If you have a String in Python and you want to convert it into a boolean value in Python, there below are a few examples of how to do that.
Example 1: Using the bool() function
true_string = "True"
false_string = "False"
true_boolean = bool(true_string)
false_boolean = bool(false_string)
print(type(true_boolean))
print(type(false_boolean))
print(true_boolean)
print(false_boolean)
Output:
<class 'bool'>
<class 'bool'>
True
False
Note that if the string is not either True or False the result will always be true.
>>> string_bool = "true"
>>> print(bool(string_bool))
True
>>>
>>> string_bool = "false"
>>> print(bool(string_bool))
True
>>>
>>> string_bool = "garbage"
>>> print(bool(string_bool))
True
>>>
Example 2: By Comparing the Strings
Its better to first validate that the strings are either true or false or 0 or 1 indicators before covering them to booleans.
def convert_to_boolean(value):
value = value.lower()
if value in ['true', '1']:
return True
elif value in ['false', '0']:
return False
else:
raise ValueError("Invalid boolean string")
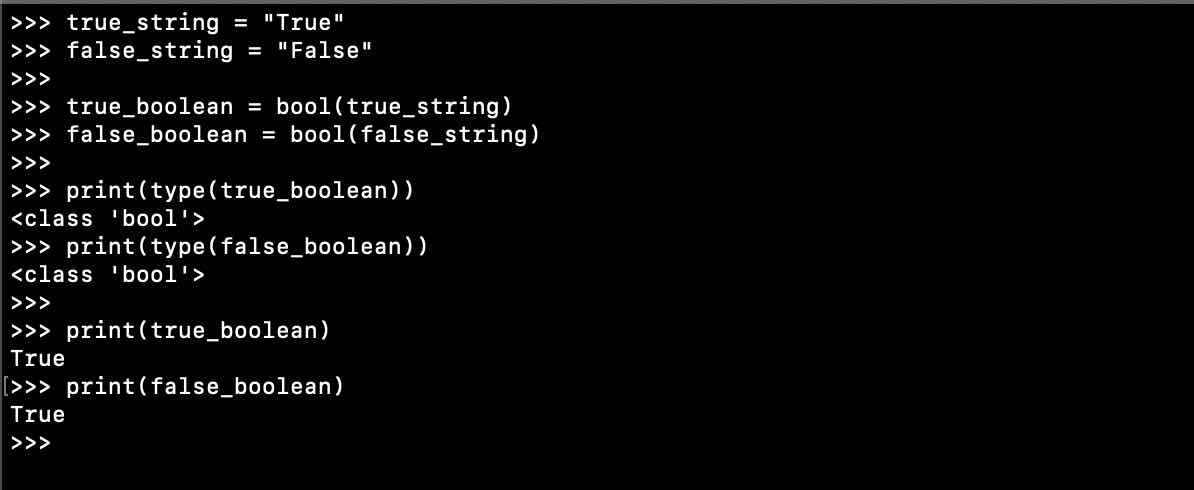
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Python,
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
More Posts:
- Select Line Number TextEdit on Mac - MacOS
- Detect if Cookies are enabled using JavaScript - JavaScript
- Lost your iPhone? Regenerate QR Code for Microsoft Authenticator App - Microsoft
- Android : Neither user 10085 nor current process has android.permission.ACCESS_NETWORK_STATE - Android
- Online Strong Random Password Generator - Tools
- Best way to Store Date of Birth in Java 8 and Above - Java
- How to Subscribe to AWS SNS Topic [SMS/Email/Lambda] via CLI - AWS
- Change Android Toast background color - Android