CRUD stands for Create, Read, Update, and Delete. These are considered as the basic operations that can be performed on a database or data storage system.
Let's take a look at how to perform these operations with Spring Boot + plain JDBC
Step 1: Database Configuration
We will be using MySQL database, you may follow the below link and choose the configuration for wide variety of RDS or NoSQL databases.
applications.propertiesspring.datasource.url=jdbc:mysql://localhost/jdbctest
spring.datasource.username=root
spring.datasource.password=
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
pom.xml dependencies
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.14</version>
</dependency>
</dependencies>
Now let us create a database table called insurance_companies,
Step 2: Setting up our Pojo for the CRUD Examples
package org.code2care.springboot.jdbctutorial;
public class InsuranceCompany {
private String companyName;
private int headcount2020;
private String headOfficeLocation;
public InsuranceCompany() {
}
public InsuranceCompany(String companyName, int headcount2020, String headOfficeLocation) {
this.companyName = companyName;
this.headcount2020 = headcount2020;
this.headOfficeLocation = headOfficeLocation;
}
public String getCompanyName() {
return companyName;
}
public void setCompanyName(String companyName) {
this.companyName = companyName;
}
public int getHeadcount2020() {
return headcount2020;
}
public void setHeadcount2020(int headcount2020) {
this.headcount2020 = headcount2020;
}
public String getHeadOfficeLocation() {
return headOfficeLocation;
}
public void setHeadOfficeLocation(String headOfficeLocation) {
this.headOfficeLocation = headOfficeLocation;
}
@Override
public String toString() {
return "InsuranceCompany{" +
"companyName='" + companyName + '\'' +
", headcount2020=" + headcount2020 +
", headOfficeLocation='" + headOfficeLocation + '\'' +
'}';
}
}
Step 3: Methods for all CRUD operations
Create Operation
-
Example: Insert a single record using Prepared Statement
String sql = "INSERT INTO insurance_companies (company_name, headcount_2020, head_office_location) VALUES (?, ?, ?)";
jdbcTemplate.update(sql, "MetLife", 49000, "New York");
Example: Insert multiple records using Batch Update
package org.code2care.springboot.jdbctutorial;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Component;
import java.util.ArrayList;
import java.util.List;
@Component
public class InsuranceCompanyRunner implements CommandLineRunner {
@Autowired
private JdbcTemplate jdbcTemplate;
@Override
public void run(String... args) throws Exception {
List<InsuranceCompanies> companies = new ArrayList<>();
companies.add(new InsuranceCompanies("MetLife", 49000, "New York"));
companies.add(new InsuranceCompanies("Prudential Financial", 50527, "Newark"));
companies.add(new InsuranceCompanies("New York Life Insurance", 11902, "New York"));
companies.add(new InsuranceCompanies("Aflac", 11128, "Columbus"));
companies.add(new InsuranceCompanies("Liberty Mutual", 45000, "Boston"));
// Prepare the SQL insert statement
String sql = "INSERT INTO insurance_companies (company_name, headcount_2020, head_office_location) VALUES (?, ?, ?)";
List<Object[]> batchArgs = new ArrayList<>();
for (InsuranceCompanies company : companies) {
Object[] params = {company.getCompanyName(), company.getHeadcount2020(), company.getHeadOfficeLocation()};
batchArgs.add(params);
}
jdbcTemplate.batchUpdate(sql, batchArgs); //batch Update
}
}
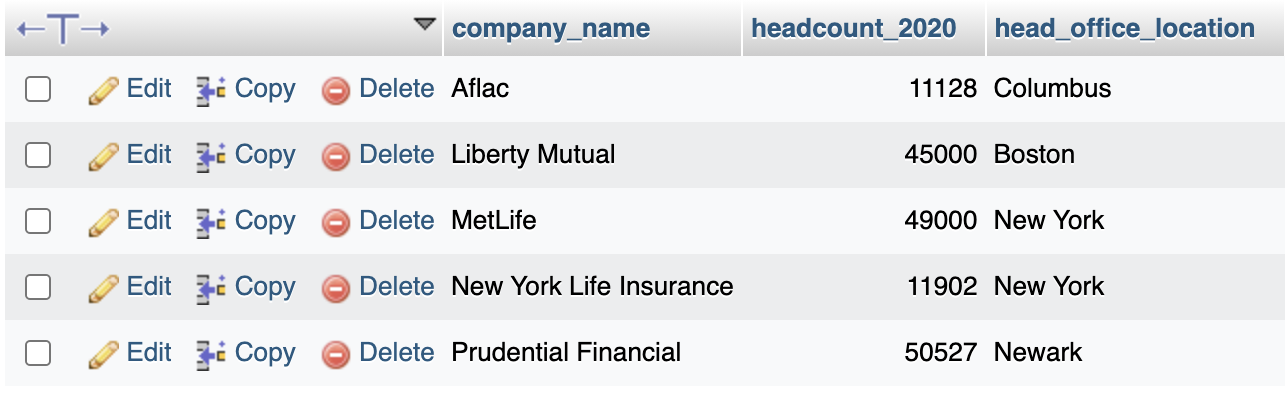
Read Operation using Prepared Statement
