If you are working with Spring Boot with a Spring Config file (application-config.xml) you may come across NoSuchBeanDefinitionException when you create an object of a bean in your code using the ApplicationContext object getBean() method but the bean is not defined in the Spring config file.
Example:package org.code2care.demo;
public class Employee {
private int empId;
private String empName;
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean name="department" class="org.code2care.demo.Department"></bean>
</beans>
package org.code2care.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("application-config.xml");
Employee employee = applicationContext.getBean("employee", Employee.class);
}
}
Stacktrace:
Exception in thread "main" org.springframework.beans.factory.NoSuchBeanDefinitionException:
No bean named 'employee' available
at org.springframework.beans.factory.support.DefaultListableBeanFactory.getBeanDefinition(DefaultListableBeanFactory.java:895)
at org.springframework.beans.factory.support.AbstractBeanFactory.getMergedLocalBeanDefinition(AbstractBeanFactory.java:1319)
at org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean(AbstractBeanFactory.java:299)
at org.springframework.beans.factory.support.AbstractBeanFactory.getBean(AbstractBeanFactory.java:204)
at org.springframework.context.support.AbstractApplicationContext.getBean(AbstractApplicationContext.java:1237)
at org.code2care.demo.DemoApplication.main(DemoApplication.java:15)
As you can see I have not defined the bean tag under the application-config.xml file for the class employee, to fix this issue, I will need to create it as well.
<bean name="employee" class="org.code2care.demo.Employee"></bean>
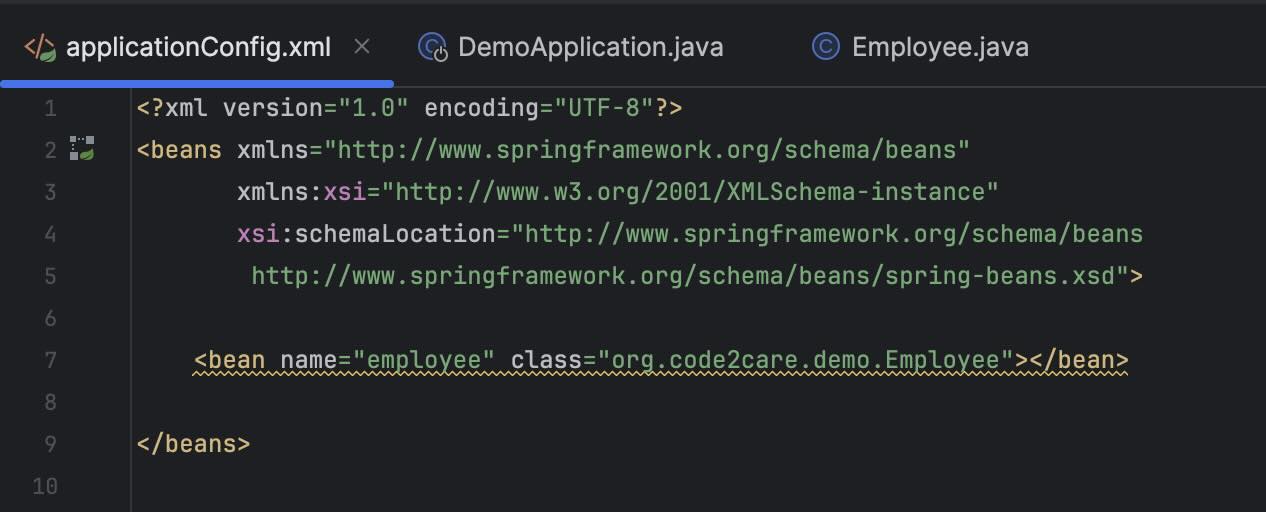
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to make Text in TextView bold and italic in Android - Android
- TextEdit Find and Replace Example - MacOS
- Top 10 emerging breakthrough trending technologies - HowTos
- [Fix] brew: command not found Mac or Linux Terminal Error - MacOS
- Keyboard Shortcut to Hide Dock on Mac - MacOS
- Install Golang (Go) on Ubuntu - Ubuntu
- 97 Useful Notepad++ Keyboard Shortcuts - NotepadPlusPlus
- How choose alternate Tab Bar icon in Notepad++ - NotepadPlusPlus