1. Using parseInt() function:
The parseInt() function parses a string argument and returns an integer.
Example 1: String to int
console.log(parseInt('100'));
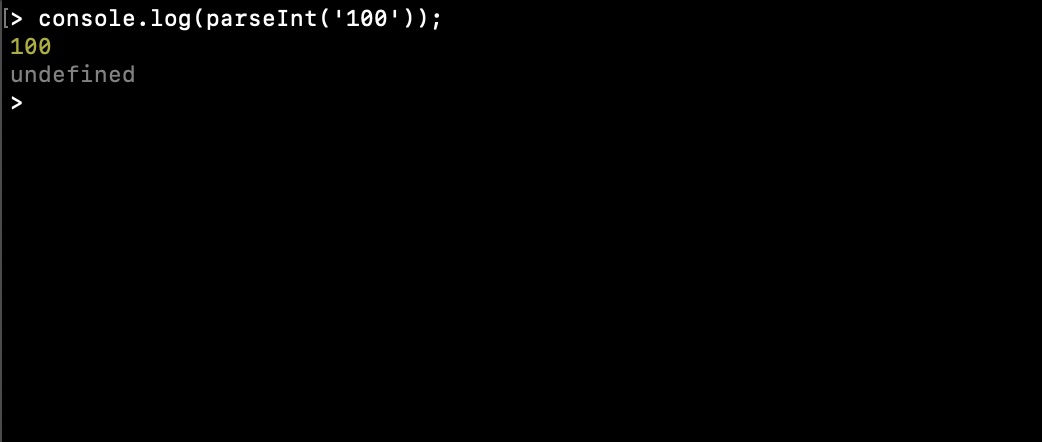
Example 2: String to int with base 2
console.log(parseInt('200', 2));
Example 3: White-spaces are ignored.
console.log(parseInt(' 300 '));
Example 4: Leading zero's are ignored.
console.log(parseInt('000400'));
Example 5: Decimal's are ignored.
console.log(parseInt('500.678'));
If the String value provided is not a valid number, then we get NaN as an output.
Example 6: NaN output for Invalid String Number.
console.log(parseInt('hello'));
Summary: parseInt()
- It accepts an optional second argument (radix) that specifies the base of the number.
- Leading whitespace is ignored.
- Leading zeros are ignored.
- Decimals are ignored..
- If the string starts with '0x' or '0X', it's interpreted as a hexadecimal number.
- If the string doesn't start with a valid numeric character, parseInt() returns NaN.
- If the radix is not specified or is 0, JavaScript assumes base 10.
- If the radix is not in the range 2-36 or is not a number, parseInt() returns NaN.
- Negative numbers can be parsed by including a '-' sign at the beginning.
- If the string contains non-numeric characters after valid numeric characters, parseInt() stops parsing at the non-numeric character.
- If the string is empty or contains only non-numeric characters, parseInt() returns NaN
2. Using parseFloat() function
The parseFloat() function parses a string argument and returns a floating point number.
