If you want to convert a HEX value into an ASCII Character in Java, you can make use of the parseInt() function from Integer class with radix as 16 (base 16), then we cast the obtained integer from parsing the hexadecimal string and into a character type.
Example:
String hexValue = "4E"; //
System.out.println("HEX: " + hexValue);
char asciiChar = (char) Integer.parseInt(hexValue, 16);
System.out.println("ASCII: " + asciiChar);
Example: ASCII and HEX Table using Java
public class AsciiTableWithHex {
public static void main(String[] args) {
// Table header
System.out.println("+-------+-------+");
System.out.println("| Hex | ASCII |");
System.out.println("+-------+-------+");
for (int i = 0; i <= 127; i++) {
String hexValue = String.format("%02X", i);
char asciiChar = (char) i;
System.out.printf("| 0x%s | %c |\n", hexValue, asciiChar);
}
// Table footer
System.out.println("+-------+-------+");
}
}
Output:
-------+-------+
| Hex | ASCII |
+-------+-------+
| 0x21 | ! |
| 0x22 | " |
| 0x23 | # |
| 0x24 | $ |
| 0x25 | % |
| 0x26 | & |
| 0x27 | ' |
| 0x28 | ( |
| 0x29 | ) |
| 0x2A | * |
| 0x2B | + |
| 0x2C | , |
| 0x2D | - |
| 0x2E | . |
| 0x2F | / |
| 0x30 | 0 |
| 0x31 | 1 |
| 0x32 | 2 |
| 0x33 | 3 |
| 0x34 | 4 |
| 0x35 | 5 |
| 0x36 | 6 |
| 0x37 | 7 |
| 0x38 | 8 |
| 0x39 | 9 |
| 0x3A | : |
| 0x3B | ; |
| 0x3C | < |
| 0x3D | = |
| 0x3E | > |
| 0x3F | ? |
| 0x40 | @ |
| 0x41 | A |
| 0x42 | B |
| 0x43 | C |
| 0x44 | D |
| 0x45 | E |
| 0x46 | F |
| 0x47 | G |
| 0x48 | H |
| 0x49 | I |
| 0x4A | J |
| 0x4B | K |
| 0x4C | L |
| 0x4D | M |
| 0x4E | N |
| 0x4F | O |
| 0x50 | P |
| 0x51 | Q |
| 0x52 | R |
| 0x53 | S |
| 0x54 | T |
| 0x55 | U |
| 0x56 | V |
| 0x57 | W |
| 0x58 | X |
| 0x59 | Y |
| 0x5A | Z |
| 0x5B | [ |
| 0x5C | \ |
| 0x5D | ] |
| 0x5E | ^ |
| 0x5F | _ |
| 0x60 | ` |
| 0x61 | a |
| 0x62 | b |
| 0x63 | c |
| 0x64 | d |
| 0x65 | e |
| 0x66 | f |
| 0x67 | g |
| 0x68 | h |
| 0x69 | i |
| 0x6A | j |
| 0x6B | k |
| 0x6C | l |
| 0x6D | m |
| 0x6E | n |
| 0x6F | o |
| 0x70 | p |
| 0x71 | q |
| 0x72 | r |
| 0x73 | s |
| 0x74 | t |
| 0x75 | u |
| 0x76 | v |
| 0x77 | w |
| 0x78 | x |
| 0x79 | y |
| 0x7A | z |
| 0x7B | { |
| 0x7C | | |
| 0x7D | } |
| 0x7E | ~ |
| 0x7F | |
+-------+-------+
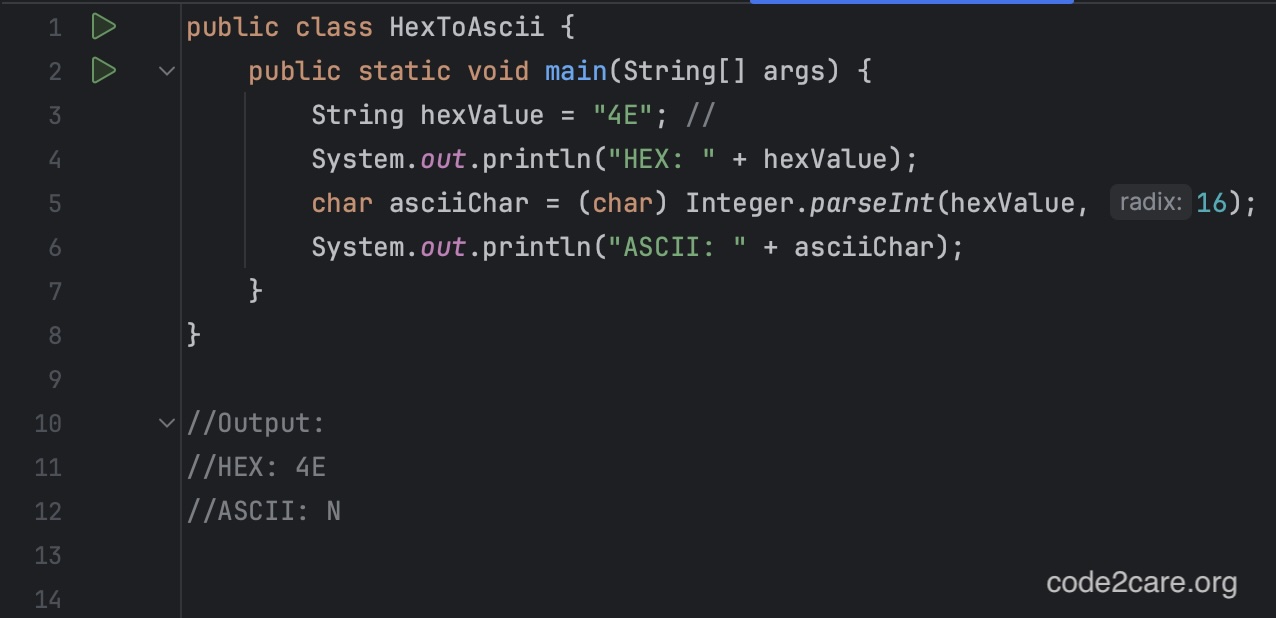
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- macOS Ventura XCode Command Line Tools Installation - MacOS
- [javaScript] Convert text case to lowercase - JavaScript
- Install Java Runtime Environment (Oracle or open JRE) on Ubuntu - Linux
- Setting $JAVA_HOME Environment Variable in macOS - MacOS
- Adding Jackson dependency to Java Gradle Project - Gradle
- auth_client_using_bad_version_title : Error Android Lint - Android
- How to take a screenshot of android emulator (AVD) screen - Android
- Fix: Windows 10/11 Update Install Error - 0x80070103 - Windows