If you have a String in Java, or you are reading an external CSV, txt, or any other type of file line-by-line and want to split it based on a specific character then you can make use of the build-in split(String regex) function from String class,
Example 1: Split String with CSV LineString csvLine = "1,2,3,4,5,6";
String[] numStrArray = csvLine.split(",");
for (String num: numStrArray) {
System.out.println(num);
}
Output:
1
2
3
4
5
6
Example 2: Split String with Pipe Seperated Line
String pipeSeperatedLine = "1|2|3|4|6";
String[] numStrArray = pipeSeperatedLine.split("\|\");
for (String num: numStrArray) {
System.out.println(num);
}
Output:
1
2
3
4
5
6
Example 3: Split String with Space Separated Line
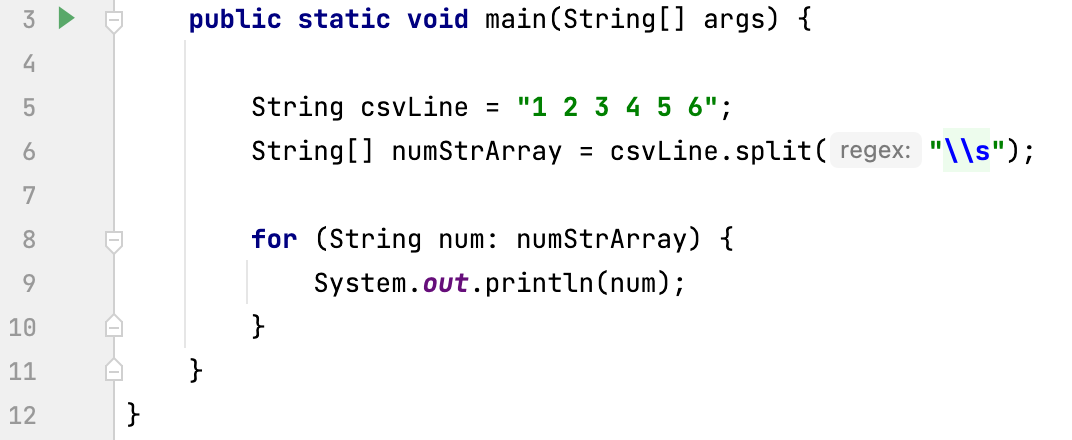
Splitting String in Java
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- How to search (find) in macOS Terminal Console Text - MacOS
- How to Select Two Separate Columns in Excel on Mac - Microsoft
- Add Sketch from iPhone to MacBook with macOS Monterey - MacOS
- Android Studio 4.2 Canary 1 now available - Android-Studio
- Fix: IntelliJ No SDK for New Project - Java
- How to Close Safari in Mac using Keyboard shortcut - MacOS
- Know installed PowerShell Version on Mac - Powershell
- How to Display Excel Spreadsheet on SharePoint site - SharePoint