package org.code2care.exceptions;
import java.util.Arrays;
import java.util.List;
public class UnsupportedOperationExceptionExample1 {
public static void main(String[] args) {
List<String> nameList = Arrays.asList("Sam", "Alex", "Mike", "Annie", "Jane");
nameList.add("Andrew");
}
}
Example 2: LinkedList
public class UnsupportedOperationExceptionExample2 {
public static void main(String[] args) {
List<String> nameList = LinkedList.of("Sam", "Alex", "Mike", "Annie", "Jane");
nameList.add("Andrew");
}
}
Example 3: Set - HashSet
public class UnsupportedOperationExceptionExample3 {
public static void main(String[] args) {
Set<Integer> numbers = Set.of(10,20,30,40,50);
numbers.add(44);
}
}
All the three above examples will give java.lang.UnsupportedOperationException because when you make use of Arrays.asList() or the Collection.of() methods you get an immutable collection, thus you cannot modify such collection by adding, deleting our updating it.
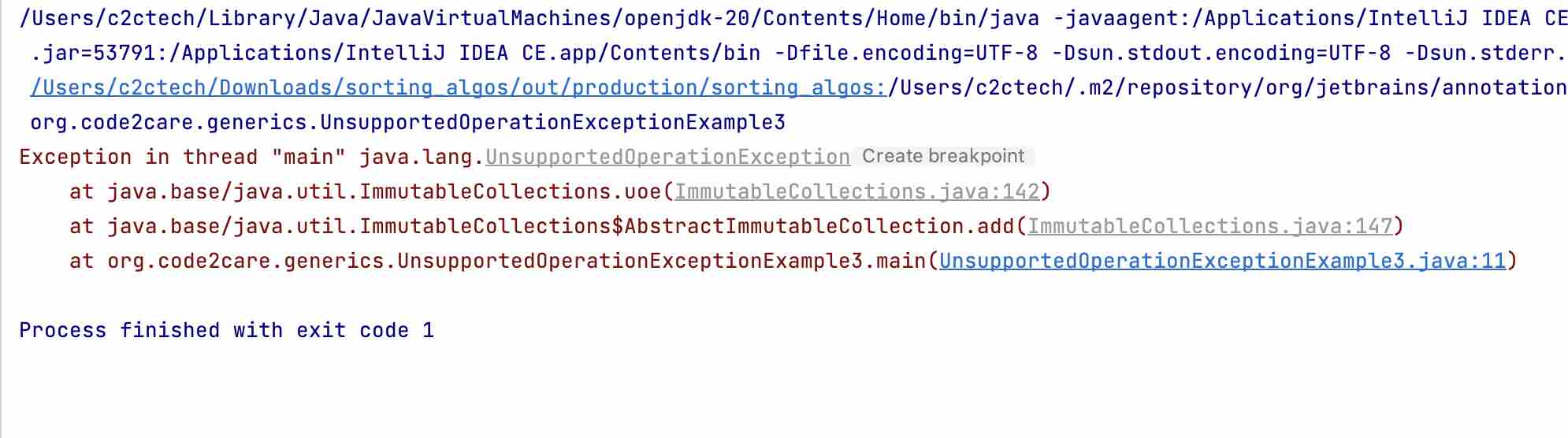
Fix: java.lang.UnsupportedOperationException
The fix is simple, just create the collection as you do use the new keyword and add elements using the add method(s).
Example:public class FixUnsupportedOperationException {
public static void main(String[] args) {
List<String> nameList = new ArrayList();
nameList.add("Sam");
nameList.add("Alex");
nameList.add("Mike");
nameList.add("Annie");
nameList.add("Jane");
nameList.add("Andrew"); //No issue
}
}
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- Send Extra Data with Ajax Get or Post Request - JavaScript
- Cargo Watch: To Recompile Rust Project Automatically - Rust
- How to turn off Automatically adjust brightness on Mac Ventura 13 - MacOS
- How to create a Array (not using list) in Python - Python
- Notepad++ Mark and Copy feature - NotepadPlusPlus
- How to Run PowerShell Script (Mac/Windows/Linux) - Powershell
- Hurry! Uninstall Adobe Flash Player - End Of Life, support end in December 2020 - News
- Using Document Map in Notepad++ - NotepadPlusPlus