Example 7:
console.log(parseFloat('600'));
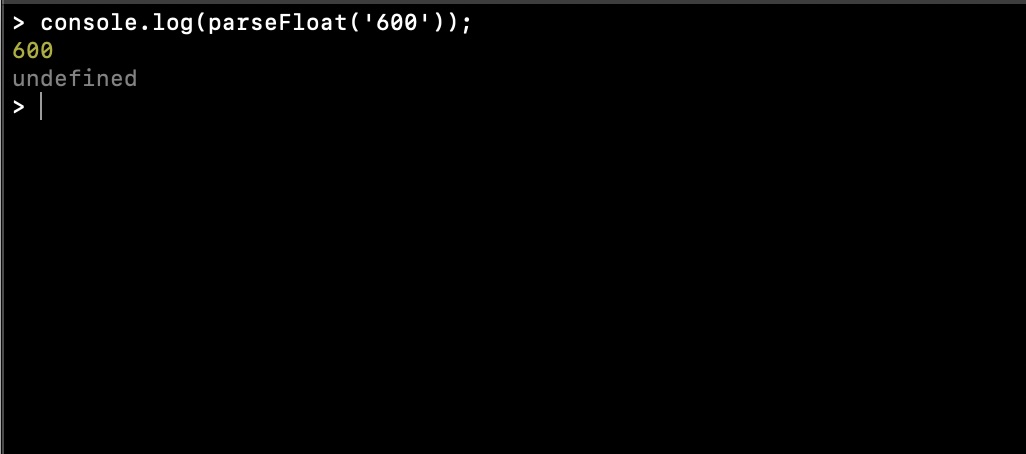
Example 8:
console.log(parseFloat('700.89'));
Example 9:
console.log(parseFloat(' 800.1 '));
Example 10:
console.log(parseFloat('000900.1'));
Example 11:
console.log(parseFloat('Code2care'));
3. Using Number() Constructor
Example 12:
console.log(Number('1200'));
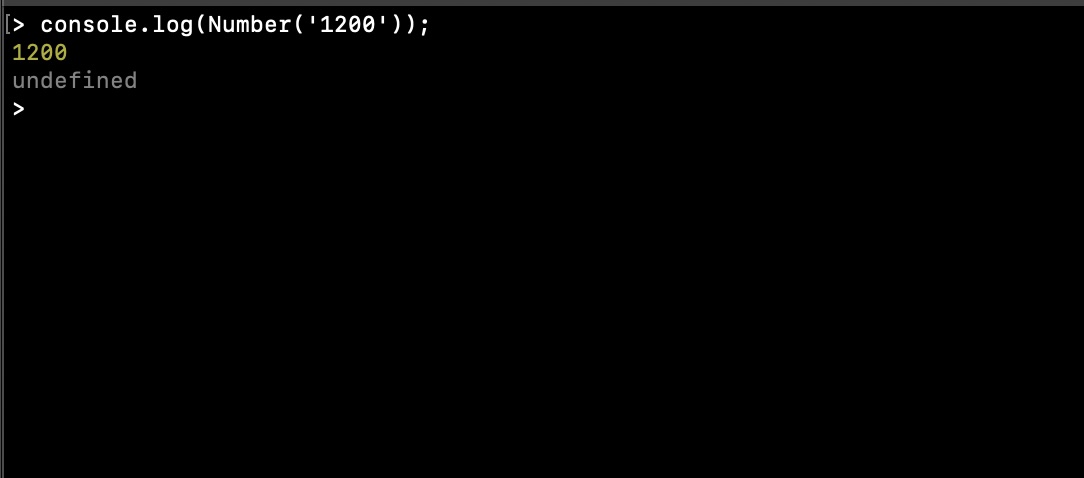
4. Using + Operator
Example 13:
console.log(+'1300');
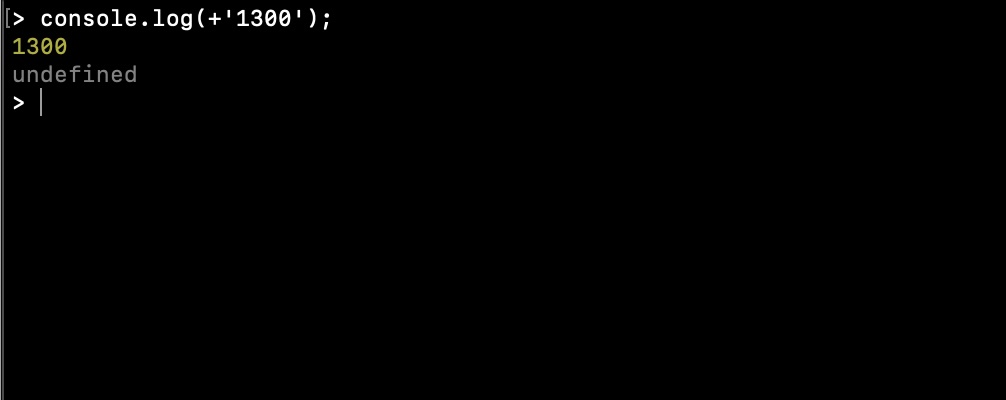
5. Math.floor() function (truncates decimal)
Example 14:
console.log(Math.floor('1400.2'));
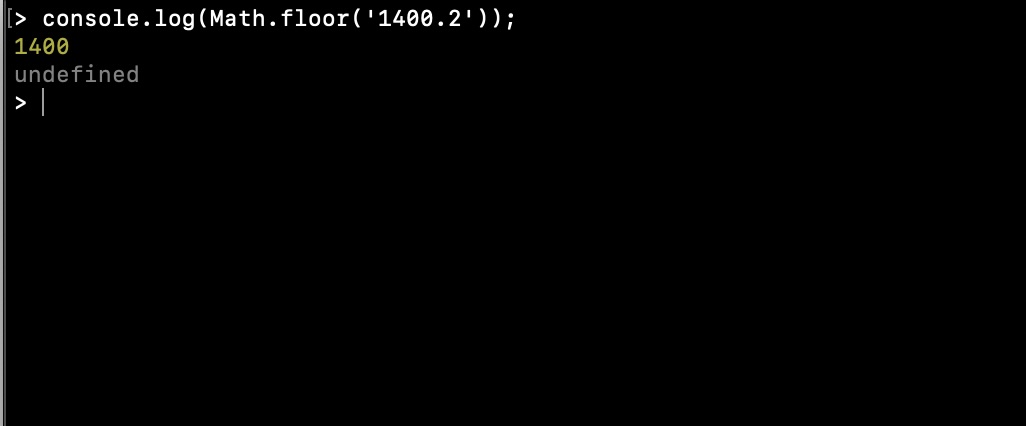
