In order to execute cURL Command from your Python code you will need to make use of the subprocess module.
The subprocess module helps to create new processes and connect to their input/output/error pipes and get their return codes.
Example:import subprocess
result = subprocess.check_output('curl https://example.com', shell=True)
print(result.decode('utf-8'))
In the above example we have made use of the check_output method from the subprocess module with the curl command as arguments and return its output to the console.
shell=True argument tells subprocess to use a shell to execute the command.
Notes:
- Subprocess module does not work with WebAssembly, wasm32-emscripten and wasm32-wasi.
- If the return code of subprocess.check_output is non-zero it raises a CalledProcessError.
--------------------------------------------------------------------------- CalledProcessError Traceback (most recent call last) <ipython-input-4-eb1a703d34f7> in <cell line: 7>() 5 6 # Execute the curl command and capture the output ----> 7 result = subprocess.check_output(cURL_command, shell=True) 8 9 print(result.decode('utf-8')) 1 frames /usr/lib/python3.9/subprocess.py in run(input, capture_output, timeout, check, *popenargs, **kwargs) 526 retcode = process.poll() 527 if check and retcode: --> 528 raise CalledProcessError(retcode, process.args, 529 output=stdout, stderr=stderr) 530 return CompletedProcess(process.args, retcode, stdout, stderr) CalledProcessError: Command 'curl -xt https://example.com' returned non-zero exit status 5.
- Caution: Executing external commands can be a potential security risk if user input is involved, so it's important to use caution and sanitize input appropriately.
References:
- https://docs.python.org/3/library/subprocess.html
- https://docs.python.org/3/library/subprocess.html#subprocess.check_output
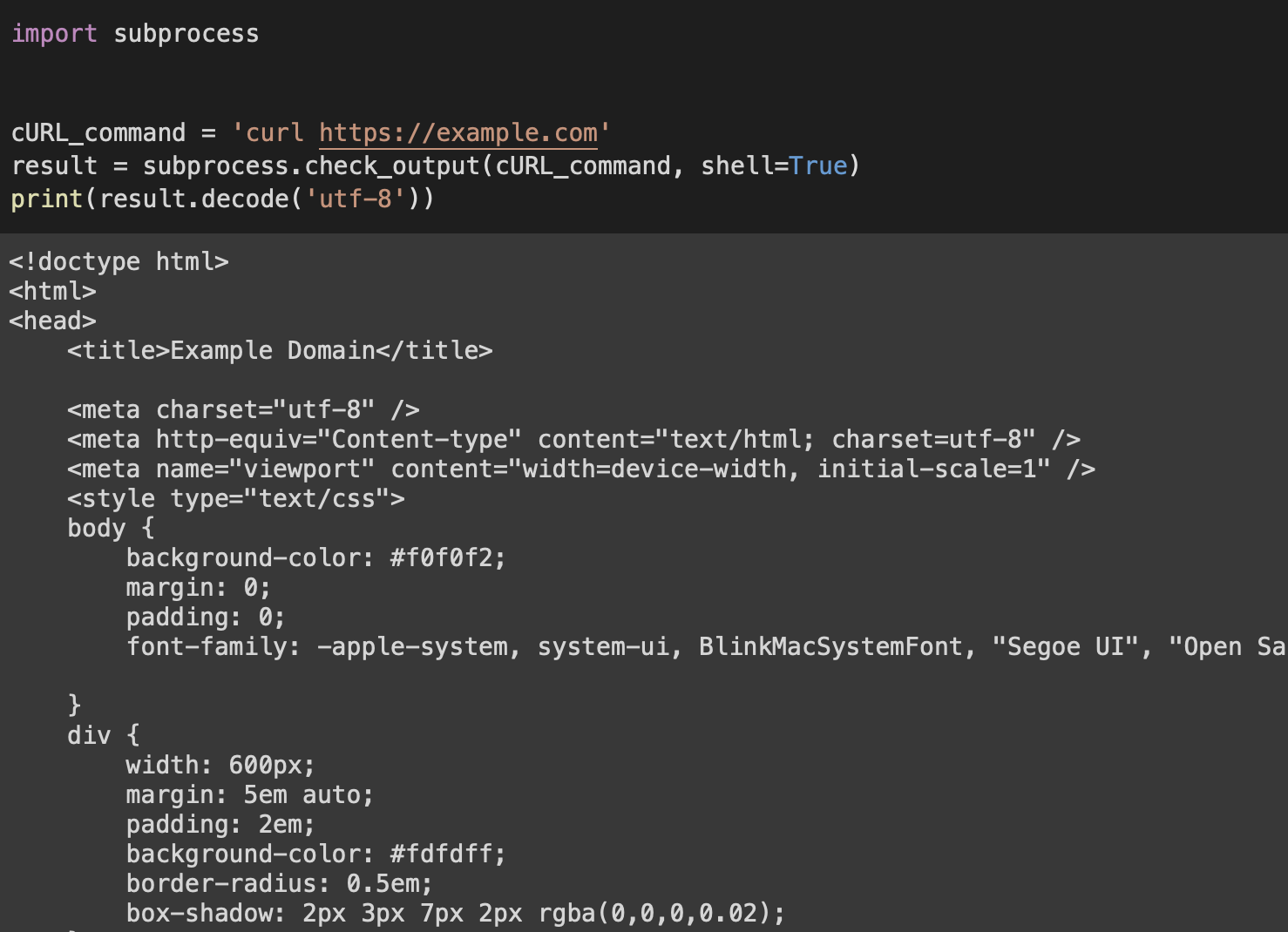
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- How to convert int to ASCII in Python
- How to make use of SQLite Module in Python?
- Split a String into Sub-string and Parse in Python
- Python: Pandas Rename Columns with List Example
- How to run Python file from Mac Terminal
- How to Exit a Loop in Python Code
- Python: How to Plot a Histogram using Matplotlib and data as list
- MD5 Hashing in Python
- Jupyter: Safari Cant Connect to the Server localhost:8888/tree
- Fix: AttributeError: str object has no attribute decode. Did you mean: encode?[Python]
- How to Read a binary File with Python
- How to add two float numbers in Python
- Python: How to install YAML Package
- Python: How to Save Image from URL
- What is Markdown in Jupyter Notebook with Examples
- How to change the Python Default version
- 33: Python Program to send an email vid GMail
- How to comment code in Python
- How to Fix AttributeError in Python
- Fix: error: Jupyter command `jupyter-nbconvert` not found [VSCode]
- How to comment out a block of code in Python
- List of All 35 Reserved Keywords in Python Programming Language 3.11
- Import Other Python Files Examples
- Python: How to add Progress Bar in Console with Examples
- 3 Ways to convert bytes to String in Python
- Display (Show) bookmarks bar Safari - HowTos
- How to activate and use Microsoft Teams Together mode - Microsoft
- Github: fatal: Authentication failed Support for password was removed on August 13, 2021 - Git
- Change label (text) color in tkinter - Python
- Notepad++ do not show CRLF characters - NotepadPlusPlus
- How to remove blank lines from a file using Notepad++ - NotepadPlusPlus
- Get the current timestamp in Java - Java
- Word wrap text in Notepad++ - NotepadPlusPlus