Just like any other programming/scripting language, one of the most common ways to debug your code is by using print statements. In JavaScript, there are several ways to print messages to the console or to the page. In this article, we'll explore some of the most popular ways to print messages in JavaScript, including,
- console.log()
- console.error()
- document.write()
- alert()
- window.print()
console.log()
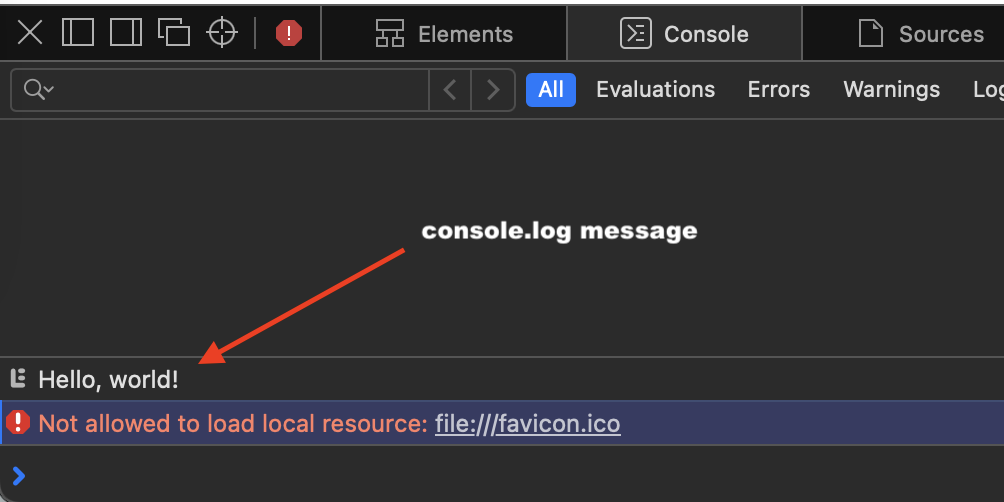
console.log('Hello, world!');
The console.log() method is the most commonly used way to print messages to the console in JavaScript. It takes one or more arguments and prints them to the console, separated by commas.
You can also pass multiple arguments to console.log()
Example:console.log('The answer is', 42);
console.log() method can be very useful for debugging your JavaScript code, you can also print the results of function calls to the console.
You can see the console logs using the Inspect Dev Tool of any web browser and under console tab.
console.error()
-
Exmple:
console.error('Error occurred while calculating Interest.');
The console.error() method is similar to console.log(), but it's used to print error messages to the console. Error messages should be printed using the console.error() making it easier to spot them in the console.
document.write()
-
Example:
document.write('Hello, world!');
The document.write() method should be used to print messages directly to the HTML page. It takes one argument, which is the message to be printed.
window.print()
-
Example:
window.print();
The window.print() method is used to print the current page. When called, it opens the print dialog box, allowing the user to print the page.
The above code will open the print dialog box, allowing the user to print the current page.
window.print() can be useful for allowing users to print out important information from your web page.
alert()
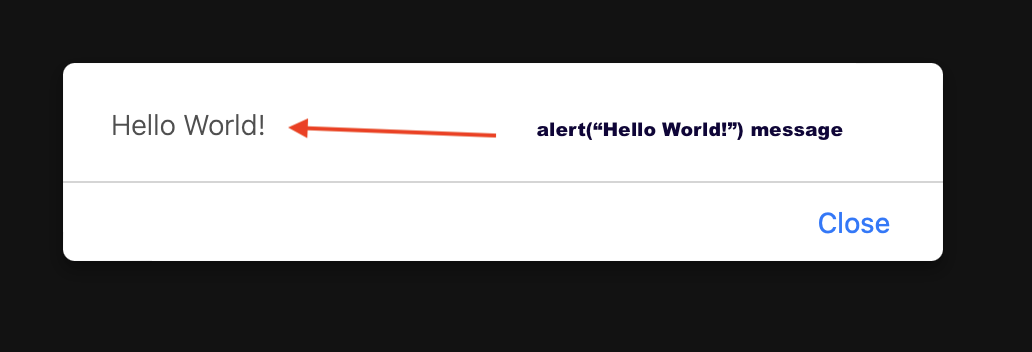
alert('Hello, world!');
The alert() method can be used to display a message box with a message and an OK or Close button on the web browser. It takes one argument, which is the message to be displayed.
Note: alert() can be at times useful for displaying important messages to the user, but it can also be annoying and have a bad user experience if overused.
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- How to Run JavaScript on Mac Terminal
- Get Current time in GMT/UTC using JavaScript
- How to yarn reinstall all Packages
- [javaScript] Convert text case to lowercase
- Get Device Screen Width and Height using javaScript
- Fix - npm start: sh: index.js: command not found
- npm WARN saveError ENOENT: no such file or directory, open /mnt/c/package.json
- JavaScript : Get url protocol HTTP, HTTPS, FILE or FTP
- JavaScript: Convert an Image into Base64 String
- JavaScript : Get current page address
- How to get query string in JavaScript HTML location.search
- Create React App using npm command with TypeScript
- JavaScript: Count Words in a String
- Add Animated Scrolling to Html Page Title Script
- How to send email from JavaScript HTML using mailto
- Javascript convert text case from uppercase to lowercase
- Submit html form on dropdown menu value selection or change using javascript
- Send Extra Data with Ajax Get or Post Request
- Fix: SyntaxError: The requested module does not provide an export named default
- Examples: Convert String to int in JavaScript
- 10 ways to Convert String to a Number in JavaScript
- Excel Fix: SECURITY RISK Microsoft has blocked macros from running because the source of this file is untrusted.
- Fix: ReferenceError: require is not defined in ES module scope [Node]
- [JavaScript] Remove all Newlines From String
- How to detect Browser and Operating System Name and Version using JavaScript
- Quickly install VS Code on macOS Sonoma/Ventura - MacOS
- Read file from Windows CMD (Command Line) - Windows
- Health Status Page for OpenAI ChatGPT or GPT 4 - HowTos
- Share Image to WhatsApp with Caption from your Android App - WhatsApp
- PowerShell Alias Type Commands List for Mac - MacOS
- Get the Complete Sha256 Container ID for Docker Run Command - Docker
- Tesla hit by a complete network and mobile app outage - 23 Sept 2020 11am ET (US and Europe) - News
- Java: Convert Byte to Binary String Example - Java