If you have a String object in JavaScript and you want to know the number of words it has, you can achieve this in various ways, lets see some of the code examples.
Example 1: Reading textarea data and finding the number of words in it.
<textarea id="myData" onBlur="getWordCount();"></textarea>
<div id="count"></div>
<script>
function getWordCount() {
var myString = document.getElementById("myData").innerHTML;
document.getElementById("count").innerHTML = myString.split(" ").length;
}
/<script>
Output:
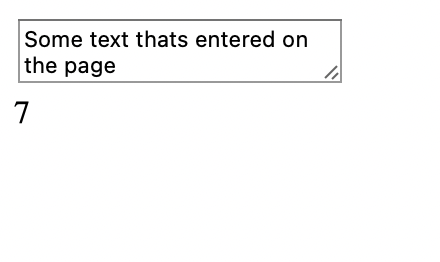
As you can see in the above example we have called a function onBlur of a textarea and read the text as a variable and then called two operations over it. First, we have done split based on space to get the array of words and then used length to get the count.
In the first example there are many ways we can go wrong. It's always better to use the trim() function along with the first example as there might be cases where the first and the last text in the string are spaces and we may get the wrong count, also what if there are multiple spaces between two words.
Example 2: Using regular expressions (RegEx)
Using regular expression is another way of knowing the number of words in a string, lets see an example,
var string = 'This is some random String to get its connt';
console.log(string.match(/\w+/g).length);
Output:
9
JSFiddle: https://jsfiddle.net/u80fx3pa/Have Questions? Post them here!
- How to Run JavaScript on Mac Terminal
- Get Current time in GMT/UTC using JavaScript
- How to yarn reinstall all Packages
- [javaScript] Convert text case to lowercase
- Get Device Screen Width and Height using javaScript
- Fix - npm start: sh: index.js: command not found
- npm WARN saveError ENOENT: no such file or directory, open /mnt/c/package.json
- JavaScript : Get url protocol HTTP, HTTPS, FILE or FTP
- JavaScript: Convert an Image into Base64 String
- JavaScript : Get current page address
- How to get query string in JavaScript HTML location.search
- Create React App using npm command with TypeScript
- JavaScript: Count Words in a String
- Add Animated Scrolling to Html Page Title Script
- How to send email from JavaScript HTML using mailto
- Javascript convert text case from uppercase to lowercase
- Submit html form on dropdown menu value selection or change using javascript
- Send Extra Data with Ajax Get or Post Request
- Fix: SyntaxError: The requested module does not provide an export named default
- Examples: Convert String to int in JavaScript
- 10 ways to Convert String to a Number in JavaScript
- Excel Fix: SECURITY RISK Microsoft has blocked macros from running because the source of this file is untrusted.
- Fix: ReferenceError: require is not defined in ES module scope [Node]
- [JavaScript] Remove all Newlines From String
- How to detect Browser and Operating System Name and Version using JavaScript
- PowerShell: Check if File Exists - Powershell
- How to Start Jupyter Notebook on Mac - MacOS
- Spell check not working in Gmail : Mac OS X - Mac-OS-X
- YAML Parser using Java Jackson Library Example - Java
- Share Story Feed on Facebook using URL - Facebook
- Disable jQuery button after being click - jQuery
- Java: Convert Char to ASCII - Java
- Time Testing with Java JUnit assertTimeout method - Java