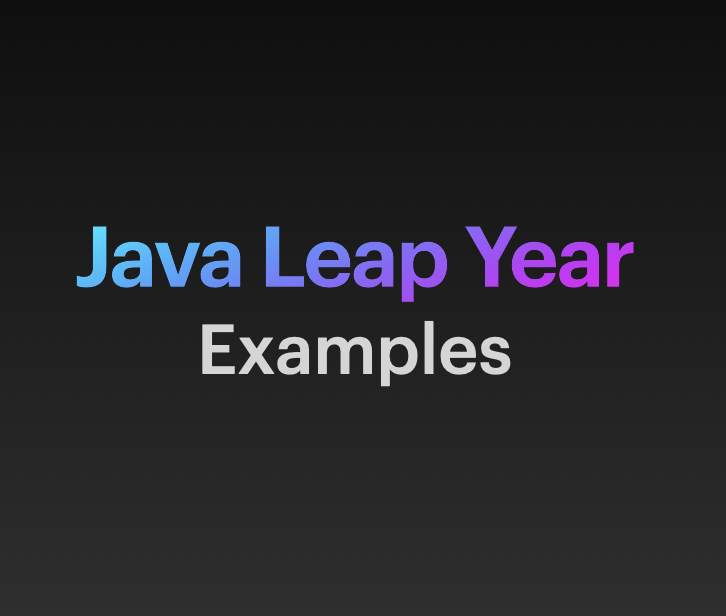
There are many ways you can check if the inputted year is a leap year or not using Java, but before we get started, let's understand what is a leap year.
What is a Leap Year?
A leap year is that year of the Gregorian calendar when we have an additional day in the year. It is also called as intercalary year or bissextile year. A Leap year will have 366 days instead of 365, this extra day is added to February - 29 days instead of 28.
Logic of identifying a Leap Year
A Year can be considered a leap year if the year is divisible by 4, also if that Year is divisible by 100 it should be also divisible by 400 to be a Leap year.
Some Examples to check Leap Years
Examples:
2019: 2019 is not divisible by 4, hence 2019 is not a leap year.
2020: 2020/4 = 0, 2020/100 is not true: So its a leap year.
1000: 2000/4 = 0, 1000/100=0 is not true, but 1000/400 is not 0, so its not a Leap year.
Java Code Example for Leap Year
Example 1:
package org.code2care;
/**
* Leap year program Java
*
* This Class demonstrates if a
* year is a leap year or not
*
* Author: Code2care
*/
public class LeapYearJava {
public static void main(String[] args) {
int year = 1000;
if(isLeapYear(year)) {
System.out.println("Year " + year + " is a Leap Year!");
} else {
System.out.println("Year " + year + " is a not Leap Year!");
}
}
/**
* Leap Year Logic:
*
* Logic:
* if the year % 4 == 0
* if yes
* Check if the year % 100 == 0
* if yes
* 3) Check if year % 400 == 0
* if no
* Not a Leap Year
* if no
* Its a Leap Year
* if no
* Its not a Leap Year
*
* @param year int value that represents a year
* @return true is year is a leap year, else false
*/
public static boolean isLeapYear(int year) {
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0) {
return true;
} else {
return false;
}
} else {
return true;
}
} else {
return false;
}
}
}
Example 2:
package org.code2care;
/**
* Leap year program Java
*
* This Class demonstrates if a
* year is a leap year or not
*
* Author: Code2care
*/
public class LeapYearJava {
public static void main(String[] args) {
int year = 1000;
if(isLeapYear(year)) {
System.out.println("Year " + year + " is a Leap Year!");
} else {
System.out.println("Year " + year + " is a not Leap Year!");
}
}
/**
* Leap Year Logic:
*
* Logic:
* if the year % 4 == 0
* if yes
* Check if the year % 100 == 0
* if yes
* 3) Check if year % 400 == 0
* if no
* Not a Leap Year
* if no
* Its a Leap Year
* if no
* Its not a Leap Year
*
* @param year int value that represents a year
* @return true is year is a leap year, else false
*/
public static boolean isLeapYear(int year) {
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0) {
return true;
} else {
return false;
}
} else {
return true;
}
} else {
return false;
}
}
}
This logic is much clearer to understand,
/**
* Pseudo code for java leap year program
*
* If year % 4 ! = 0
* not leap year
* else if year % 400 == 0
* leap year
* else if year % 100 == 0
* not leap year
* else
* leap year
*
* @param year int value that represents a year
* @return true is year is a leap year, else false
*/
public static boolean isLeapYearLogic2(int year) {
if (year % 4 != 0) {
return false;
} else if (year % 400 == 0) {
return true;
} else if (year % 100 == 0) {
return false;
} else {
return true;
}
}
Leap year code in Java is often tested in Schools and Colleges! Hope you find it helpful!
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Calculate days between dates using dateutils ddiff command - Linux
- [Solved] Notepad++ Menu Bar Missing - NotepadPlusPlus
- How to enable Do Not Disturb mode for Notification Center in Mac OS X 10.10 Yosemite - Mac-OS-X
- Install GitHub Command Line Tool on Mac - Git
- Set Falling Show on Website for Christmas using Pure CSS Code - CSS
- How to Find and View Stored Wi-Fi Password on iPhone - iOS
- Merge Mp3/M4a Audio Files Online Tool - Tools
- Fix - zsh: permission denied: ./gradlew [Android Studio Terminal] - Android-Studio