If you have a statement in Python that is too long and you want to break that statement into multiple lines without breaking the indentation rule of Python, then you need to take a look at the PEP 8 which is titled "Style Guide for Python Code"
To break a line without breaking the indentation rule, you can use line continuation techniques.
Example:
Let's say we have a string with a concatenation of a few strings.
my_data = "This is some text" + " that is too long " + " and breaks readability" + " as it spans over 79 characters."+ " Lets try to fix it"
We can make use of below-line continuation techniques
Option 1: Using parentheses for line continuation
my_data = ("This is some text" +
" that is too long " +
" and breaks readability" +
" as it spans over 79 characters." +
" Let's try to fix it")
Option 2: Using backslashes for line continuation
my_data = "This is some text" + \
" that is too long " + \
" and breaks readability" + \
" as it spans over 79 characters." + \
" Let's try to fix it"
Option 3: Implicit line continuation (no plus sign needed)
my_data = "This is some text" \
" that is too long " \
" and breaks readability" \
" as it spans over 79 characters." \
" Let's try to fix it"
Example:
>>> my_data = "This is some text" \
... " that is too long " \
... " and breaks readability" \
... " as it spans over 79 characters." \
... " Let's try to fix it"
>>> print(my_data)
This is some text that is too long and breaks readability as it spans over 79 characters. Let's try to fix it
>>>
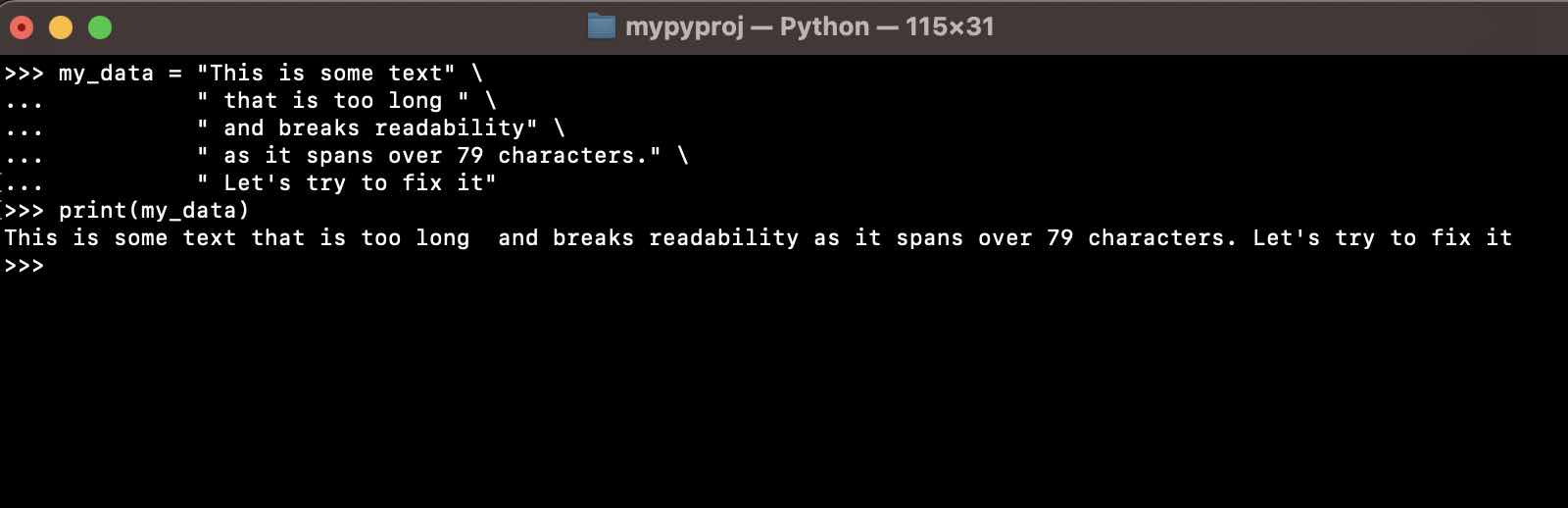
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- Docker - Error response from daemon: You cannot remove a running container - Docker
- 86 Gmail keyboard shortcuts that you may find Advantageous - Google
- Fix Microsoft Teams error We're sorryβwe have run into an issue Try again - Teams
- cURL -d Option with Examples - cURL
- Spring Boot: JdbcTemplate Update Query With Parameters Example - Java
- Share or Send SMS via Android Intent - Android
- How to detect Browser and Operating System Name and Version using JavaScript - JavaScript
- How to check if a variable is set in Bash Script or Not - Bash