Let us take a look at a simple example of how to make use of Spring Boot AI with LLM AI models such as ChatGPT
Step 1: Create a new project using Spring CLI
# spring boot new --from ai --name myai-app
Getting project from https://github.com/rd-1-2022/ai-openai-helloworld
Created project in directory 'myai-app'
Step 2: Add the API key details in the application.properties file
spring.ai.openai.api-key=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
spring.ai.openai.chat.options.model=gpt-3.5-turbo
spring.ai.openai.chat.options.temperature=0.6
Step 3: Run the application and access endpoint /ai/simple
You can notice that we already get a hello-world project setup with a SimpleAIController created for us with an API end-point /ai/simple with a default prompt as "Tell me a joke"
package org.springframework.ai.openai.samples.helloworld.simple;
import org.springframework.ai.chat.ChatClient;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.Map;
@RestController
public class SimpleAiController {
private final ChatClient chatClient;
@Autowired
public SimpleAiController(ChatClient chatClient) {
this.chatClient = chatClient;
}
@GetMapping("/ai/simple")
public Map<String, String> completion(@RequestParam(value = "message", defaultValue = "Tell me a joke") String message) {
return Map.of("generation", chatClient.call(message));
}
}
Example 1: Default Prompt:
# curl localhost:8080/ai/simple
Example 2: Custom Prompt:
# curl localhost:8080/ai/simple?message=What is 2+5
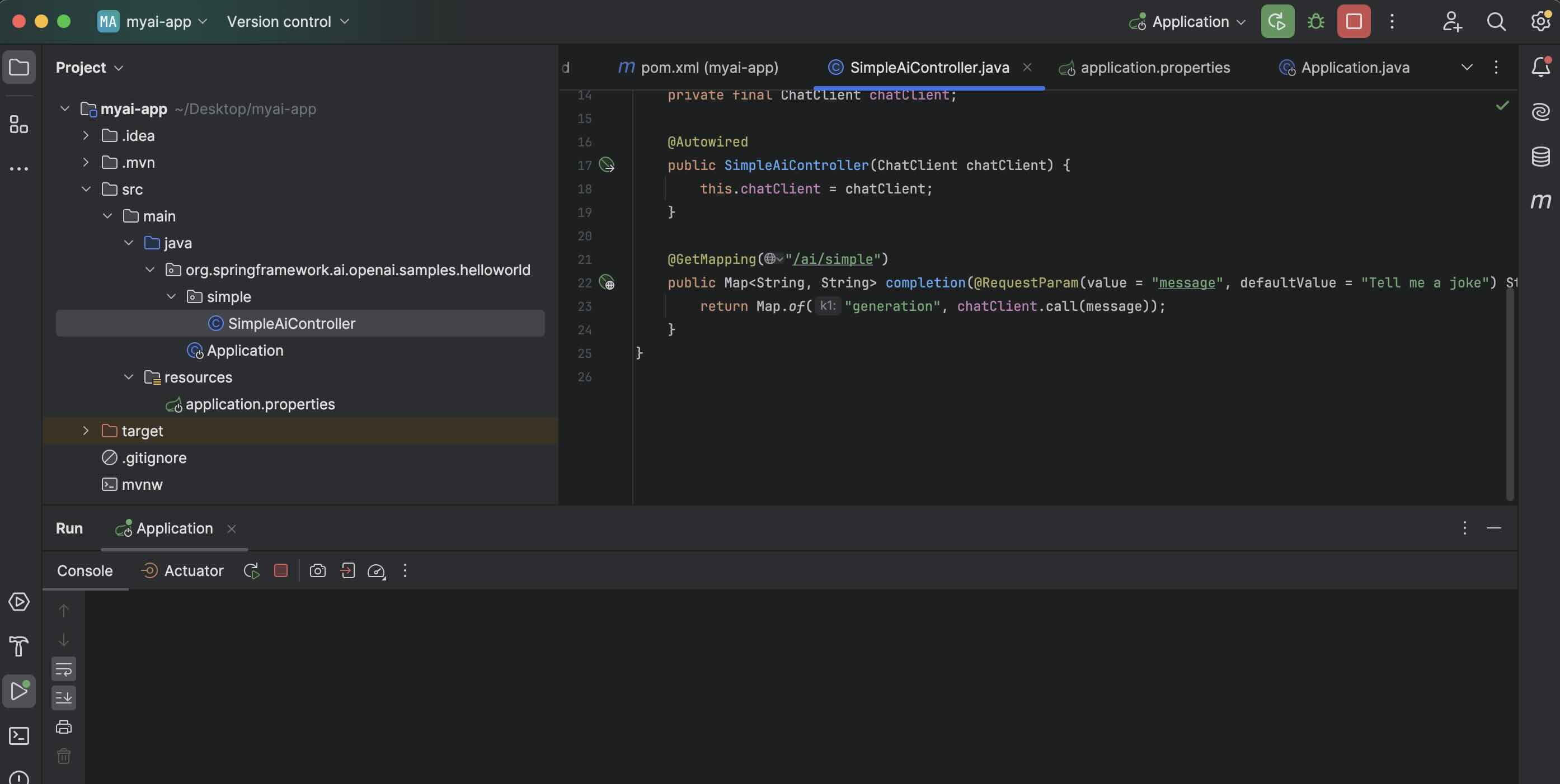
Note: If you do not add the API key you will get an exception:
java.lang.IllegalArgumentException: OpenAI API key must be set
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Record Audio and Save on System Tool - Tools
- How to disable warnings while Python file execution - Python
- How to exit from nano command - Linux
- Turn Off Google Analytics intelligence Alert Emails - Google
- How to tar.gz a directory or folder Command - Linux
- How to Exit a File in Terminal (Bash/Zsh) - Linux
- clear is not recognized as an internal or external command operable program or batch file. - DOS
- How to change Android Titlebar theme color from Purple - Android