We can make use of the isBefore(), isAfter() and, isEqual() methods to compare two dates in Java of type java.time.LocalDate.
Example 1: Compare if two dates are equal
LocalDate date1 = LocalDate.of(2023, 10, 18);
LocalDate date2 = LocalDate.of(2023, 10, 19);
boolean isEqual = date1.isEqual(date2);
Example 2: Check if one date is before another
LocalDate date1 = LocalDate.of(2023, 10, 18);
LocalDate date2 = LocalDate.of(2023, 10, 19);
boolean isEqual = date1.isBefore(date2);
Example 3: Check if one date is after another
LocalDate date1 = LocalDate.of(2023, 10, 18);
LocalDate date2 = LocalDate.of(2023, 10, 19);
boolean isEqual = date1.isAfter(date2);
Now let's combine this code with a if-else ladder.
package org.code2care.examples;
import java.time.LocalDate;
/**
* Compare two dates in Java
*
* Author: code2care.org
*
*/
public class DateComparisonExample {
public static void main(String... args) {
LocalDate date1 = LocalDate.of(2023, 10, 18);
LocalDate date2 = LocalDate.of(2023, 10, 19);
if (date1.isEqual(date2)) {
System.out.println(date1 + " is equal to " + date2);
} else if (date1.isBefore(date2)) {
System.out.println(date1 + " is before " + date2);
} else {
System.out.println(date1 + "is after" + date2);
}
}
}
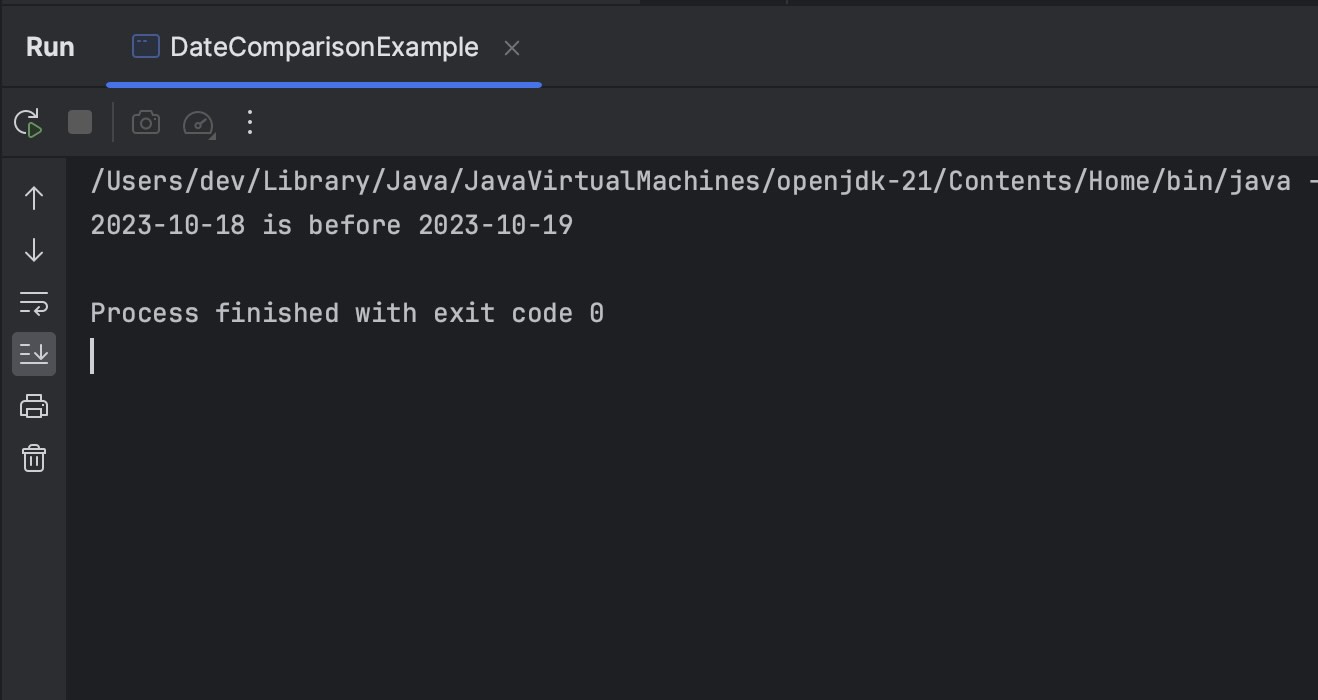
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Docker - Incompatible CPU detected - M1/M2 Mac (macOS Sonoma) - Docker
- How to install npm on Ubuntu - Linux
- Command to check Last Login or Reboot History of Users and TTYs - Linux
- How to Copy all text to Clipboard in Vim - vi
- Program 38: Store two numbers in variables and print their sum - Python-Programs
- Fix - Microsoft Teams Operation Failed With Unexpected Error - Teams
- Bash Command to Find OS Version in Terminal - Bash
- How to check PowerShell version - Powershell