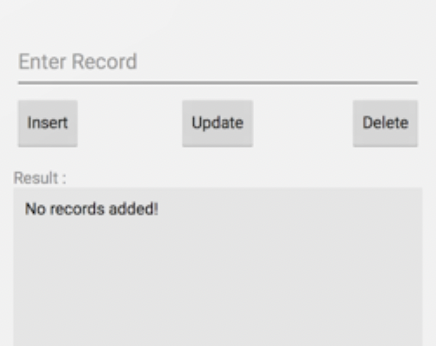
Room in Android App development is a persistence library (just like an ORM tool JPA/Hibernate) that provides an abstraction layer over SQLite Database, thus this give you a benefit of an easy seamless database access without compromising the the full power of SQLite. It is recommended to make use of the Room instead of directly using SQLite because of the below reasons,
- Do compile time verification of your SQL queries.
- Streamline the migration path of your database.
- Make use of annotations that help to reduce boilerplate code and thus errors resulting from it.
- Easy seamless database access without compromising the the full power of SQLite
Tutorial: Setting up Room in Android Project
- Setting up Gradle: Add the dependency in build.gradle,
dependencies { .... implementation "androidx.room:room-runtime:2.4.1" annotationProcessor "androidx.room:room-compiler:2.4.1" .... }
- Create the Entity classes,
@Entity public class Employee { @PrimaryKey public int emp_id; @ColumnInfo(name = "emp_name") public String empName; @ColumnInfo(name = "emp_dept") public String empDept; @ColumnInfo(name = "emp_sal") public Double empSal; }
- Creating the DAO layer,
@Dao public interface EmployeeDao { @Query("SELECT * FROM employee") List< Employee > getAll(); @Query("SELECT * FROM employee WHERE emp_id IN (:empIds)") List<Employee> loadAllByEmpIds(int[] empIds); @Query("SELECT * FROM employee WHERE emp_name LIKE :empName LIMIT 1") Employee findByEmpName(String firstName); @Insert void insertAll(Employee... employee); @Delete void delete(Employee employee); }
Create the app database: You must extend RoomDatabase and annotate the class with @Database
@Database(entities = {Employee.class}, version = 1) public abstract class AppDatabase extends RoomDatabase { public abstract EmployeeDao employeeDao(); }
How to use The Room Database in your Android Application
Now that we have set the DAO, DAO implementation and the Database, lets use it in your Intents,
AppDatabase appDb = Room.databaseBuilder(getApplicationContext(),
AppDatabase.class, "database-name").build();
EmployeeDao empDao = db.empDao();
Employee empDetails = findByEmpName("Sam");
List<Employee> employees = empDao.getAll();
More Posts related to Android,
- Increase Android Emulator Timeout time
- Android : Remove ListView Separator/divider programmatically or using xml property
- Error : Invalid key hash.The key hash does not match any stored key hashes
- How to Change Android Toast Position?
- Android Alert Dialog with Checkboxes example
- Android : No Launcher activity found! Error
- Android Development: Spinners with Example
- Failed to sync Gradle project Error:failed to find target android-23
- INSTALL_FAILED_INSUFFICIENT_STORAGE Android Error
- Disable Fading Edges Scroll Effect Android Views
- How to create Toast messages in Android?
- Channel 50 SMSes received every few minutes Android Phones
- Android xml error Attribute is missing the Android namespace prefix [Solution]
- Create Custom Android AlertDialog
- How To Disable Landscape Mode in Android Application
- Android Development - How to switch between two Activities
- incorrect line ending: found carriage return (\r) without corresponding newline (\n)
- Generate Facebook Android SDK keyhash using java code
- Android Error Generating Final Archive - Debug Certificate Expired
- 21 Useful Android Emulator Short-cut Keyboard Keys
- Android RatingBar Example
- 11 Weeks of Android Online Sessions-15-Jun-to-28-Aug-2020
- Download interrupted: Unknown Host dl-ssl.google.com Error Android SDK Manager
- fill_parent vs match_parent vs wrap_content
- Android : Connection with adb was interrupted 0 attempts have been made to reconnect
More Posts:
- Quickly install VS Code on macOS Sonoma/Ventura - MacOS
- Read file from Windows CMD (Command Line) - Windows
- Health Status Page for OpenAI ChatGPT or GPT 4 - HowTos
- Share Image to WhatsApp with Caption from your Android App - WhatsApp
- PowerShell Alias Type Commands List for Mac - MacOS
- Get the Complete Sha256 Container ID for Docker Run Command - Docker
- Tesla hit by a complete network and mobile app outage - 23 Sept 2020 11am ET (US and Europe) - News
- Java: Convert Byte to Binary String Example - Java