In order to merge DataFrames with pandas in Python we can make use of the pandas.merge() function.
Example:
import pandas as pd
data_city_NYC = {
'Temp_NYC': [25, 30, 27, 25, 24, 24],
'Humidity_NYC': [50, 45, 55, 30, 55, 45]
}
data_city_Chicago = {
'Temp_Chicago': [28, 32, 29, 28, 29, 30],
'Humidity_Chicago': [60, 58, 62, 61, 65, 67]
}
dates = pd.date_range(start='2023-07-01', periods=6, freq='D')
df_city_NYC = pd.DataFrame(data_city_NYC, index=dates)
df_city_Chicago = pd.DataFrame(data_city_Chicago, index=dates)
print("DataFrame: NYC City")
print(df_city_NYC)
print("\nDataFrame: Chicago City")
print(df_city_Chicago)
merged_cities_df = pd.merge(df_city_NYC, df_city_Chicago, left_index=True, right_index=True)
print("\nMerged DataFrame Data:")
print(merged_cities_df)
Output:
DataFrame: NYC City
Temp_NYC Humidity_NYC
2023-07-01 25 50
2023-07-02 30 45
2023-07-03 27 55
2023-07-04 25 30
2023-07-05 24 55
2023-07-06 24 45
DataFrame: Chicago City
Temp_Chicago Humidity_Chicago
2023-07-01 28 60
2023-07-02 32 58
2023-07-03 29 62
2023-07-04 28 61
2023-07-05 29 65
2023-07-06 30 67
Merged DataFrame Data:
Temp_NYC Humidity_NYC Temp_Chicago Humidity_Chicago
2023-07-01 25 50 28 60
2023-07-02 30 45 32 58
2023-07-03 27 55 29 62
2023-07-04 25 30 28 61
2023-07-05 24 55 29 65
2023-07-06 24 45 30 67
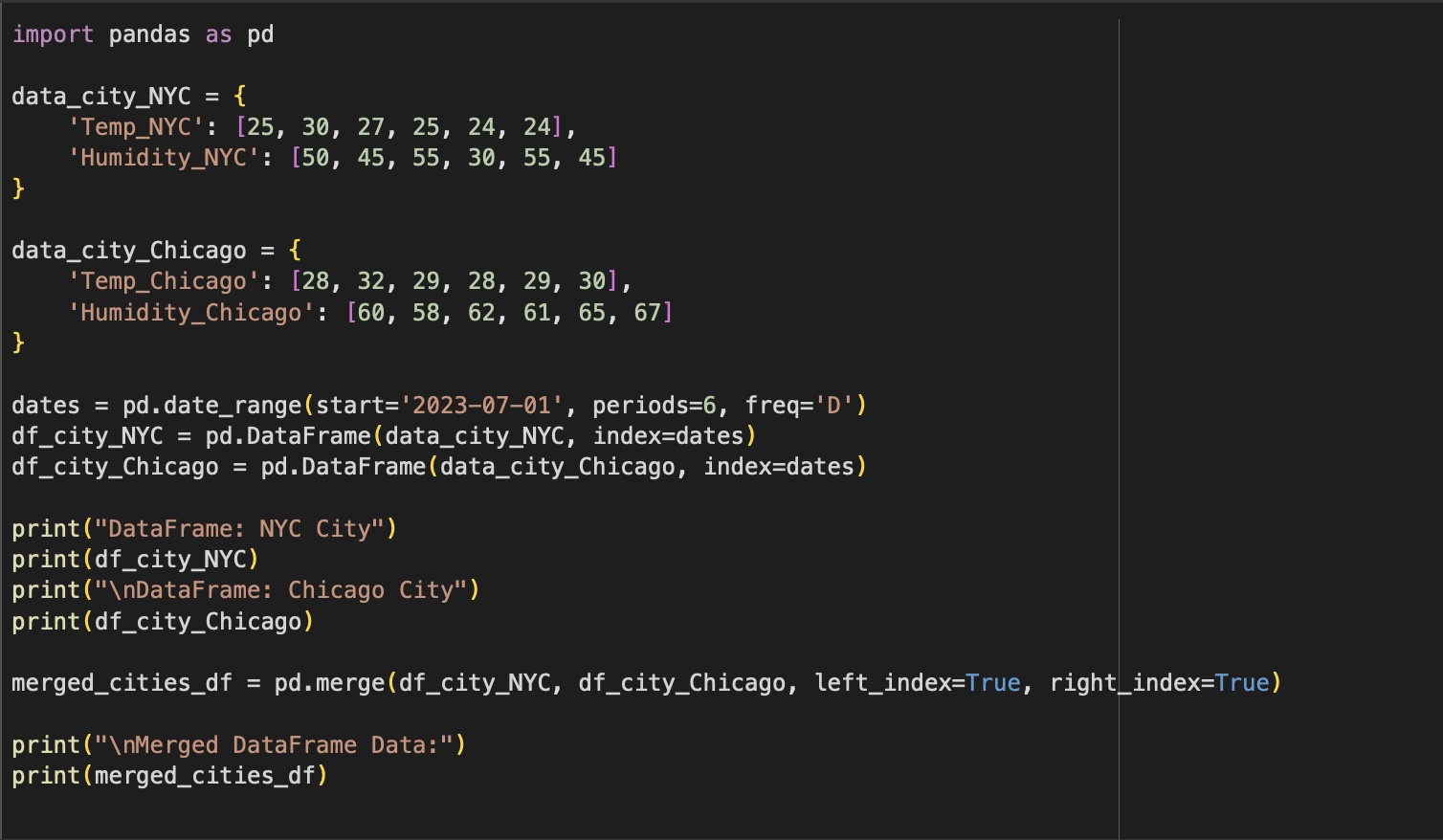
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- Android EditText Cursor Colour appears to be white - Android
- Create a Database Table using JDBC PreparedStatement - Java
- How to Write Code in Windows Notepad - Windows
- Check DNS Lookup using Mac Terminal - MacOS
- [Error] zsh: command not found: mvn - HowTos
- Command to check Last Login or Reboot History of Users and TTYs - Linux
- Microsoft Teams adds New Conversation Button - Teams
- Delete Android Studio Projects - Android-Studio