In this tutorial, we will take a look at ways in which how we can declare and initialize an Array in Java Programming Language,
Part 1: Declaring an Array
Syntax:data-type[ ] variableName;
Example:int[] myIntArray;
Declaring an Array in Java is quite similar to declaring a variable, the only difference you will see is that the datatype has rectangular brackets [] around it, wherever you see these brackets around a datatype in Java it is to signify that its an Array,
More Examples:// Array of Primitive Type
int[] evenNumbers;
double[] measurements;
boolean[] validity;
// Array of Object Type
String[] userNames;
Employee[] employeeArr;
⛏️ You can also declare an Array as int evenNumbers[] where the brackets are placed at the end of the variable name and not the datatype, but this is not the preferred way!
Arrays are Objects in Java, so if you try to use the array Object that's defined with unitized to null, you will get NullPointerException.
public static void main(String[] args) {
int[] evenNumbers = null;
System.out.println(evenNumbers.length);
}
⛔️ Exception in thread "main" java.lang.NullPointerException
at Example.main(Example.java:5)
Part 2: Initilizing an Array
When you just declare an Array there is no memory allocated, so if you try to use it you will get a Compile Time Error,
java: variable evenNumbers might not have been initialized
As Array's in Java are of type Object, you can initialize them using a new keyword followed by the data-type and size of the Array value between the braces,
int[] evenNumbers = new int[10];
double[] measurements = new double[10];
boolean[] validity = new boolean[10];
String[] userNames = new String[10];
If you do not provide the size you will get an compilation time error saying "array dimension missing"
public static void main(String[] args) {
String[] cha = new String[];
}
⛔️ java: array dimension missing
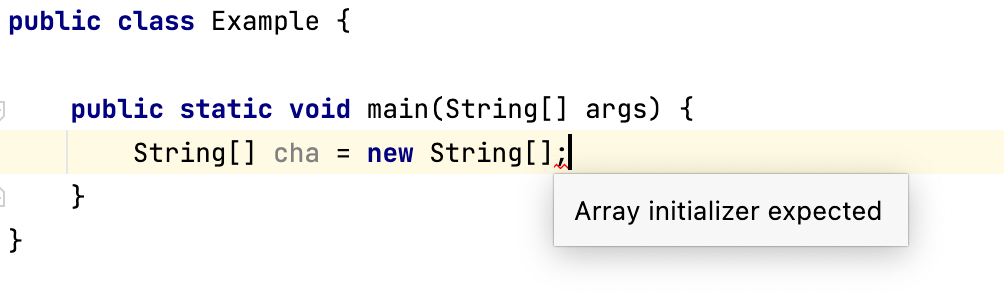
When you print the variables now, you will see that you get [ which implies an Array, followed by I -> Integer Array, D -> Double Type Array, Z -> Boolean Type Array, followed by @ and the Object hash,
System.out.println(evenNumbers);
System.out.println(measurements);
System.out.println(validity);
System.out.println(userNames);
[I@6bdf28bb
[D@6b71769e
[Z@2752f6e2
[Ljava.lang.String;@e580929
When you initialize an Array the elements of the array are initialized to the default value by the JVM,
- integer Array elements default value: 0
- double Array elements default value: 0.0
- boolean Array elements default value:
- char Array elements default value: NUL
- String/User Defined Array elements default value: null
int[] myArray = {10,20,30,40,50}
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
- Convert Hex to ASCII in Java with Example - Java
- AWS CLI Change Default Output Format - AWS
- W3 HTML validator warning Unable to Determine Parse Mode - Html
- Share image and text Twitter using your Android Application Programatically - Twitter
- How to Apply Themes to Notepad++ - NotepadPlusPlus
- How to Make Android TextView Text Bold - Android
- How to remove unwanted Java imports in IntelliJ (alternative of eclipse Ctrl + Shift + O) - Eclipse
- How to enable line numbers in IntelliJ - HowTos