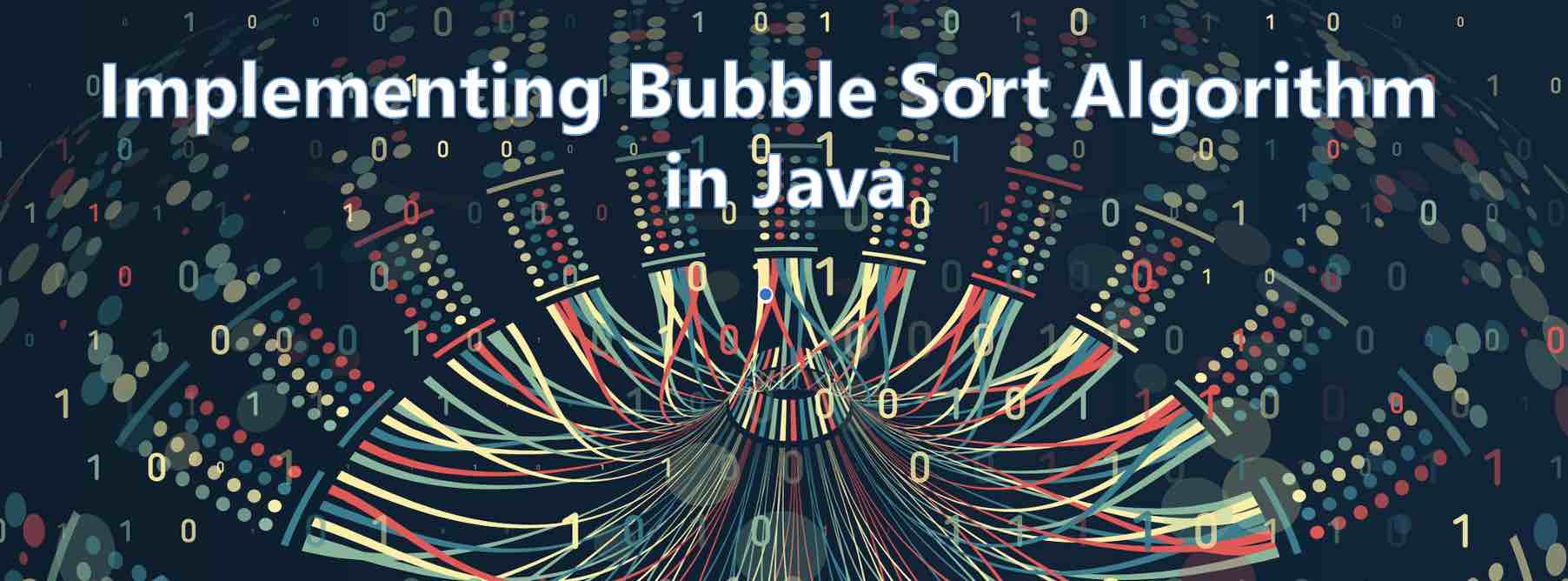
package org.code2care.java.sorting.algo;
import java.util.Arrays;
/**
* BubbleSort Example in Java
*
* Author: Code2care.org
* Date: 28 April 2023
* Version: v 1.0
*
*/
public class BubbleSortJavaExample {
public static void main(String[] args) {
//Unsorted Array
int[] unsortedArray = {4, 99, 2, 11, 34, 67, 54, 12, 45, 245, 234, 12, 200};
BubbleSortJavaExample bubbleSort = new BubbleSortJavaExample();
//Note: new array or Arrays.toString is not needed - added just for clarity
int[] sortedArray = bubbleSort.bubbleSortAlgorithm(unsortedArray);
System.out.println(Arrays.toString(sortedArray));
}
/**
*
* @param arrayOfNumbers: unsorted array as provided to sort
* @return: sorted array using bubble sort
*/
public int[] bubbleSortAlgorithm(int[] arrayOfNumbers) {
int arrayLength = arrayOfNumbers.length;
int tempVariable = 0;
for (int outerIndex = 0; outerIndex < arrayLength - 1; outerIndex++) {
for (int innerIndex = 0; innerIndex < arrayLength - outerIndex - 1; innerIndex++) {
int elementN = arrayOfNumbers[innerIndex];
int elementNPlus1 = arrayOfNumbers[innerIndex + 1];
if (elementN > elementNPlus1) {
tempVariable = arrayOfNumbers[innerIndex];
arrayOfNumbers[innerIndex] = arrayOfNumbers[innerIndex + 1];
arrayOfNumbers[innerIndex + 1] = tempVariable;
}
}
}
return arrayOfNumbers;
}
}
Output:
Complexity | Notation |
---|---|
Time complexity (worst-case) | O(n^2) |
Time complexity (average-case) | O(n^2) |
Time complexity (best-case) | O(n) |
Space complexity | O(1) |
Facing issues? Have Questions? Post them here! I am happy to answer!
Author Info:
Rakesh (He/Him) has over 14+ years of experience in Web and Application development. He is the author of insightful How-To articles for Code2care.
Follow him on: X
You can also reach out to him via e-mail: rakesh@code2care.org
More Posts related to Java,
- Get the current timestamp in Java
- Java Stream with Multiple Filters Example
- Java SE JDBC with Prepared Statement Parameterized Select Example
- Fix: UnsupportedClassVersionError: Unsupported major.minor version 63.0
- [Fix] Java Exception with Lambda - Cannot invoke because object is null
- 7 deadly java.lang.OutOfMemoryError in Java Programming
- How to Calculate the SHA Hash Value of a File in Java
- Java JDBC Connection with Database using SSL (https) URL
- How to Add/Subtract Days to the Current Date in Java
- Create Nested Directories using Java Code
- Spring Boot: JDBCTemplate BatchUpdate Update Query Example
- What is CA FE BA BE 00 00 00 3D in Java Class Bytecode
- Save Java Object as JSON file using Jackson Library
- Adding Custom ASCII Text Banner in Spring Boot Application
- [Fix] Java: Type argument cannot be of primitive type generics
- List of New Features in Java 11 (JEPs)
- Java: How to Add two Maps with example
- Java JDBC Transition Management using PreparedStatement Examples
- Understanding and Handling NullPointerException in Java: Tips and Tricks for Effective Debugging
- Steps of working with Stored Procedures using JDBCTemplate Spring Boot
- Java 8 java.util.Function and BiFunction Examples
- The Motivation Behind Generics in Java Programming
- Get Current Local Date and Time using Java 8 DateTime API
- Java: Convert Char to ASCII
- Deep Dive: Why avoid java.util.Date and Calendar Classes
More Posts:
- 33: Python Program to find the current time in India (IST) - Python-Programs
- How to connect Relational and NoSQL databases with Spring Boot - HowTos
- Check If Python pip modules are outdated and New Version is Available - PIP
- Remove Now Playing icon from macOS Big Sur Menu Bar - MacOS
- Android Parsing Data for android-L failed Unsupported major.minor version 51.0 Error - Android
- How to Rename a file using Mac Terminal - MacOS
- Fix SharePoint Error - Unable to Display Named Item - SharePoint
- Read a file using Java 8 Stream - Java