6. Math.round() function (rounds up/down decimals)
Example 15:
console.log(Math.round('1500.7'));
console.log(Math.round('1500.2'));
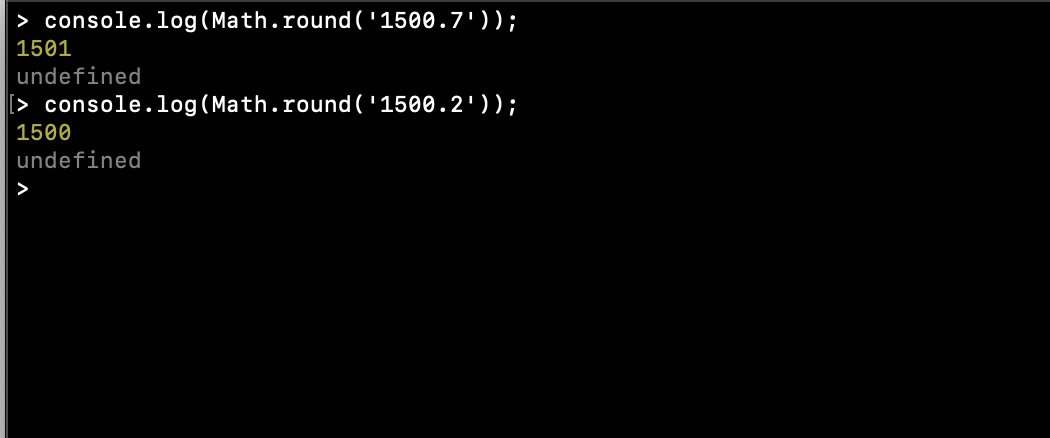
7. Math.ceil() function (rounds up decimals)
Example 16:
console.log(Math.ceil('1600.2'));
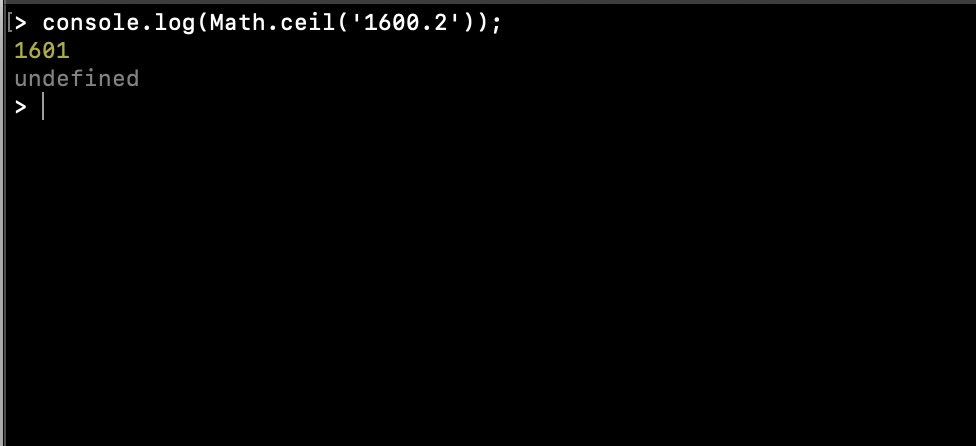
8. BigInt() Constructor
Example 17:
console.log(String(BigInt('238923820340230470237')));
9. Bitwise OR | Operator
Example 18:
console.log("1800" | 0);
10. Implicit Type Conversion
Example 19:
When we do arithmetic operation with a String and a Number, JavaScript automatically converts strings into a number.
