Let's see how we can create a simple Android AlertDialog with a message and 3 buttons, i.e. Yes, No and Cancel.
MainActivity.javaimport android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.support.v7.widget.Toolbar;
import android.util.Log;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends ActionBarActivity {
private Toolbar toolbar;
private Button myButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setTitle("AlertDialog with No Buttons");
builder.setMessage("Hello, you can hide this message by just tapping outside the dialog box!");
//Yes Button
builder.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(getApplicationContext(),"Yes button Clicked",Toast.LENGTH_LONG).show();
Log.i("Code2care ", "Yes button Clicked!");
}
});
//No Button
builder.setNegativeButton("No", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(getApplicationContext(),"No button Clicked",Toast.LENGTH_LONG).show();
Log.i("Code2care ","No button Clicked!");
dialog.dismiss();
}
});
//Cancel Button
builder.setNeutralButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(getApplicationContext(),"Cancel button Clicked",Toast.LENGTH_LONG).show();
Log.i("Code2care ","Cancel button Clicked!");
dialog.dismiss();
}
});
AlertDialog alertDialog = builder.create();
alertDialog.show();
}
}
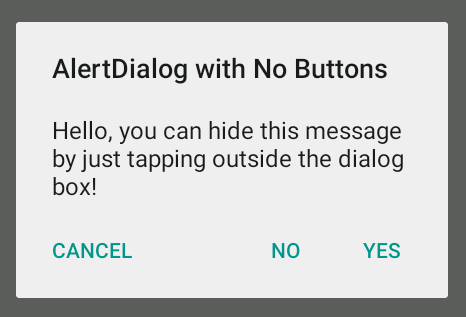
Alert Dialog with Yes No and Cancel Buttons
More Posts related to Android,
- Increase Android Emulator Timeout time
- Android : Remove ListView Separator/divider programmatically or using xml property
- Error : Invalid key hash.The key hash does not match any stored key hashes
- How to Change Android Toast Position?
- Android Alert Dialog with Checkboxes example
- Android : No Launcher activity found! Error
- Android Development: Spinners with Example
- Failed to sync Gradle project Error:failed to find target android-23
- INSTALL_FAILED_INSUFFICIENT_STORAGE Android Error
- Disable Fading Edges Scroll Effect Android Views
- How to create Toast messages in Android?
- Channel 50 SMSes received every few minutes Android Phones
- Android xml error Attribute is missing the Android namespace prefix [Solution]
- Create Custom Android AlertDialog
- How To Disable Landscape Mode in Android Application
- Android Development - How to switch between two Activities
- incorrect line ending: found carriage return (\r) without corresponding newline (\n)
- Generate Facebook Android SDK keyhash using java code
- Android Error Generating Final Archive - Debug Certificate Expired
- 21 Useful Android Emulator Short-cut Keyboard Keys
- Android RatingBar Example
- 11 Weeks of Android Online Sessions-15-Jun-to-28-Aug-2020
- Download interrupted: Unknown Host dl-ssl.google.com Error Android SDK Manager
- fill_parent vs match_parent vs wrap_content
- Android : Connection with adb was interrupted 0 attempts have been made to reconnect
More Posts:
- Python Program: Use NumPy to generate a random number between 0 and 1 - Python-Programs
- Fix: Eclipse Connection time out: github.com - Eclipse
- MacBook - Time Limit - You have reached your time limit, Ignore Limit - MacOS
- Create Custom Android AlertDialog - Android
- Python: Pandas Rename Columns with List Example - Python
- How to configure PDF iFilter for SharePoint - SharePoint
- Upload Pdf file using PHP Script - PHP
- Align html element at the center of page vertically and horizontally - Html