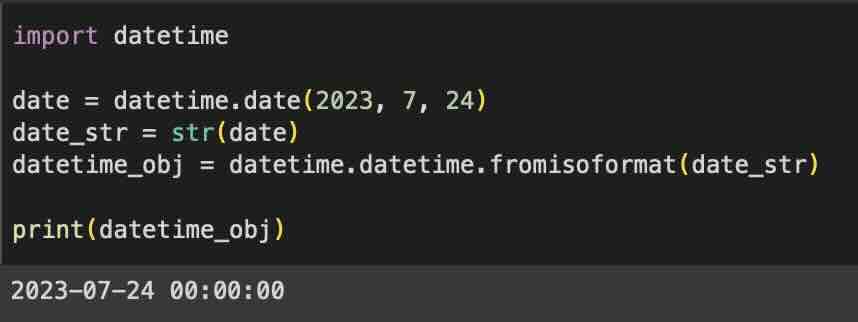
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- How to restart WiFi using Crosh Terminal (ChromeOS Chromebook) - Chrome
- How to Turn Off Assistive Access on iOS 17 - iOS
- Git Remove Untracked Files using Command - Git
- Cannot open or preview pdf with view only and restricted download access in Microsoft Teams - Teams
- Fix: NoSuchBeanDefinitionException: No bean named x available (application-config.xml) - Java
- How to Uninstall Brew on Mac - MacOS
- Wrap Text in Python using - textwrap module - Python
- 45: Take two strings and concatenate them. [1000+ Python Programs] - Python-Programs