console.log("19"*100);
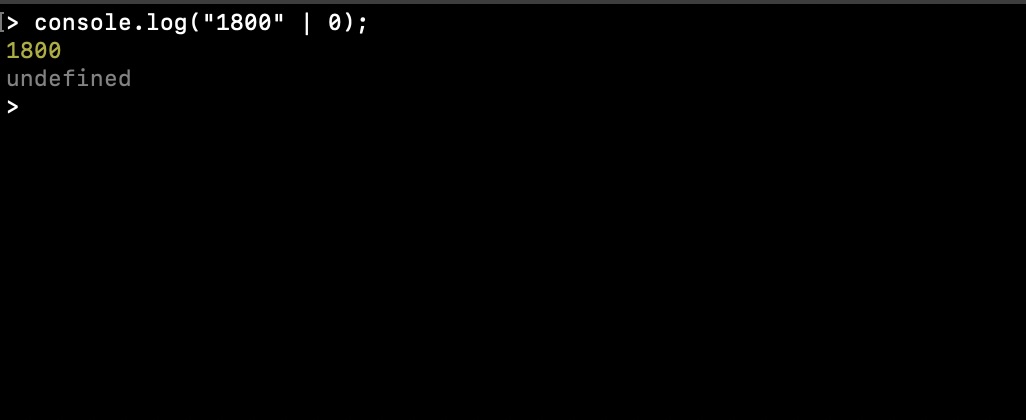
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- How to Run JavaScript on Mac Terminal
- Get Current time in GMT/UTC using JavaScript
- How to yarn reinstall all Packages
- [javaScript] Convert text case to lowercase
- Get Device Screen Width and Height using javaScript
- Fix - npm start: sh: index.js: command not found
- npm WARN saveError ENOENT: no such file or directory, open /mnt/c/package.json
- JavaScript : Get url protocol HTTP, HTTPS, FILE or FTP
- JavaScript: Convert an Image into Base64 String
- JavaScript : Get current page address
- How to get query string in JavaScript HTML location.search
- Create React App using npm command with TypeScript
- JavaScript: Count Words in a String
- Add Animated Scrolling to Html Page Title Script
- How to send email from JavaScript HTML using mailto
- Javascript convert text case from uppercase to lowercase
- Submit html form on dropdown menu value selection or change using javascript
- Send Extra Data with Ajax Get or Post Request
- Fix: SyntaxError: The requested module does not provide an export named default
- Examples: Convert String to int in JavaScript
- 10 ways to Convert String to a Number in JavaScript
- Excel Fix: SECURITY RISK Microsoft has blocked macros from running because the source of this file is untrusted.
- Fix: ReferenceError: require is not defined in ES module scope [Node]
- [JavaScript] Remove all Newlines From String
- How to detect Browser and Operating System Name and Version using JavaScript
- How to Calculate Age from Date of Birth in Java - Java
- How to create a Symbolic Link in Linux (ln -s) - Linux
- MongoDB: Failed to connect to 127.0.0.1:27017 reason: Connection refused - HowTos
- Change battery percentage in Android Emulator - Android
- Add Syntax Highlighting in Zsh Shell - zsh
- How to Save and Exit a File in Nano [macOS/Linux/Ubuntu] Terminal - Linux
- Google Celebrates Rosa Bonheur 200th birthday with a Google Doodle - Google
- How to install iTerm2 - Terminal alternative for Mac - MacOS