It's year 2023 and one may not use the XML based Spring Application Context for new projects in production, but there are few reasons why its still relevant,
- Working on a legacy product: If you are working on a legacy product that uses an older version of Spring or a configuration file written in XML format, you may need to use ClassPathXmlApplicationContext to initialize the Spring application context.
- Learning Spring: If you are new to Spring and want to learn how it works, starting with ClassPathXmlApplicationContext is a good option. It is a simple way to set up a Spring application context using an XML configuration file, and it can help you understand the basic concepts of Spring.
- Limited resources constraint: If you are working on a project with limited resources, using ClassPathXmlApplicationContext is a good option as it has a smaller footprint compared to other Spring container implementations like AnnotationConfigApplicationContext.
- Testing: ClassPathXmlApplicationContext is also commonly used in testing scenarios, where it can be used to create a lightweight Spring context for testing individual components of a larger Spring application.
- Integrating with other frameworks: In some cases, other frameworks may require the use of ClassPathXmlApplicationContext to integrate with Spring. For example, some legacy ORM frameworks may require configuration via an XML file and integration with Spring through ClassPathXmlApplicationContext.
- Limited deployment options: Some cloud deployment platforms may not support certain newer Spring container implementations, in which case ClassPathXmlApplicationContext can be used as a fallback option.
Let us take a example of IoC using a Interface Teacher and its implementations like MathTeacher and ScienceTeacher.
Teacher.javapackage example.spring_ioc_with_xml;
/**
* Our interface to learn
* String IoC using XML
* based ClassPathXmlApplicationContext
*
*/
public interface Teacher {
String doStudies();
}
ScienceTecher.java
package example.spring_ioc_with_xml;
public class ScienceTeacher implements Teacher {
@Override
public String doStudies() {
return "Let us study chapter 'Work & Energy'!";
}
}
MathTecher.java
package example.spring_ioc_with_xml;
public class MathTeacher implements Teacher {
@Override
public String doStudies() {
return "Let us study chapter 'Quadratic Equations'!";
}
}
MusicTeacher.java
package example.spring_ioc_with_xml;
public class MusicTeacher implements Teacher {
@Override
public String doStudies() {
return "Let us learn how to play Guitar!";
}
}
Now lets create our ApplicationContext.xml file.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<bean id="mathTeacher" class="example.spring_ioc_with_xml.MathTeacher"/>
<bean id="scienceTeacher" class="example.spring_ioc_with_xml.ScienceTeacher" />
<bean id="musicTeacher" class="example.spring_ioc_with_xml.MusicTeacher" />
</beans>
And its time to create a Main class with the implementation of ClassPathXmlApplicationContext to perform IoC.
package example.spring_ioc_with_xml;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ClassPathXmlApplicationContext classPathXmlApplicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
Teacher teacher = classPathXmlApplicationContext.getBean("scienceTeacher", ScienceTeacher.class);
String scienceTeachersMessage = teacher.doStudies();
System.out.println(scienceTeachersMessage);
teacher = classPathXmlApplicationContext.getBean("mathTeacher", MathTeacher.class);
System.out.println(teacher.doStudies());
teacher = classPathXmlApplicationContext.getBean("musicTeacher", MusicTeacher.class);
System.out.println(teacher.doStudies());
}
}
Output:
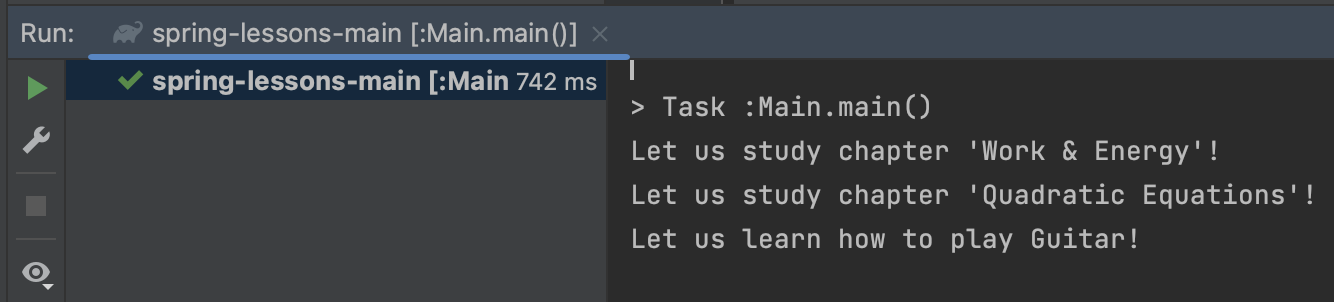
Note:
Make sure to add the below dependency in your build.gradle dependencies.
implementation group: 'org.springframework', name: 'spring-context', version: '5.3.22'
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Download a SSL Certificate from a URL in Terminal - Bash
- Command: How to scp a file to remote server location? - HowTos
- SharePoint installation - Appfabric installation failed because installer MSI returned with error code:1603 - SharePoint
- Trigger Flow on selected Listitem from SharePoint view - create button with JSON column formatting - SharePoint
- Restore deleted Office 365 SharePoint group site - SharePoint
- Convert Java String to JSON Object using Jackson - Java
- How to Run Terminal As Admin on Mac - MacOS
- Remove git config at Local, Global or System Levels? - Git