Introduction to the Object Class
The Object class from java.lang package is the root class of all classes in Java Programming Langauge. All classes have the Object class as its Superclass (Parent class).
How to view the Object Class Code?
You can find the Object.class file in the core API rt.jar (Java Runtime Environment), If you use IDE like IntelliJ or Visual Studio Code, you can make use of the built-in decompiler.
Using IntelliJ:
- Right Click on the Object keyword in a file and select Go To -> Implementation (s)
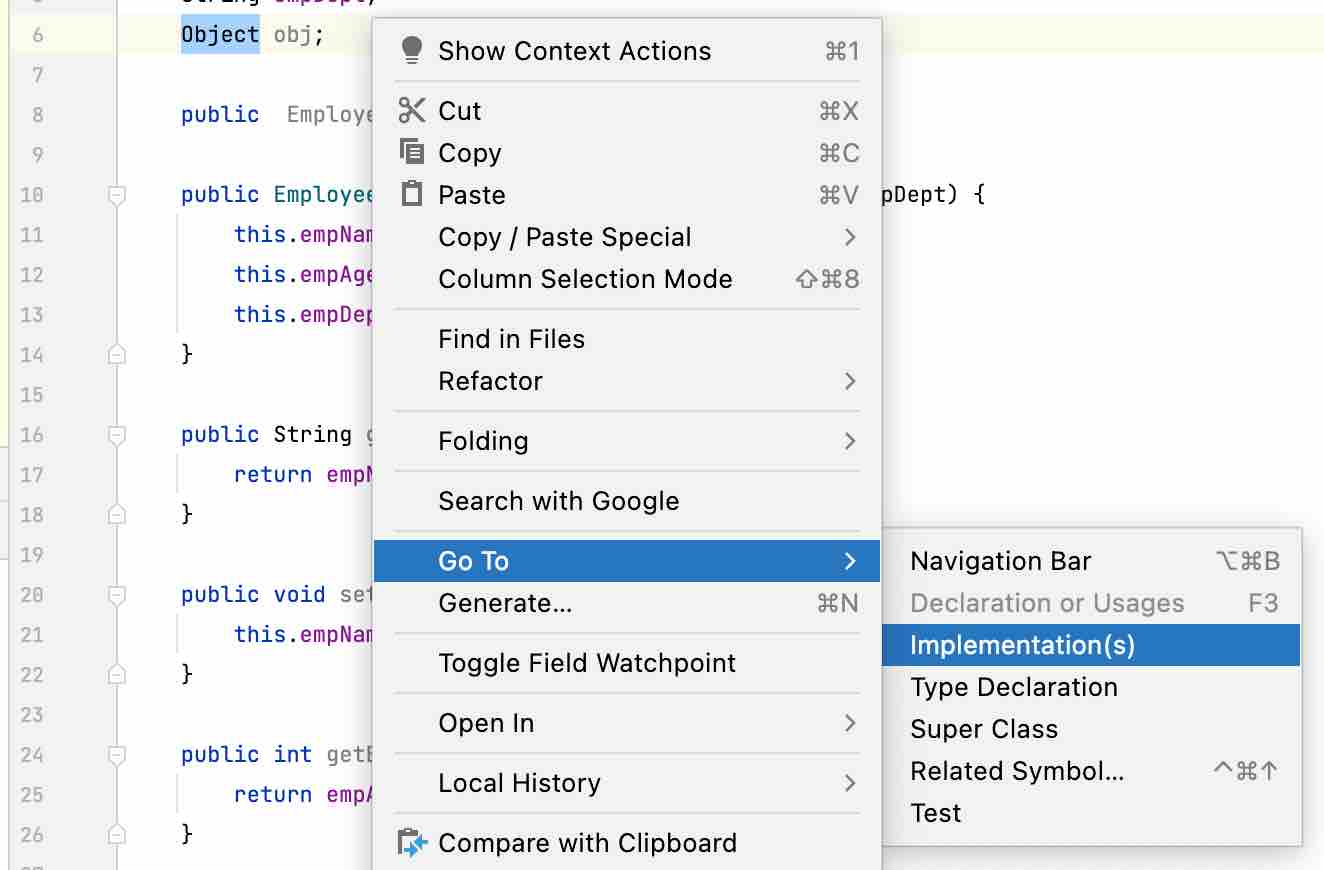
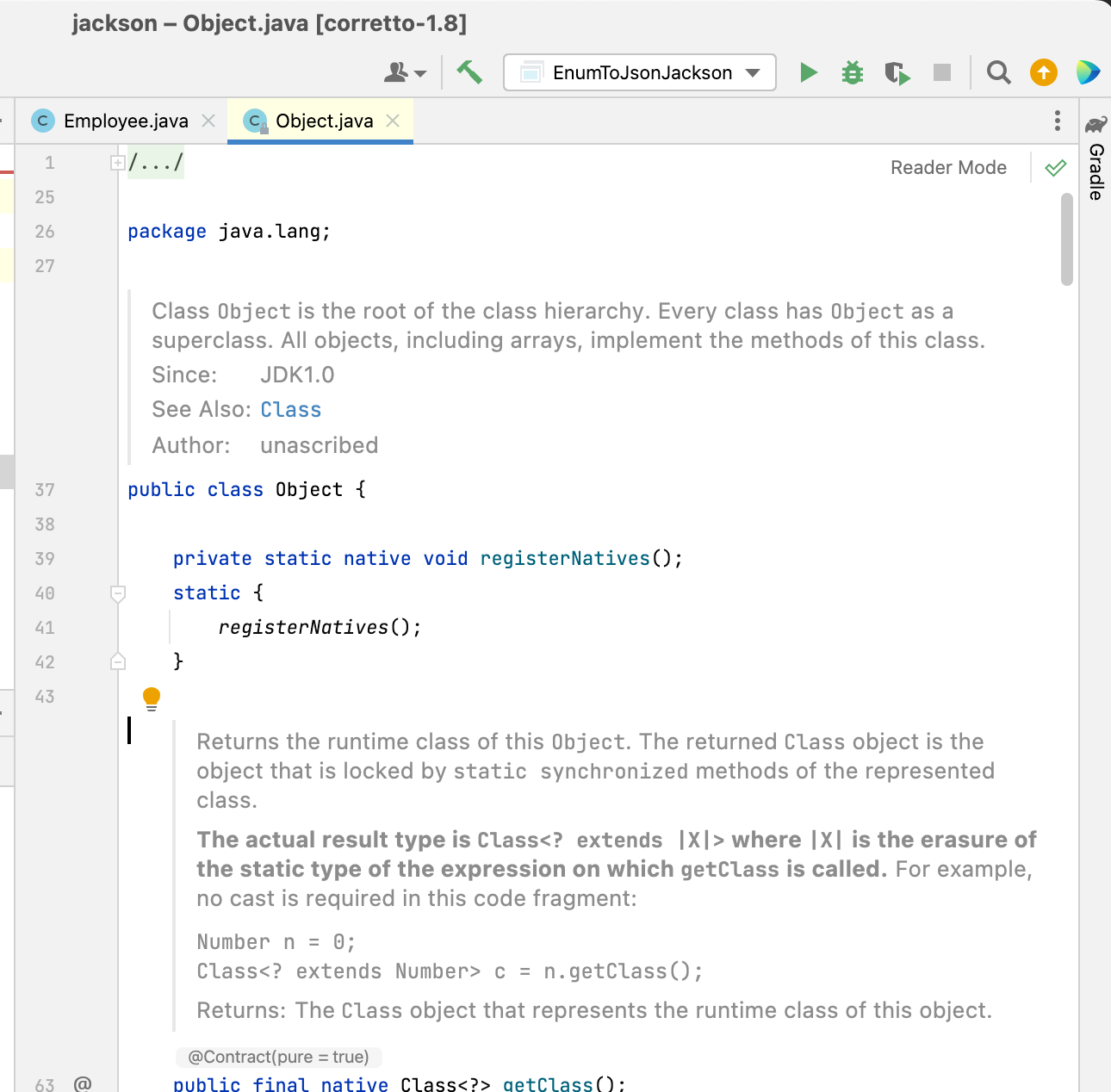
You can also make use of decompilers or view the code online http://www.docjar.com/html/api/java/lang/Object.java.html.
List of Methods in Object.java class
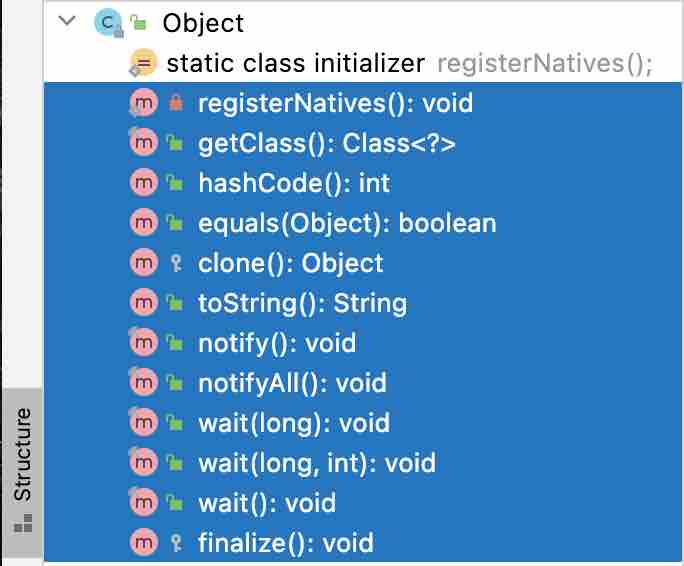
There are 12 methods available in the Object class. The methods from the Object class are available for all classes that we create. Out of these 12 methods, 7 methods are of type native - they are defined and executed using code written in another programming language native to the Operating System (mostly C Langauge)
- private static native void registerNatives();
- public final native Class<?> getClass();
- public native int hashCode();
- protected native Object clone();
- public final native void notify();
- public final native void notifyAll();
- public final native void wait(long timeout);
- public boolean equals(Object obj)
- public String toString()
- public final void wait(long timeout, int nanos)
- public final void wait()
- protected void finalize()
Facing issues? Have Questions? Post them here! I am happy to answer!
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Simple Crossword Puzzle example using Pure HTML, CSS and JavaScript - Html
- Fix: This file is set to read-only. Try again with a different file name. [Windows 10/11] - Windows
- How to add Date and Time to Windows Notepad File - NotepadPlusPlus
- How to load zsh profile on opening shell terminal - MacOS
- Program 4: Input Name and Age and Print - 1000+ Python Programs - Python-Programs
- How to Show Commit Date in Eclipse Git History Details - Git
- How to stop/start/restart apache server using command [Ubuntu] - Ubuntu
- Flash Player will no longer be supported after December 2020. Turn off [Google Chrome] - Chrome