Like we have NUL, NULL, or null in other programming languages, in Python we have None which is used to represent the absence of a value.
In order to check if a particular object is None or not, we can make use of the If condition as below,
-
Syntax 1:
if variable is not None:
Syntax 2:
def function(object):
if object is not None:
# Your code Logic
else:
# Your code Logic
It is always better to look at the PEP (Python Enhancement Proposals) Guides to know what they say.
As per PEP-8
# Correct:
if foo is not None:
# Wrong:
if not foo is None:
Read More: Programming Recommendations
Now let's take a look at a few examples.
def check_for_none(object):
if object is not None:
print(f"The object is: {object}")
else:
print("The object is None!")
check_for_none(42)
check_for_none("Hello")
check_for_none(None)
Output:
The object is: 42
The object is: Hello
The object is None!
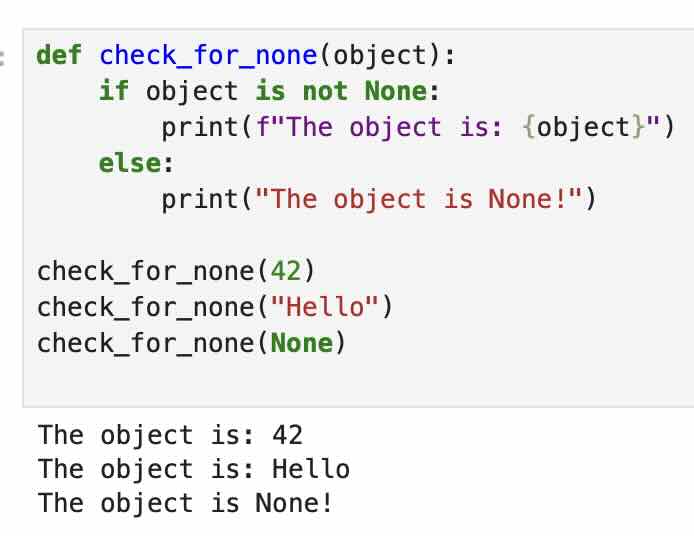
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- Save Java Object as JSON file using Jackson Library - Java
- [Soluiton] You already have the latest version of Android Studio installed - Android
- How to disable button in Bootstrap - Bootstrap
- How to Install CVS Version Control on Linux/Ubuntu - Linux
- How to write JSON file in Python Program - Python
- How to add maven central repository in build.gradle - Gradle
- Android appcompat_v7 Error retrieving parent for item: No resource found that matches the given name - Android
- Maven Central Repository: URL and Details - Java