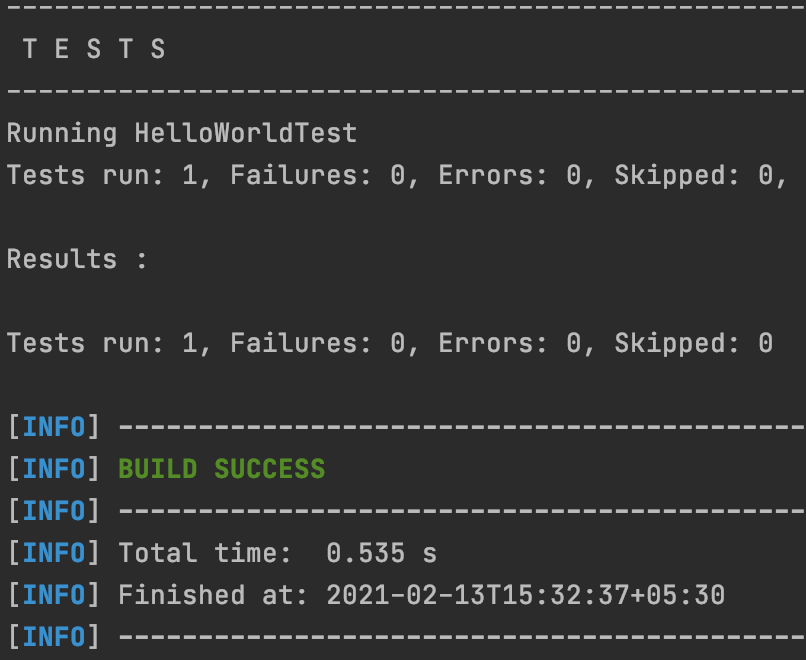
If you are working with a Apache Maven Java project and want to run your unit test cases, you would need to do the following,
1. Add Java JUnit dependencies to pom.xml
Open pom.xml file and the junit dependency as highlighted in bold, you can find the details on official JUnit website: https://junit.org/junit4/dependency-info.html
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 \
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>maven-juit-proj</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.1</version>
</dependency>
</dependencies>
</project>
2. The Java Project Structure
I am using Idea IntelliJ IDE on macOS, and my project structure is as below,
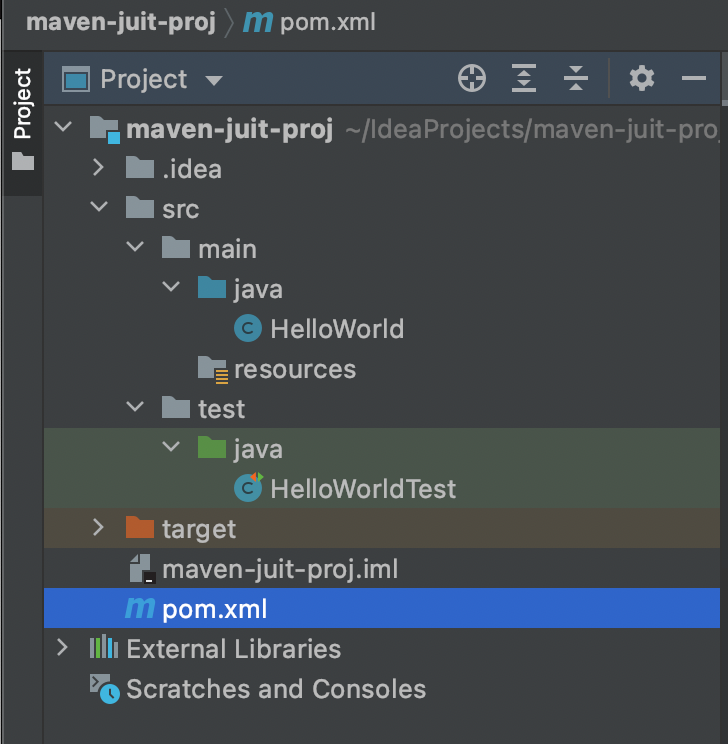
public class HelloWorld {
public String greeting(String name) {
return "Hello, " + name;
}
}
HelloWorldTest.java
import org.junit.Test;
import org.junit.Assert;
public class HelloWorldTest {
private HelloWorld hello = new HelloWorld();
@Test
public void test() {
Assert.assertEquals("Hello, Neo", hello.greeting("Neo"));
}
}
3. Running Maven Test
Now that we have our simple HelloWorld class and its HelloWorldTest class ready with the JUnit 4 dependency set in our pom.xml we are ready to run our text using maven commands,
⛔️ Make sure Maven is installed on your computer if not check out this article: How to install maven in macOS using Terminal Command
✏️ Command: mvn test - to run all the test cases in our project
code2care@mac maven-juit-proj % mvn test
[INFO] Scanning for projects...
[INFO]
[INFO] --------------------< org.example:maven-juit-proj >---------------------
[INFO] Building maven-juit-proj 1.0-SNAPSHOT
[INFO] --------------------------------[ jar ]---------------------------------
[INFO]
[INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ maven-juit-proj ---
[WARNING] Using platform encoding (US-ASCII actually) to copy filtered resources, i.e. build is platform dependent!
[INFO] Copying 0 resource
[INFO]
[INFO] --- maven-compiler-plugin:3.1:compile (default-compile) @ maven-juit-proj ---
[WARNING] Using platform encoding (US-ASCII actually) to copy filtered resources, i.e. build is platform dependent!