package org.code2care.springboot.jdbctutorial;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class InsuranceCompanyRunner implements CommandLineRunner {
@Autowired
private JdbcTemplate jdbcTemplate;
@Override
public void run(String... args) throws Exception {
String findQuery = "SELECT * FROM insurance_companies WHERE company_name = ?";
List<InsuranceCompanies> companies = jdbcTemplate.query(
findQuery,
new Object[]{"MetLife"},
(rs, rowNum) -> new InsuranceCompanies(
rs.getString("company_name"),
rs.getInt("headcount_2020"),
rs.getString("head_office_location")
)
);
for (InsuranceCompanies company : companies) {
System.out.println(company.toString());
}
}
}
Update Operation with Prepared Statement
String updateQuery = "UPDATE insurance_companies SET headcount_2020 = ? WHERE company_name = ?";
int rowsAffected = jdbcTemplate.update(updateQuery, 11129, "Alfac");
if (rowsAffected > 0) {
System.out.println("Record updated successfully.");
} else {
System.out.println("No record found with Company Name Alfac.");
}
Delete Operation with Prepared Statement
String deleteSQL = "DELETE FROM insurance_companies WHERE company_name = ?";
int rowsAffected = jdbcTemplate.update(deleteSQL, "Aflac");
if (rowsAffected > 0) {
System.out.println("Record deleted successfully.");
} else {
System.out.println("No record found with Company Name Aflac.");
}
Step 4: Complete CRUD Code in One Class
package org.code2care.springboot.jdbctutorial;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class InsuranceCRUD implements CommandLineRunner {
@Autowired
private final JdbcTemplate jdbcTemplate;
public InsuranceCRUD(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
@Override
public void run(String... args) throws Exception {
// 1. Create
String sql = "INSERT INTO insurance_companies (company_name, headcount_2020, head_office_location) VALUES (?, ?, ?)";
jdbcTemplate.update(sql, "MetLife", 49000, "New York");
System.out.println("Created Db entry for company MetLife");
// 2. Read
String findQuery = "SELECT * FROM insurance_companies WHERE company_name = ?";
List<InsuranceCompanies> companies = jdbcTemplate.query(
findQuery,
new Object[]{"MetLife"},
(rs, rowNum) -> new InsuranceCompanies(
rs.getString("company_name"),
rs.getInt("headcount_2020"),
rs.getString("head_office_location")
)
);
for (InsuranceCompanies company : companies) {
System.out.println("Read: "+ company.toString());
}
// 3. Update
String updateQuery = "UPDATE insurance_companies SET headcount_2020 = ? WHERE company_name = ?";
int rowsAffected = jdbcTemplate.update(updateQuery, 50000, "MetLife");
if (rowsAffected > 0) {
System.out.println("Updated record successfully.");
} else {
System.out.println("No record found with Company Name MetLife.");
}
// 4. Delete
String deleteSQL = "DELETE FROM insurance_companies WHERE company_name = ?";
rowsAffected = jdbcTemplate.update(deleteSQL, "MetLife");
if (rowsAffected > 0) {
System.out.println("Deleted record successfully.");
} else {
System.out.println("No record found with Company Name MetLife.");
}
}
}
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- What are E, K, N, S, T, U, V in Java Generics - Java
- How to uninstall Microsoft Edge from Mac (macOS) - MacOS
- INVALID FILE NAME: MUST CONTAIN ONLY [a-z0-9_.] Android Eclipse Error - Eclipse
- Free Unlimited Calls from MTNL & BSNL Landlines from 1st May 2015 - HowTos
- Fix Xcode: Git Repository Creation Failed - Git
- How to go to the End of File in Nano Editor - Linux
- How to make TextEdit the default text Editor on Mac - MacOS
- ls command to list only directories - Linux