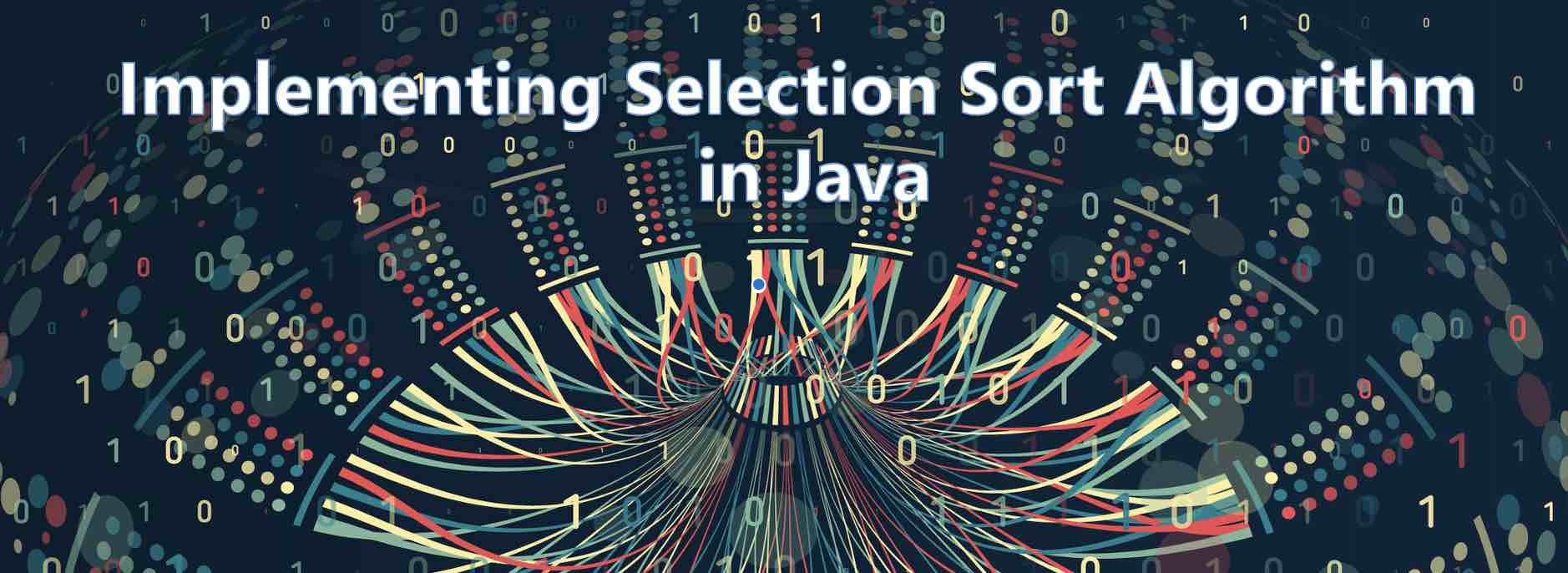
package org.code2care.java.sorting.algo;
import java.util.Arrays;
/**
* SelectionSort Example in Java
*
* Author: Code2care.org
* Date: 28 April 2023
* Version: v1.0
*
*/
public class SelectionSortJavaExample {
public static void main(String[] args) {
//Unsorted Array
int[] unsortedArray = {4, 99, 2, 11, 34, 67, 54, 12, 45, 245, 234, 12, 200};
SelectionSortJavaExample selectionSort = new SelectionSortJavaExample();
int[] sortedArray = selectionSort.selectionSortAlgorithm(unsortedArray);
System.out.println(Arrays.toString(sortedArray));
}
public int[] selectionSortAlgorithm(int[] arrayOfNumbers) {
int arrayLength = arrayOfNumbers.length;
for (int i = 0; i < arrayLength - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < arrayLength; j++) {
if (arrayOfNumbers[j] < arrayOfNumbers[minIndex]) {
minIndex = j;
}
}
int tempVariable = arrayOfNumbers[minIndex];
arrayOfNumbers[minIndex] = arrayOfNumbers[i];
arrayOfNumbers[i] = tempVariable;
}
return arrayOfNumbers;
}
}
Time Complexity | |||||
---|---|---|---|---|---|
Best Case | Average Case | Worst Case | Space Complexity | ||
Selection Sort | O(n^2) | O(n^2) | O(n^2) | O(1) |
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Fix - macOS Sonoma 14 - zsh: command not found: python - Python
- How to Join Microsoft Teams Meeting Without an Account - Teams
- Graph API error when querying BookingBusinesses - ErrorExceededFindCountLimit, The GetBookingMailboxes request returned too many results - Microsoft
- Where are screenshots saved on Windows 11 using Snipping Tool? - Windows-11
- Solution: Office 365 - Request denied while Login Verification (MFA) - Windows
- Step-by-Step: How to delete a git branch from local as well as remote origin - Git
- Check if String Contains a Substring - Python - Python
- How to Initialize ArrayList Java with Values - Java