[INFO] Copying 0 resource
[INFO]
[INFO] --- maven-compiler-plugin:3.1:compile (default-compile) @ maven-juit-proj ---
[INFO] Changes detected - recompiling the module!
[WARNING] File encoding has not been set, using platform encoding US-ASCII, i.e. build is platform dependent!
[INFO] Compiling 1 source file to /Users/code2care/IdeaProjects/maven-juit-proj/target/classes
[INFO]
Downloading from central:
https://repo.maven.apache.org/maven2/org/apache/maven/surefire/surefire-junit4/2.12.4/surefire-junit4-2.12.4.pom
https://repo.maven.apache.org/maven2/org/apache/maven/surefire/surefire-junit4/2.12.4/surefire-junit4-2.12.4.pom
https://repo.maven.apache.org/maven2/org/apache/maven/surefire/surefire-providers/2.12.4/surefire-providers-2.12.4.pom
https://repo.maven.apache.org/maven2/org/apache/maven/surefire/surefire-providers/2.12.4/surefire-providers-2.12.4.pom
https://repo.maven.apache.org/maven2/org/apache/maven/surefire/surefire-junit4/2.12.4/surefire-junit4-2.12.4.jar
https://repo.maven.apache.org/maven2/org/apache/maven/surefire/surefire-junit4/2.12.4/surefire-junit4-2.12.4.jar
-------------------------------------------------------
T E S T S
-------------------------------------------------------
Running HelloWorldTest
Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.04 sec
Results :
Tests run: 1, Failures: 0, Errors: 0, Skipped: 0
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 2.241 s
[INFO] Finished at: 2021-02-13T14:51:48+05:30
[INFO] ------------------------------------------------------------------------
Let's add one more test case that would fail,
@Test
public void test2() {
Assert.assertEquals("Hello, Neo", hello.greeting("Sam"));
}
mvn test output:
Running HelloWorldTest
Tests run: 2, Failures: 1, Errors: 0, Skipped: 0, Time elapsed: 0.038 sec <<< FAILURE!
test2(HelloWorldTest) Time elapsed: 0.003 sec <<< FAILURE!
org.junit.ComparisonFailure: expected:<[Hello, Neo]> but was:<[Sam]>
at org.junit.Assert.assertEquals(Assert.java:117)
at org.junit.Assert.assertEquals(Assert.java:146)
at HelloWorldTest.test2(HelloWorldTest.java:15)
at java.base/java.lang.reflect.Method.invoke(Method.java:564)
at org.junit.runners.ParentRunner.runChildren(ParentRunner.
....
at java.base/java.lang.reflect.Method.invoke(Method.java:564)
at org.apache.maven.surefire.util.ReflectionUtils.invokeMethodWithArray(ReflectionUtils.java:189)
at org.apache.maven.surefire.booter.ForkedBooter.runSuitesInProcess(ForkedBooter.java:115)
at org.apache.maven.surefire.booter.ForkedBooter.main(ForkedBooter.java:75)
Results :
Failed tests: test2(HelloWorldTest): expected:<[Hello, Neo]> but was:<[Sam]>
Tests run: 2, Failures: 1, Errors: 0, Skipped: 0
[INFO] ------------------------------------------------------------------------
[INFO] BUILD FAILURE
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 0.760 s
[INFO] Finished at: 2021-02-13T15:24:00+05:30
[INFO] ------------------------------------------------------------------------
[ERROR] Failed to execute goal org.apache.maven.plugins:maven-surefire-plugin:2.12.4:test (default-test) on project maven-juit-proj: There are test failures.
[ERROR]
[ERROR] Please refer to /Users/code2care/IdeaProjects/maven-juit-proj/target/surefire-reports for the individual test results.
[ERROR] -> [Help 1]
[ERROR]
[ERROR] To see the full stack trace of the errors, re-run Maven with the -e switch.
[ERROR] Re-run Maven using the -X switch to enable full debug logging.
[ERROR]
[ERROR] For more information about the errors and possible solutions, please read the following articles:
[ERROR] [Help 1] http://cwiki.apache.org/confluence/display/MAVEN/MojoFailureException
✏️ Command: mvn -Dtest=<Test-Class-Name> test
To run individual test,
Example:
$ mvn -Dtest=HelloWorldTest test
✏️ Command: mvn -Dtest=<Test-Class-Name-1>,<Test-Class-Name-2 test
To run multiple tests at once.
Example:
$ mvn -Dtest=HelloWorldTest, HelloWorldTest2 test
✏️ Command: mvn -Dtest=<Test-Class-Name-1>#methodName test
To run a particular test method from a class.
Example:
$ mvn -Dtest=HelloWorldTest#test test
✏️ Command: mvn -Dtest=<Test-Class-Name-1>#methodName* test
To run all test methods what that matches the regular expression,
Example:
$ mvn -Dtest=HelloWorldTest#test* test
The above will run test and test1 methods from class HelloWorldTest
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Bash Command to Do Nothing with Example - Bash
- Graph API error when querying BookingBusinesses - ErrorExceededFindCountLimit, The GetBookingMailboxes request returned too many results - Microsoft
- [Vi/Vim] How to move cursor to the start of a line - MacOS
- JavaScript : Get url protocol HTTP, HTTPS, FILE or FTP - JavaScript
- Android: Unknown error code during application install : - Android
- Mac (macos) startup keyboard boot sequence combinations - MacOS
- Fix SharePoint 2019 installation error This product requires Visual C++ Redistributable Package for Visual Studio 2017 - SharePoint
- Increase Font Size of Eclipse Java Code - Eclipse