In this tutorial we will cover step-by-step how to setup plain JDBC with Spring Boot 3 and Maven and MySQL Database.
Step 1: Downloading the Spring Initializr Project
Go to start.spring.io and fill in the following information for to download our JDBC project structure.
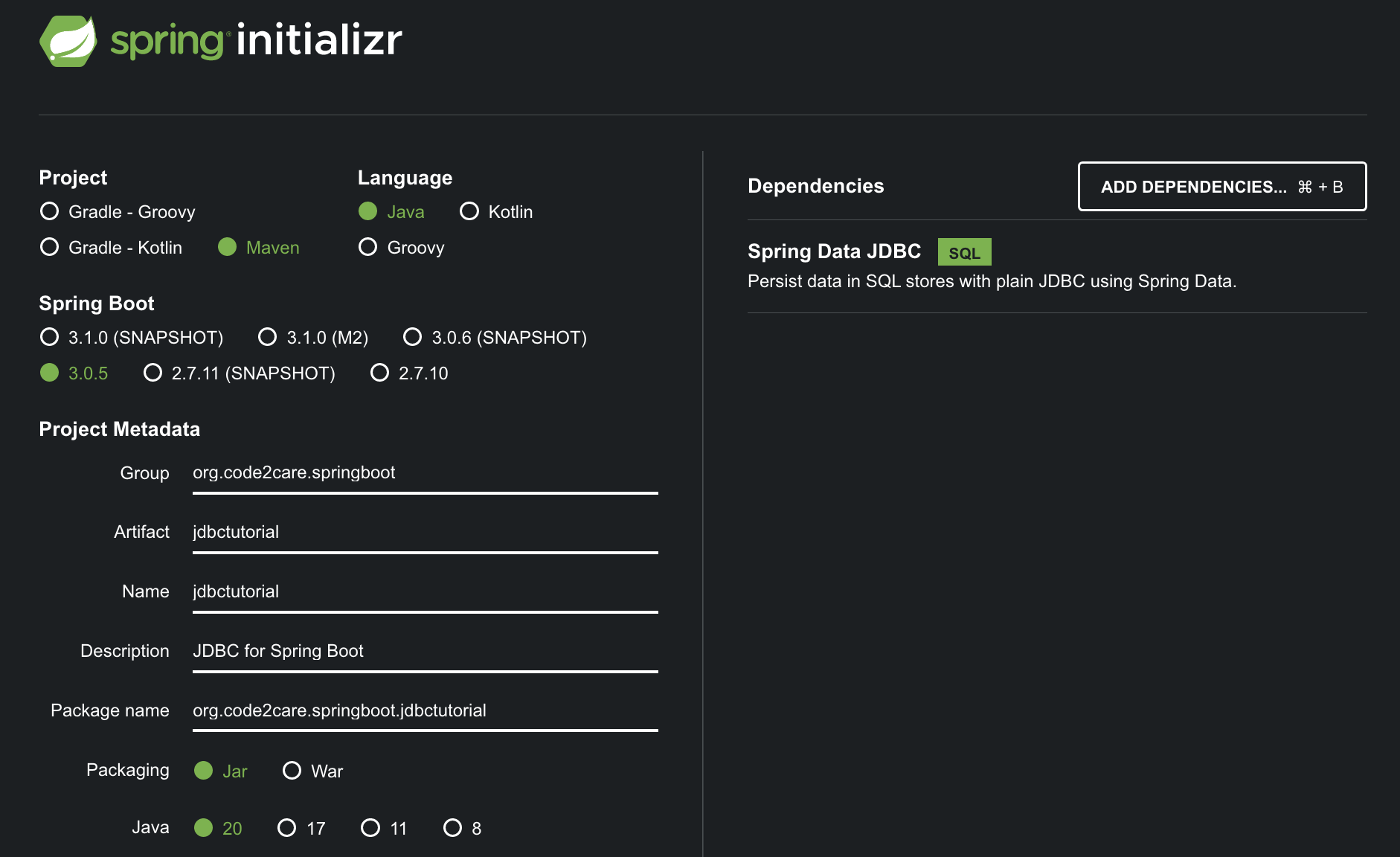
Make sure to select Project as Maven and Language as Java, you may choose the latest version of Spring Boot (not Snapshot or Mx versions), for me its 3.0.5
Do select the Packaging as jar and you may choose any version of Java thats available, I am going with the latest thats Java 20.
Once all is set, you can click on, Generate button at the bottom to download the project zip file.
Step 2: Importing the JDBC Project to IDE
- Unzip the project file we downloaded at Step 1.
- Open IntelliJ IDE.
- Go to Menu: File -> New -> Project From Existing Source...
- Now select your project folder and click Open
- Keep clicking next, and "Please select project SDK" choose your JDK (in my case JDK 20)
- Right-click on your project and select Maven -> Download Sources
Extract the zip project and open your IDE (Eclipse, IntelliJ, NetBeans or VSCode) to import the project, we will demonstrate using IDEA IntelliJ.
Step 3: Setting up MySQL Database.
Make sure you have download and installed MySQL Database. We will first create our test database.
Next lets create a simple database table.
Next, lets insert a record.
Finally lets check the record was inserted properly.
We are all set with the MySQL Database for our tutorial!
Step 4: Configuration for MySQL with Spring Boot
Open the applications.properties files in your project that you will find under src -> main -> resources and add the below details.
spring.datasource.url=jdbc:mysql://localhost/jdbctest
spring.datasource.username=root
spring.datasource.password=your-db-password-can-be-blank-by-default
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
Next open the pom.xml file add the MySQL connector dependency.
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.14</version>
</dependency>
We are all set with the JDBC configuration!
Step 5: Creating our CommandLineRunner Class
Let's first create a Pojo Message,
package org.code2care.springboot.jdbctutorial;
public class Message {
private String message;
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
@Override
public String toString() {
return "Message{" +
"message='" + message + '\'' +
'}';
}
}
Now we are all set for the final class that we need, we call it JDBCRunner
package org.code2care.springboot.jdbctutorial;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.jdbc.core.BeanPropertyRowMapper;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class JDBCRunner implements CommandLineRunner {
private String query = "Select * from message";
@Autowired
JdbcTemplate jdbcTemplate;
@Override
public void run(String... args) throws Exception {
List<Message> messages = jdbcTemplate.query(query, new BeanPropertyRowMapper<>(Message.class));
System.out.println(messages.get(0).getMessage());
}
}
Step 6: Running your Hello JDBC Project
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- VS Code Remove Unused Imports Keyboard Shortcut - HowTos
- What is exit(0), exit(1) ... exit(8) codes in Python Programming - Python
- Error : Invalid key hash.The key hash does not match any stored key hashes - Android
- osascript: Docker Desktop wants to create symlinks [macOS] - Docker
- Setting up JUnit 5 dependency with Maven Example - Java
- What is Bootstrap Jumbotron and how to use it - Bootstrap
- How to Exit Node Prompt from Mac Terminal - MacOS
- Code2care.org: A Decade of Serving the Tech Community - News
Go to the JdbctutorialApplication file and hit run, you should see "Hello Spring JDBC" printed in your IDE Console.
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org